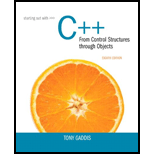
Concept explainers
Explanation of Solution
Given
//Include the header files
#include <iostream>
using namespace std;
//Main function
int main()
{
//Declare the variables
int integer1, integer2;
double result;
//Intialize the variables
integer1 = 19;
integer2 = 2;
/*Divide the integers and store the result in a variable*/
result = integer1 / integer2;
//Print the result
cout << result << endl;
/*Divide the integers by type-casting the first integer and store the result in a variable*/
result = static_cast<double>(integer1) / integer2;
//Print the result
cout << result << endl;
/*Divide the integers by type-casting the both integers and store the result in a variable*/
result = static_cast<double>(integer1 / integer2);
//Print the result
cout << result << endl;
//Return the statement
return 0;
}
Explanation:
- The first process in the program is to include the required header files...

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Starting Out with C++ from Control Structures to Objects (8th Edition)
- int x1 = 66; int y1 = 39; int d; _asm { } mov EAX, X1; mov EBX, y1; push EAX; push EBX; pop ECX mov d, ECX; What is d in decimal format?arrow_forwardC++ Functions provide a means to modularize applications Write a function called "Calculate" takes two double arguments returns a double result For example, the following is a function that takes a single "double" argument and returns a "double" result double squareArea(double side){ double lArea; lArea = side * side; return lArea;}arrow_forward#include <iostream>using namespace std; struct Person{ int weight ; int height;}; int main(){ Person fred; Person* ptr = &fred; fred.weight=190; fred.height=70; return 0;} What is a correct way to subtract 5 from fred's weight using the pointer variable 'ptr'?arrow_forward
- #include using namespace std; int main() int x=1,y=2; for (int i=0; i<3; i++) e{ x=x*y; 8{ } cout<arrow_forward#include<stdio.h> #include<stdarg.h> void fun1(int num, ...); void fun2(int num, ...); int main() { fun1(1, "Apple", "Boys", "Cats", "Dogs"); fun2(2, 12, 13, 14); return 0; } void fun1(int num, ...) { char *str; va_list ptr; va_start(ptr, num); str = va_arg(ptr, char *); printf("%s ", str); } void fun2(int num, ...) { va_list ptr; va_start(ptr, num); num = va_arg(ptr, int); printf("%d", num); }.arrow_forwardNonearrow_forward#include using namespace std3; int main() { int x-6; int y=103; int z=0; int q=1; Z+= X-- -ys q*=++z+y; cout<arrow_forwardStatic Variable: a variable whose lifetime is the lifetime of the program (static int x;) Dynamic Variable: It is a pointer to a variable (int *x;) Is this comparison true?arrow_forward#include using namespace std; || function declaration int max(int num1, int num2); int main () { // local variable declaration: int a = 100; int b = 200; int ret; I| calling a function to get max value. ret = max(a, b); cout num2) result = num1; else result = num2; return result; }arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_iosRecommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSONC How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education