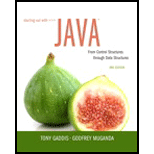
Concept explainers
Find the errors in the following code:
3. // Warning! This code contains ERRORS!
if (num2 == 0)
System.out.println(“Division by zero is not possible.”);
System.out.println(“Please run the
System.out.println(“and enter a number besides zero.”);
else
Quotient = num1 / num2;
System.out.print(“The quotient of ” + Num1);
System.out.print(“ divided by ” + Num2 + “ is ”);
System.out.println(Quotient);

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Additional Engineering Textbook Solutions
C Programming Language
Starting Out with Python (4th Edition)
Concepts of Programming Languages (11th Edition)
Problem Solving with C++ (9th Edition)
Artificial Intelligence: A Modern Approach
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Time CalculatorWrite a program that asks the user to enter a number of seconds.-There are 60 seconds in a minute. If the number of seconds entered by the user is greater than or equal to 60, the program should display the number of minutes and leftover seconds in that many seconds.-There are 3,600 seconds in an hour. If the number of seconds entered by the user is greater than or equal to 3,600, the program should display the number of hours, minutes, and leftover seconds in that many seconds.-There are 86,400 seconds in a day. If the number of seconds entered by the user is greater than or equal to 86,400, the program should display the number of days, hours, minutes, and leftover seconds in that many seconds.arrow_forward1. Write a program that lets the user guess whether a randomly generated integer would be even or odd. The program randomly generates an integer and divides it by 2. The integer is even if the remainder is 0, otherwise odd. The program prompts the user to enter a guess and reports whether the guess is correct or incorrect.arrow_forward3. Random Symbol Guessing Game Write a program that generates a random symbol and asks the user to guess what the symbol is. If the user's guess has a higher ASCII number than the random symbol, the program should display "Symbol ASCII too high, try again." If the user's guess has a lower ASCII number than the random symbol, the program should display "Symbol's ASCII too low, try again." The program should use a loop that repeats until the user correctly guesses the random symbol.arrow_forward
- Collatz Sequence: In 1937, Lothar Collatz proposed that no matter what number you begin with, the sequence eventually reaches 1. This is widely believed to be true but has never been formally proved. Write a program that inputs a number from the user, and then displays the Collatz Sequence starting from that number. Stop when you reach 1. If n is even, divide it by 2 to get n / 2. If n is odd, multiply it by 3 and add 1 to get 3n + 1. Repeat the process indefinitely. Find out and print the maximum number that you can get in these calculations. ' Part-3: Please enter a number: 2051 6154 3077 9232 4616 2308 1154 577 1732 866 433 1300 650 325 976 488 244 122 61 184 92 46 23 70 35 106 53 160 80 40 20 10 5 16 8 4 2 1 The maximum number you get: 9232arrow_forwardi. Find the loop invariant for the program code below. void main() { int y = 1, x = 0; while (x < 4) { y = y * z; x = x + 1; }arrow_forwardPython Programming onlyarrow_forward
- char letter1; char letter2; letter1 = 'y'; while (letter1 <= 'z') { letter2 = 'a'; while (letter2 <= 'c'){ System.out.print("" + letter1 + letter2 + " "); ++letter2; } ++letter1; } For this code why did we use "" before and after in this line? System.out.print("" + letter1 + letter2 + " "); ++letter2; What if we don't use the blank quotation would the output be same? because I don't see the reason for putting those quotation mark.arrow_forwardBox Office: A movie theater only keeps a percentage (20%) of the revenue earned from ticket sales. The remainder goes to the movie distributor. Write a program that calculates a theater’s gross and net box office profit for a night. The program should ask for the name of the movie, and how many adult and child tickets were sold. (The price of an adult ticket is $10.00 and a child’s ticket is $6.00.) Input: the name of the movie, number of adult tickets sold, number of child tickets sold Output: as specified below Object: cin, cout, getline, format output Note: 1. Movie names contain spaces 2, the report is aligned. 3. The decimal number is displayed with 2 decimal places.arrow_forward1. Kilometer Converter Write a program that asks the user to enter a distance in kilometers, then converts distance to miles. The conversion formula is as follows: Miles = Kilometers X 0.6214 %3Darrow_forward
- Question: Write program that asks the user to enter car information for 3 cars. The user will read the car plate number, driven mileage and number of months since last service. The program should display the message ‘Car is due for service’ if one of the following is true: The car drove more than 5000 KM The program should also count and display the number of cars due for service. Input/Output example: Enter car plate number: 600600 Enter the mileage and number of months since last service: 5230 Car is due for service Enter car plate number: 600666 Enter the mileage and number of months since last service: 5002 Car is due for service Enter car plate number: 600222 Enter the mileage and number of months since last service: 1500 The number of cars due is: 2arrow_forwardPlease add commentsarrow_forwardC Language - Write a program that takes in three integers and outputs the largest value. If the input integers are the same, output the integers' value.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
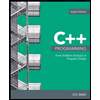