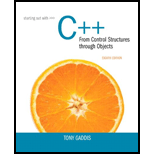
Concept explainers
Match the conditional expression with the if /else statement that performs the same operation.
A) q = x < y ? a + b : x * 2;
B) q = x < y ? x * 2 : a + b;
C) x < y ? q = 0 : q = 1;
_______if (x < y)
q = 0;
else
q = 1;
_______ if (x < y)
q = a + b;
else
q = x * 2;
________ if (x < y)
q = x * 2;
else
q = a + b;

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
SURVEY OF OPERATING SYSTEMS
Database Concepts (8th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Modern Database Management
Java: An Introduction to Problem Solving and Programming (8th Edition)
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, 1. Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key 2. Is there a direct relationship that exists between Store and Customer entities? AnswerYes/No?arrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer thequestions below, 1. Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M 2. Which one of the 4 Entities mention in the diagram can have a recursive relationship? 3. If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)? NO AI use pencil and paperarrow_forwardSTEP 1: The skeleton Let's start by creating a skeleton for some of the classes you will need. • Write a class called Tile. You can think of a tile as a square on the board on which the game will be played. We will come back to this class later. For the moment you can leave it empty while you work on creating classes that represents characters in the game. • Write an abstract class Fighter which has the following private fields: - A Tile field named position, representing the fighter's position in the game. A double field named health, representing the fighter's health points (HP). An int field named weaponType, representing the type of weapon the fighter is using. This value is used to rank different weapon types: higher values indicate higher weapon ranks. -An int field named attackDamage, representing the fighter's attack power. The class must also have the following public methods: 3 A constructor that takes as input a Tile indicating the position of the fighter, a double…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
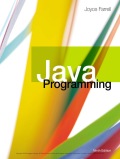
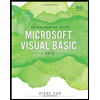
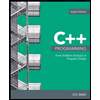
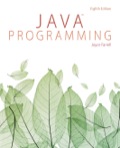
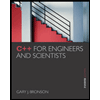