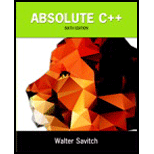
You would like to know how fast you can run in miles per hour. Your treadmill will tell you your speed in terms of a pace (minutes and seconds per mile, such as "5:30 mile") or in terms of kilometers per hour (kph).
Write an overloaded function called convertToMPH. The first definition should take as input two integers that represent the pace in minutes and seconds per mile and return the speed in mph as a double. The second definition should take as input one double that represents the speed in kph and return the speed in mph as a double. One mile is approximately 1.61 kilometers. Write a driver

Want to see the full answer?
Check out a sample textbook solution
Chapter 4 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
Mechanics of Materials (10th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Database Concepts (8th Edition)
Modern Database Management
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- Write the definition of a function that takes as input two decimal numbers and returns first number to the power of the second number plus second number to the power of the first number. (4)arrow_forwardWrite a function that computes the area of a triangle given the length of itsthree sides as parameters (see Programming Exercise 9 from Chapter 3).Use your function to augment triangle2 . py from this chapter so that italso displays the area of the triangle.arrow_forwardWrite a function that takes an integer input and check whether it can be expressed as the sum of two prime numbers. If it can be expressed as sum of two prime numbers then print those numbers. You also need to account for multiple combinations of prime number. Take a range as input from use and print all such number in that range. You can use as many helper function as needed. Sample: Enter range: 2 10 Range 2 to 10: 4: Can be written as 2 +2 5: Can be written as 3+ 2 6: Can be written as 3 +3 7: Can be written as 2 + 5 8: Can be written as 3 +5 9: Can be written as 2 + 7 10: Can be written as 3 + 7 10: Can be written as 5 + 5arrow_forward
- Write a function that adds two numbers. You should not use+ or any arithmeticoperators.arrow_forwardIf a year has the digit 7 OR the digit 4 in it, then it is called a “Beautiful year”. For example, 2017, 2014, and 2072 are all beautiful years. If the year has BOTH 4 and 7 in it, then it's an “Amazing year”. For example, the years 2047 and 2074 are amazing years. All other years are “Ugly years”. You need to write a function that takes a year as an argument and return it's type. Then, finally print the returned values in the function call. ================================================ Sample Function Call 1: function_name(2470) Sample Output 1: Amazing year ================================================ Sample Function Call 2: function_name(2170) Sample Output 2: Beautiful year ================================================ Sample Function Call 3: function_name(2021) Sample Output 3: Ugly yeararrow_forwardEngineers usually measure angles in degrees, yet most computer programs and many calculators require that the input to trigonometric functions be in radians. i. Write and test a function call DegToRadFunc that changes degrees to radians and Write another function RadToDegFunc that changes radians to degrees. Write another function called DegRadFunc that does the work of both DegToRadFunc and RadToDegFunc. Your functions should be able to accept both scalar an matrix input. ii. ... 11.arrow_forward
- Write a function that returns the average of the first N numbers,where N is a parameter.arrow_forwardWrite a function that computes the average of the digits in an integer. Use the following function header: double averageDigits(long n)arrow_forwardIn a particular jurisdiction, taxi fares consist of a base fare of $4.00, plus $0.25 for every 140 meters travelled. There are no "partial" travel charges, so the $0.25 is only applied if the passenger is taken to the 140-meter threshold. Some examples: if a passenger only travels 139 meters, the fare will be $4.00. If the passenger travels 279 meters, the fare will be $4.25. Write a function called fare() that takes the distance travelled (in miles) as its only parameter and returns the total fare as its only result. We are providing our test suite (test_fare.py) so you can test your code against a portion of the test framework we'll use to validate your solution.arrow_forward
- Write a function which can check whether a number is a prime number or not. Function prototype is: int prime(int a);Using this function, in the main function print all the prime numbers that are from 200 to 500. Also find out how many prime numbers are in that range.arrow_forwardWatch the video about Gauss's Addition in the Resources tab to see how you can use the formula for your function. Create a function that adds all the numbers together from 1 to n or, if two numbers are given: n to m. The input can be in any order. Examples gauss (100) → 5050 // From the video gauss (5001, 7000) gauss (1975, 165) → 1937770 12001000 // Also ^^arrow_forwardWrite a function that gets the area of a rectangle. The function must receive two parameters (decimal numbers) that represent the base and height of the rectangle and must return the calculated area (decimal number). Write a second function that obtains the volume of a rectangular prism with a rectangular base. The volume of such a prism is equal to the area of the base times the height of the figure. It uses calls to the previous function for this calculation. Volume=base*height*depth Call this last function in the main with user data. Execution example Give me the base: 21.3 Give me the height: 10 Give me the depth: 2.0 The volume of the prism is: 426.0arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
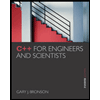
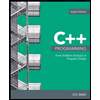