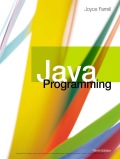
EBK JAVA PROGRAMMING
9th Edition
ISBN: 9781337671385
Author: FARRELL
Publisher: CENGAGE LEARNING - CONSIGNMENT
expand_more
expand_more
format_list_bulleted
Question
Chapter 4, Problem 2GZ
Program Plan Intro
Die game
Program plan:
Filename: “Die.java”
- Define the “Die” class
- Declare the variable.
- Define the constructor
- Set the value
- Define the “mythrow” method
- Determine the random value
- Return the value
Filename: “FiveDice.java”
- Define the “FiveDice” class
- Define the main method.
- Create two objects for “Die” class
- Declare the variable.
- Iterate “i” until it reaches 5
- Allocate memory for both the objects for the “Die” class.
- Iterate “i” until it reaches 5
- Throw the die for computer.
- Display the die value of the computer.
- Throw the die for player.
- Display the die value of the player.
- Define the main method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
This is the question:
Write an application that displays every perfect number from 1 through 1,000. A perfect number is one that equals the sum of all the numbers that divide evenly into it. For example, 6 is perfect because 1, 2, and 3 divide evenly into it, and their sum is 6; however, 12 is not a perfect number because 1, 2, 3, 4, and 6 divide evenly into it, and their sum is greater than 12.
This is the code given:
public class Perfect {
public static void main (String args[]) {
// Write your code here
}
public static boolean perfect(int n) {
// Write your code here
}
}
Using C# in Microsoft Visual Studio create an application that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Do not display the computer’s choice yet.)2. The user selects his or her choice of rock, paper, or scissors. To get this input you can use Button controls, or clickable PictureBox controls displaying some of the artwork that you will find in the student sample files.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors…
THIS MUST BE DONE IN CORAL:
Cracked Egg Game: There are a dozen eggs in a basket; some are hard boiled and some are raw. The object of this game is for the user to guess the number of hard-boiled eggs prior to playing the game. The computer then simulates cracking all 12 eggs, using a random number 0 or 1 to simulate raw or hard boiled. The number 0 should represent raw eggs and the number 1 should represent hard boiled. The computer must keep track of the number of hard-boiled eggs. At the conclusion of cracking all 12 eggs, the actual number of hard boiled is compared to the user’s guess, and whether the user won or lost is given as output.
The goal of this milestone is to use Coral to program one of the loops you will need for your finalized program in Project Two. This will help you define the logic, flow, and sequence of the game.
For this work, you will write code in Coral for your selected programming game by accessing the activity in the zyBook. Note that links for each are…
Chapter 4 Solutions
EBK JAVA PROGRAMMING
Ch. 4 - Prob. 1RQCh. 4 - Prob. 2RQCh. 4 - Prob. 3RQCh. 4 - Prob. 4RQCh. 4 - Prob. 5RQCh. 4 - Prob. 6RQCh. 4 - Prob. 7RQCh. 4 - Prob. 8RQCh. 4 - Prob. 9RQCh. 4 - Prob. 10RQ
Ch. 4 - Prob. 11RQCh. 4 - Prob. 12RQCh. 4 - Prob. 13RQCh. 4 - Prob. 14RQCh. 4 - Prob. 15RQCh. 4 - Prob. 16RQCh. 4 - Prob. 17RQCh. 4 - Prob. 18RQCh. 4 - Prob. 19RQCh. 4 - Prob. 20RQCh. 4 - Prob. 1PECh. 4 - Prob. 2PECh. 4 - Prob. 3PECh. 4 - Prob. 4PECh. 4 - Prob. 5PECh. 4 - Prob. 6PECh. 4 - Prob. 7PECh. 4 - Prob. 8PECh. 4 - Prob. 9PECh. 4 - Prob. 10PECh. 4 - Prob. 11PECh. 4 - Prob. 1GZCh. 4 - Prob. 2GZ
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Nim is a two-player game played with several piles of stones. You can use as many piles and as many stones in each pile as you want, but in order to better understand the game, we'll start off with just a few small piles of stones (see figure 1 below). Pile 1 Pile 1 Pile 2 The two players take turns removing stones from the game. On each turn, the player removing stones can only take stones from one pile, but they can remove as many stones from that pile as they want (please note, a player must remove atleast 1 stone from a pile during his/her turn). If they want, they can even remove the entire pile from the game! The winner is the player who removes the final stone (avoid taking the last stone - see figure 2 below). Pile 2 Pile 3 Pile 3 Let's say its Max (player 1) turn to play. Then Max can win by simply removing a stone from Pile 2 or Pile 3 Draw a game tree (upto depth level 2) for the given version of the Nim game. Please consider figure 1 as your initial game configuration/state…arrow_forwardDesign the worst calculator ever: