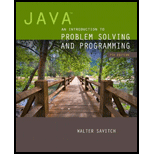
Write a fragment of code that will test whether an integer variable score contains a valid test score. Valid test scores are in the range 0 to 100.

Program plan:
- • Include necessary header files.
- • Define the class “Fragments”.
- • Define the “main()” function.
- ○ Declare and initialize the necessary variables.
- ○ The “if” statement check the condition.
- ■ True, print the valid statement.
- ○ Otherwise, print the invalid statement.
Program to display the valid test score.
Explanation of Solution
Program:
//Import necessary header files
import java.util.*;
//Define the class
class Fragments
{
//Define the main() method
public static void main(String[] args)
{
//Initialize the variable
int score = 10;
//Check the condition
if( score >= 0 && score <= 100 )
{
//True, print the statement
System.out.println("The score " + score + " is valid.");
}
//Otherwise
else
{
//Print the statement
System.out.println("The score " + score + " is not valid.");
}
}
}
Output:
The score 10 is valid.
Want to see more full solutions like this?
Chapter 3 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Additional Engineering Textbook Solutions
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
Starting Out with C++: Early Objects
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out With Visual Basic (7th Edition)
Database Concepts (8th Edition)
- X609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forwardAssignment4B: Diamonds in the sky. In our earliest labs, we asked you to print a diamond pattern to the screen using predefined print statements. Now that we know how to use loops, we can make more dynamic and customizable patterns. For this assignment, we will prompt the user to enter a maximum width for the diamond. If they enter a number less than 3, we'll prompt them to choose a correct width. If they enter an even number (greater than 3), we will add 1 to it and let the user know the final diamond size. Then we will generate and print out the diamond using * and '' symbols. Hints: Each line of the "diamond" is made up of two parts – the asterisks in the center and the spaces to the left of it. The amount of "left space" decreases as we go towards the middle diamond, then increases afterwards as we go towards the bottom. Could we use multiple loops (or even nested loops) to model this behavior? Also, note that the number of asterisks increases (and later decreases) by two on each…arrow_forwardThe value of x at the end.int x = 1;x = x + 1;x++;arrow_forward
- ATM machines allow 4 or 6 digit PIN codes and PIN codes cannot contain anything but exactly 4 digits or exactly 6 digits. Your task is to create a function that takes a string and returns true if the PIN is valid and false if it's not. Examples validatePIN("1234") ➞ true validatePIN("12345") ➞ false validatePIN("a234") ➞ false validatePIN("") ➞ false Notes ● Some test cases contain special characters. ● Empty strings must return false.arrow_forwardPRG11: Write a program that will calculate and show bills of the Manila Electric Company. The rates vary depending on whether the useR is residential (R), commercial( C) , or industrial (I). Any other code should be treated as an error. The program should accept the subscriber ID, Subscriber Name, his total electrical consumption in a month, and the code of the consumer type. The rates are computed as follows: R: 50 plus .50 per kwh used C: 100 for the first 1000 kwh and 0.45 for each additional kwh I: 180 for the first 1000 kwh and 0.75 for each additional kwharrow_forwardAnalyze the following code: int x = 0;int y = ((x < 100) && (x > 0)) ? 1: -1; The code has syntax error. y becomes 1 after the code is executed. y becomes -1 after the code is executed. The code has run time error.arrow_forward
- What will be the value of x after the following code is executed? int x = 0; while (x < 50) { x += 20; } 50 20 40 60arrow_forwardThis assignment is to modify the rock-paper-scissors game f. As before, each of twoplayers enters P, R, or S. The program will announce the winner and the basis for determining thewinner: Paper covers rock, Rock breaks scissors, Scissors cut paper, or Draw, nobody wins.The players must be able to enter either upper-case or lower-case letters.There are two major changes. First, you will need to create an enumeration called choices thathas three values, ROCK, PAPER, and SCISSORS. You should then use the typedef keyword to createa type for this enumeration named Choice. When you read the player's choice in your getThrowfunction, you will need to return a Choice for that player rather than a char as before. This makesit so that the only part of the program that deals with character type variables is the getThrowfunction; the rest of your program should always use variables of the enumerated Choice type. Thismeans that your checkWinner function will take two Choices as parameters…arrow_forwardPrint "user_num1 is negative." if user_num1 is less than 0. End with newline. Assign user_num2 with 1 if user_num2 is greater than 12. Otherwise, print "user_num2 is less than or equal to 12.". End with newline. My code: user_num1 = int(input())user_num2 = int(input()) if user_num1 < 0: print("user_num1 is negative.") if user_num2 > 12: user_num2 == 1 else: print("user_num2 is less than or equal to 12.") print('user_num2 is', user_num2) When the input is 0 and 13 my output for user_num2 is 13 and it should be 1. I don't know what I'm doing wrong.arrow_forward
- you need to fill the blank code partarrow_forwardConsider this code: "int s = 20; int t = s++ + --s;". What are the values of s and t? A. s is 19 and t is 38 B. s is 20 and t is 38 C. s is 20 and t cannot be determined D. s is 19 and t is 39 E. s is 20 and t is 39arrow_forward3. int x =9 ;display the address of the variable x? Answer:arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
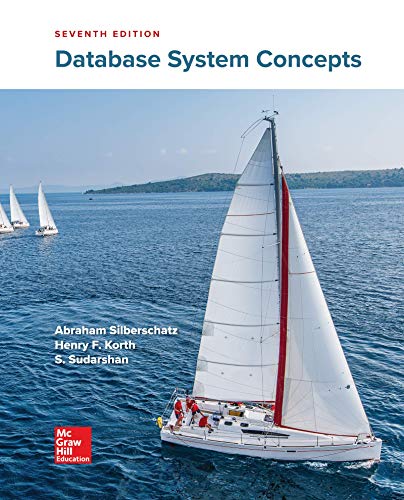
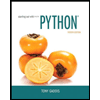
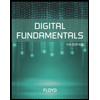
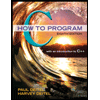
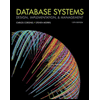
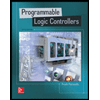