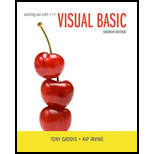
Concept explainers
The Upper West View Yacht club sponsors sailboat races every weekend for its fleet of SuperGee sailboats, and wants to determine which boat is the winner out of every four races. The scoring system is simple: a boat finishing in first position (first place) receives 1 point; a boat in second place receives 2 points, and so on. At the end of four races, the boats are ranked in ascending order by number of points, so the boats with the lowest points win.
Your task is to write an application that lets the user input the finishing position for each of three boats for four races. The program will then display the total points for each boat. Here are some specific requirements:
- Validate all text boxes to make sure the user enters integer values.
- Use a StatusStrip with a label to display an error message. When the user corrects an error and clicks the Calculate button again, clear any existing error message.
- Set TabIndex properties so that the focus will move between input fields in a logical manner.
- Do not allow the user to maximize or resize the form.
Figure 3-62 shows a sample of the program as the user is entering all valid values. In any single race, no place value should appear twice, although you currently do not have the
Figure 3-62 User input at runtime to the Sailboat Races program

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Starting Out With Visual Basic (7th Edition)
Additional Engineering Textbook Solutions
Starting Out with C++: Early Objects (9th Edition)
SURVEY OF OPERATING SYSTEMS
Mechanics of Materials (10th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Degarmo's Materials And Processes In Manufacturing
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
- whats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forwardWhat is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.arrow_forward
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
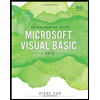
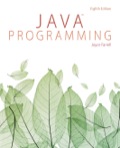
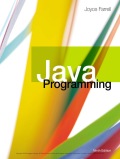
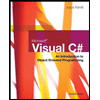