an application that accepts two numbers from the user and, when a button is pushed, performs a single operation and displays the results. You may choose: Multiplication, Division, Subtraction, square root, or raising the first number to the exponent of the second number. Don't use addition as we already did that in class. The result must be displayed to the user only when an appropriately labeled button is pushed.arrow_forwardCreate an application that lets the user play the game of Rock, Paper, Scissors against the computer. The program should work as follows:1. When the program begins, a random number in the range of 1 through 3 is generated. If the number is 1, then the computer has chosen rock. If the number is2, then the computer has chosen paper. If the number is 3, then the computer has chosen scissors. (Do not display the computer’s choice yet.)2. The user selects his or her choice of rock, paper, or scissors. To get this input you can use Button controls, or clickable PictureBox controls displaying some of the artwork that you will find in the student sample files.3. The computer’s choice is displayed.4. A winner is selected according to the following rules:• If one player chooses rock and the other player chooses scissors, then rock wins. (Rock smashes scissors.)• If one player chooses scissors and the other player chooses paper, then scissors wins. (Scissors cuts paper.)• If one player chooses…arrow_forward
- Travel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. Accept the ticket number from the agent and verify whether it is a valid number. Test the application with the following ticket numbers: . 123454; the comparison should evaluate to true . 147103; the comparison should evaluate to true . 154123; the comparison should evaluate to false Save the program as TicketNumber.java.arrow_forwardGame Description: Pig is a game that has two players (in our case one human and one computer) that alternate turns. Each player’s goal is to get 100 points rolled on a normal six-sided die first. Each turn consists of the rolling the die repeatedly until you get a 1 or decide to stop. As long as you roll a 2-6, you will add this amount to your total for that turn. But if you roll a 1 during your turn, your turn ends and you receive zero points for that entire turn (erasing all of the progress you made since you last agreed to stop). If you decide to stop rolling at any point in your turn, your points for that turn are then added to the overall score. The overall score is then safe from future rolls. The trick is knowing how long to push it before we should stop and save our gains. See end of this document for an example of a game of pig we should write coding as below In order to explore what is the best strategy for the computer player (i.e. at what score do we stop each computer…arrow_forwardJava Programming A bakery company provides a bonus of a new bakery product package for customers who buy at least 10 breads in the company anniversary award which will be celebrated in the next three months. To get the bonus, the customer is given the opportunity three times to guess a number determined by the application from numbers 1 to 20. For each guess number given by the customer, the application will respond as follows:• If the guessed number is smaller than the lucky number, you will see the words "Bigger Number"• If the guessed number is greater than the lucky number, you will see the words "Smaller Number"• If the guessed number is the same as a lucky number, it will match the words "Congratulations ... you found a LUCKY number"As part of the company's IT team, you serve to create a lucky number guessing game console.A. Determine the inputs and outputs of the design programb. Draw a Flowchart of the programc. Write Coding to make the applicationd. Give Screen shoot the…arrow_forward
- Create an Adder application which prompts a player for the answer to an addition problem. The Adder game creates a problem from two randomly selected integers between 0 and 20. Adderallows the player three tries to enter a correct answer. If the correct answer isentered on the first try, the player is awarded 5 points. If the correct ansewer is enterd on the second try, 3 points are awarded. The correct answer on the third try earns 1 point. If after three tries, the correct answer is still not entered the player receives no points and the correct answer is displayed. The game continues until 999 is entered as sn answer. At the end of the game, Adder displays the players score. Application output should look similar to the following 20+5=25 20+2=20 Wrong answer. Enter another answer: 22 3+6=5 Wrong answer. Enter