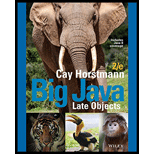
In which sequence are the lines of the Cubes.java program in Section 5.2 executed, starting with the first line of main?

Introduction:
- Define the class named “Cubes”.
- Define the main method.
- Declare and assign the required variables.
- Print the volume of the cube with length of the side as “2”.
- Print the volume of the cube with length of the side as “10”.
- Define the static method named “cubeVolume”.
- Declare and assign the required double variable.
- Return the value stored in the variable “volume”.
Explanation of Solution
//define a class
public class Cubes
{
//define the main method
public static void main(String[] args)
{
//declare the required variables
double result1=cubeVolume(2);
double result2=cubeVolume(10);
//print the output statements
System.out.println("A cube with side length 2 has
volume"
+result1);
System.out.println("A cube with side length 10
has volume"
+result2);
}
//define the static method
public static double cubeVolume(double sideLength)
{
//declare the required variables
double volume=sideLength*sideLength*sideLength;
//return statement
return volume;
}
}
A cube with side length 2 has volume 8
A cube with side length 10 has volume 1000
Want to see more full solutions like this?
Chapter 5 Solutions
Big Java Late Objects
Additional Engineering Textbook Solutions
Modern Database Management
Starting Out with Python (3rd Edition)
Starting Out with C++: Early Objects (9th Edition)
Artificial Intelligence: A Modern Approach
Database Concepts (8th Edition)
Java How To Program (Early Objects)
- Write a java program that can examine school children on multiplication. The kid has to select which multiplication table he wants to be tested in.- Display 10 random questions every time,- Display whether the answers are right or wrong,- Show the correct answers for wrong answers and- calculate the total score (out of 10) ((((It is just AN EXERCISE NOT AN EXAM)))) Thanksarrow_forwardHow to write a java program that return the nth Fibonacci number when passed the argument using a recursive method called Fibonacci and using a nonrecurive called FibonacciNonRecursive. Then write a driver to test the two versions of the two methods. Then compare the recursive method and a nonrecurive method for efficiency using comments. (The Fibonnacci sequence is the series of integers 0,1,1,2,3,5,8,13,21,34,55,89... Each element in the series is the sum of the preceding two elements. Here is recursive formula for calculating the nth number of the sequence fib(N)=N if N=0 or 1, fib(N)= Fib(N-2) +Fib(n-1), if N>1)arrow_forwardWrite a Java program that computes the product of odd numbers that are in the range [a, b] where a and b are given by the user.arrow_forward
- Write an application in java that prints the sum of cubes. Promptfor and read two integer values and print the sum of eachvalue raised to the third power.arrow_forwardWrite a java code to take the number of students, their names, their GPA, and their id, and show the name, the id, and the GPA of the from first to third.and sort their names alphabetically(WITHOUT USING JAVA METHODS), and show the students who their GPA is less than 2.arrow_forwardWrite a java program that will solve and display the problem below: Find three consecutive integers such that the sum of the first, twice the second, and three times the third is −76.arrow_forward
- Write a Java program to find and print the first 10 happy numbers.arrow_forwardWrite a program in java to compute the population of current year,if the population of current year is 10% more than that of last year. When the last year population is 140. Compute the current population.arrow_forwardHow would I solve this in Java? it has to: return a string for the day of the week, param offset -the offset from Thursday, and return the name of day of the week.arrow_forward
- Write a Java program Primes that finds all possible prime numbers in the range of (1, n], where 1 < n ≤ 1000. Solve the problem by implementing and using the helper method isPrime(number) which determines whether the given argument is prime or not. (the code should have the structure as in the picture)arrow_forwardWrite a java program to help the student to arrange his Diploma Marks of 12 subjects from high to low and Advanced diploma marks of 12 subjects low to high and also find the lowest mark in the advanced diploma.arrow_forwardWrite a Java program to find the largest number ‘L’ less than a given number ‘N’ which should not contain a given digit ‘D’. For example, If 145 is the given number and 4 is the given digit, then you should find the largest number less than 145 such that it should not contain 4 in it. In this case, 139 will be the answer.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
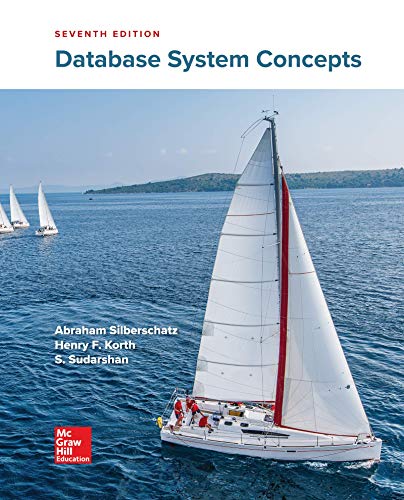
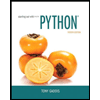
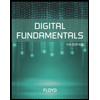
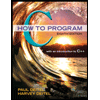
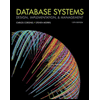
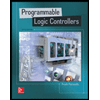