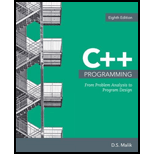
Consider the following incomplete C++
#include <iostream>
int main()
{
…
}
Write a statement that includes the header files fstream, string, and iomanip in this program.
Write statements that declare inFile to be an ifstream variable and outFile to be an ofstream variable.
The program will read data from the file inData.txt and write output to the file outData.txt. Write statements to open both of these files, associate inFile with inData.txt, and associate outFile with outData.txt.
Suppose that the file inData.txt contains the following data:
Giselle Robinson Accounting
5600 5 30
450 9
75 1.5
The first line contains a person's first name, last name, and the department the person works in. In the second line, the first number represents the monthly gross salary, the bonus (as a percent), and the taxes (as a percent). The third line contains the distance traveled and the traveling time. The fourth line contains the number of coffee cups sold and the cost of each coffee cup. Write statements so that after the program executes, the contents of the file outData.txt are as shown below. If necessary, declare additional variables. Your statements should be general enough so that if the content of the input file changes and the program is run again (without editing and recompiling), it outputs the appropriate results.
Name: Giselle Robinson, Department: Accounting
Monthly Gross Salary: $5600.00, Monthly Bonus: 5.00, Taxes: 30.000
Paycheck: $4116.00
Distance Traveled 450.00 miles, Traveling Time: 9.00 hours
Average Speed: 50.00 miles per hour
Number of coffee Cups Sold: 75, Cost: $1.50 per cup
Sales Amount = $112.50
Write statements that close the input and output files.
Write a C++ program that tests the statements in parts a through e.

Trending nowThis is a popular solution!

Chapter 3 Solutions
C++ Programming: From Problem Analysis to Program Design
- in c++ input file is .txt Write a program that asks for the name of an input file. Then, read all the numbers in the file, and display the following information to the screen: count of numbers in the file sum of all numbers in the file average of all numbers in the file (to 2 decimal places) count of numbers in each range (100-199, 200-299, 300-399, etc.) The program should: display a hello message ask the user for an input file display the name of the input file validate the file opens correctly display statistical information as shown above display a goodbye messagearrow_forwardInstructions Consider the following incomplete C++ program: #include <iostream> int main(){ ...} Write statements that include the header files fstream, string, and iomanip in this program. Write statements that declare inFile to be an ifstream variable and outFile to be an ofstream variable. The program will read data from the file inData.txt and write output to the file outData.txt. Write statements to: Open both of these files Associate inFile with inData.txt Associate outFile with outData.txt Suppose that the file inData.txt, data set 1, contains the following data: Giselle Robinson Accounting 5600 5 30 450 9 75 1.5 The first line contains a person’s first name, last name, and the department the person works in. In the second line, the first number represents the monthly gross salary, the bonus (as a percent), and the taxes (as a percent). The third line contains the distance traveled and the traveling time. The fourth line contains the number of coffee cups sold…arrow_forwardIn c++ write a code that tells a user to enter a filename and read that text file line by line.arrow_forward
- flowchrt for the code below //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days absent.The function takes two arguments:the number of…arrow_forwardWrite a C++ program which takes 5 students’ information (records) as inputs from the user and save them on a file. Your program should have two options: writing and searching. When a user selects writing option, you are required to take three inputs from the user: student name (string type), student age (int type). After reading these data-items write to the file named “student.txt”. Please note: write data using append mode otherwise previous information will be lost.Your program should have a search function. The search function should provide three options for searching: by name, by age, or by registration number. After selection of the search option by the user, please take input (name, age, or registration number) and search that student from the file and display its record on the screen.arrow_forwardThis is a C++ program, to be done in a .cpp file Problem Statement: Write rules for the user on how to play the game in a text file. Then, create a program that will read in the text file and display it out to the user. LCR Game Rules: The Dice● There are three dice rolled each turn. Each die has the letters L, C, and R on it along with dots on the remaining three sides. These dice determine where the player’s chips will go.○ For each L, the player must pass one chip to the player sitting to the left.○ For each R, the player must pass one chip to the player sitting to the right.○ For each C, the player must place one chip into the center pot and those chips are now out of play.○ Dots are neutral and require no action to be taken for that die.The Chips● Each player will start with three chips.● If a player only has one chip, he/she rolls only one die. If a player has two chips left, he/she rolls two dice. Once a player is out of chips, he/she is still in the game (as he/she may get…arrow_forward
- SUBJECT NAME IS C++: WAP to read 4 student objects and write them into a file, then print total number of student number in file. After that read an integer and print details of student stored at this number in the file using random access and file manipulatorsarrow_forwardC++ Create a program to open a text file and read in multiple lines of data. Use a regular expression to see if each line contains: Your First Name, Your Last Name, Your First Name or Last Name (anywhere in the text) Your First Name and Last Name (anywhere in the text) Your First Name followed by your Last Name (can contain text between first and last - such as middle name or middle initial).arrow_forwardC++: Create a standalone program that performs the tasks below. Start by solving task one and complete that task before starting task 2.Your program should read the files mentioned from the "current working directory".All files are in text format with "\ n" at the end of the line.If you wish, you can use pipes and redirects to solve the file reading and printing instead of std :: ifstream and std :: ofstream.In that case, comment on it in the codealong with examples of how the program is used. The file names.txt contains names and social security numbers in the following form. The file contains several people:First Name Last NameYYMMDDNNNNAddress bar Your task is to convert the input data into the form:Surname, First nameAddress bar The output should indicate whether the person is a man or a woman. For example, for a man it may say:Last name, First name [M]Address bar * YYMMDDNNNN: All those registered in Sweden are given a personal identity number as identification. A person who has…arrow_forward
- C++ Write a code fragment to: declare two file streams, infile and outfile Open them and connect them to the files file1.dat and file2.dat read an int from file1.dat and print it to file2.dat This should only be a half dozen lines of code.arrow_forwardDevelop a program in C++ that will create a filename NAMES.txt containing 20 string name. The program will determine if the given string name is in the file, if found the program will display “STRING FOUND” if not the program will display “STRING NOT FOUND”. *Using Iostream* With Screenshotarrow_forwardCreate the following programs in C++. Each program should be reading from a file (i.e., test1.txt, test2.txt, test3.txt) and then outputting the following to the screen. Note: No parameters in the main function Program 1: last line of the file Program 2: size of the longest line of the filearrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
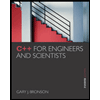