another answer: 4 5+12=17 14+18=999 Your score is 13arrow_forwardIn java A Carpet Company has asked you to write an application that calculates the price of carpeting for rectangular rooms in a building. To calculate the price, you multiply the area of the floor (width * length) by the price per square foot of carpet. For example, the area of floor that is 12 feet long and 10 feet wide is 120 square feet. To cover that floor with carpet that costs 300 per square foot would cost (12 * 10 * 300 = 36000.) First, you should create a class named RoomDimension that has two attributes: one for the length of the room and one for the width. Provide getters/setters, parameterized constructor and a toString method. The RoomDimension class should also have a member function that returns the area of the room. Once you have written the class, use in a test application that asks the user to enter the number of rooms in building create array of that size, then ask user to enter dimensions for each room, also ask user for price per square foot of the desired…arrow_forwardGreetings. The programming language to be used is: JAVA Please write a GUI that implements a game, where the user has to click the buttons in the order of their numbering. * The 9 buttons are arranged in a grid of 3 by 3. Each one has a different number from 1 to 9. * If the user selects the correct button, it should turn green and become disabled. The score should beincremented by 1. * If the user selects the incorrect button, it should turn red and all the buttons should become disabled. * If the user selects the “Reset” button, all buttons should be white and become enabled, their random numbers should be reassigned and the score set to 0. Thank you.arrow_forward
- The Westfield Carpet Company has asked you to write an application that calculates theprice of carpeting for rectangular rooms. To calculate the price, you multiply the area of thefloor (width times length) by the price per square foot of carpet. For example, the area offloor that is 12 feet long and 10 feet wide is 120 square feet. To cover that floor with carpetthat costs $8 per square foot would cost $960. (12 3 10 3 8 5 960.)First, you should create a class named RoomDimension that has two fields: one for the lengthof the room and one for the width. The RoomDimension class should have a method thatreturns the area of the room. (The area of the room is the room’s length multiplied by theroom’s width.)Next you should create a RoomCarpet class that has a RoomDimension object as a field. Itshould also have a field for the cost of the carpet per square foot. The RoomCarpet classshould have a method that returns the total cost of the carpet.Figure 8-21 is a UML diagram that shows…arrow_forwardJava Programming Travel Tickets Company sells tickets for airlines, tours, and other travel-related services. Because ticket agents frequently mistype long ticket numbers, Travel Tickets has asked you to write an application that indicates invalid ticket number entries. The class prompts a ticket agent to enter a six-digit ticket number. Ticket numbers are designed so that if you drop the last digit of the number, then divide the number by 7, the remainder of the division will be identical to the last dropped digit. This process is illustrated in the following example: Step 1. Enter the ticket number; for example, 123454. Step 2. Remove the last digit, leaving 12345. Step 3. Determine the remainder when the ticket number is divided by 7. In this case, 12345 divided by 7 leaves a remainder of 4. Step 4. Assign the Boolean value of the comparison between the remainder and the digit dropped from the ticket number. Step 5. Display the result—true or false—in a message box. Accept the…arrow_forwardWrite an application that allows a user to enter any number of student quiz scores, as integers, until the user enters 99. If the score entered is less than 0 or more than 10, display Score must be between 10 and 0 and do not use the score. After all the scores have been entered, display the number of valid scores entered, the highest score, the lowest score, and the arithmetic average.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
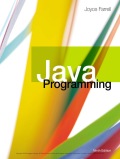
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
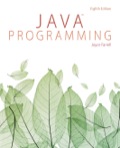
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
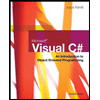
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
CPP Function Parameters | Returning Values from Functions | C++ Video Tutorial; Author: LearningLad;https://www.youtube.com/watch?v=WqukJuBnLQU;License: Standard YouTube License, CC-BY