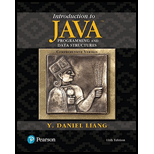
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 27, Problem 27.11PE
Program Plan Intro
Set operations in MyHashSet
Program Plan:
- Include a class name named “Exercise_11”.
- Import util package.
- Declare the main() method.
- Declare constructor.
- Create array for string names.
- Take user inputs and add into respective arrays.
- Create new Hashset.
- Add elements from set1 into set2.
- Remove all elements present in set1 from set 2.
- Retain elements present in set2 and set1.
- Check if set1 and set2 contains same elements.
- Close the main() method.
- Close the class.
- Include a static class “MyHashSet”.
- Define the default hash table size. Must be a power of 2.
- Define the maximum hash table size. 1 << 30 is same as 2^30.
- Declare current hash table capacity. Capacity is a power of 2.
- Define default load factor.
- Specify a load factor threshold used in the hash table.
- Declare a Hash table that is an array with each cell that is a linked list.
- Declare a constructor to Construct a set with the default capacity and load factor.
- Declare a constructor to Construct a set with the specific capacity and load factor.
- Declare an override method that Remove all elements from this set.
- Declare an override method that Return true if the element is in the set.
- Declare an override method that adds an element to the set.
- Create a linked list for the bucket if it is not created.
- Declare an override method that Remove the element from the set.
- Declare an override method that Return the number of elements in the set.
- Declare an override method to return the number of iterators.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Implement set operations in MyList)(JAVA)
Please use class name: Exercise_01
The implementations of the methods addAll, removeAll, retainAll, toArray(), and toArray(T[]) are omitted in the MyList interface. Implement these methods. Test your new MyList class using the code at https://liveexample.pearsoncmg.com/test/Exercise24_01.txt.
Sample Output:
Enter five strings for array name1 separated by space: TomGeorgePeterJeanJaneEnter five strings for array name2 separated by space: TomGeorgeMichaelMichelleDanielEnter two strings for array name3 separated by space: TomPeterlist1:[Tom, George, Peter, Jean, Jane]list2:[Tom, George, Michael, Michelle, Daniel]After addAll:[Tom, George, Peter, Jean, Jane, Tom, George, Michael, Michelle, Daniel]
list1:[Tom, George, Peter, Jean, Jane]list2:[Tom, George, Michael, Michelle, Daniel]After removeAll:[Peter, Jean, Jane]
list1:[Tom, George, Peter, Jean, Jane]list2:[Tom, George, Michael, Michelle, Daniel]After retainAll:[Tom, George]
list1:[Tom, George,…
Below is the specs and my main method. (ignore add and remove method for now)
Specs:
Part 1 - Tweet Bot
To start analyzing tweets, we first need to read in and manage the state of tweets. To do this, create a class called TweetBot. A TweetBot should have the following constructor and methods:
Constructor
public TweetBot(List<String> tweets)
Given a List of tweets, initialize a tweet list containing all tweets from the given collection. Note that you should not initialize your tweet list to the given List reference. You should create a new data structure and copy over all of the tweets from the given List, leaving the given List unmodified after the constructor is finished executing.
Throws an IllegalArgumentException if the size of the given collection is less than 1.
You may assume the given collection contains only non-empty and distinct strings.
Methods
public int numTweets()
Returns the number of tweets currently in the tweet list.
public void addTweet(String tweet)
Adds the…
(see image for code) Consider the following code. Replace the tags _??1_ and _??2_, respectively, by filling in the text fields below, such that running printGenericCollection(c) prints the contents of the Collection object c the console:
Chapter 27 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 27.2 - Prob. 27.2.1CPCh. 27.3 - Prob. 27.3.1CPCh. 27.3 - Prob. 27.3.2CPCh. 27.3 - Prob. 27.3.3CPCh. 27.3 - Prob. 27.3.4CPCh. 27.3 - Prob. 27.3.5CPCh. 27.3 - Prob. 27.3.6CPCh. 27.3 - If N is an integer power of the power of 2, is N /...Ch. 27.3 - Prob. 27.3.8CPCh. 27.3 - Prob. 27.3.9CP
Ch. 27.4 - Prob. 27.4.1CPCh. 27.4 - Prob. 27.4.2CPCh. 27.4 - Prob. 27.4.3CPCh. 27.4 - Prob. 27.4.4CPCh. 27.4 - Prob. 27.4.5CPCh. 27.4 - Prob. 27.4.6CPCh. 27.5 - Prob. 27.5.1CPCh. 27.6 - Prob. 27.6.1CPCh. 27.6 - Prob. 27.6.2CPCh. 27.6 - Prob. 27.6.3CPCh. 27.7 - Prob. 27.7.1CPCh. 27.7 - What are the integers resulted from 32 1, 32 2,...Ch. 27.7 - Prob. 27.7.3CPCh. 27.7 - Describe how the put(key, value) method is...Ch. 27.7 - Prob. 27.7.5CPCh. 27.7 - Show the output of the following code:...Ch. 27.7 - If x is a negative int value, will x (N 1) be...Ch. 27.8 - Prob. 27.8.1CPCh. 27.8 - Prob. 27.8.2CPCh. 27.8 - Can lines 100103 in Listing 27.4 be removed?Ch. 27.8 - Prob. 27.8.4CPCh. 27 - Prob. 27.1PECh. 27 - Prob. 27.2PECh. 27 - (Modify MyHashMap with duplicate keys) Modify...Ch. 27 - Prob. 27.6PECh. 27 - Prob. 27.7PECh. 27 - Prob. 27.8PECh. 27 - Prob. 27.10PECh. 27 - Prob. 27.11PECh. 27 - (setToList) Write the following method that...Ch. 27 - (The Date class) Design a class named Date that...Ch. 27 - (The Point class) Design a class named Point that...
Knowledge Booster
Similar questions
- (The ComparableSquare class) Define a class named ComparableSquare thatextends Square and implements Comparable. Implement the compareTo methodto compare the Squares on the basis of area. Write a test class to find the larger oftwo instances of ComparableSquareobjects.arrow_forwardComplete the Course class by implementing the printRoster() method, which outputs a list of all students enrolled in a course and also the total number of students in the course. Given classes: Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. (Hint: toString() returns a String representation of the Student object.) Ex: If the following students and their GPA values are added to a course: Henry Cabot with 3.5 GPABrenda Stern with 2.0 GPAJane Flynn with 3.9 GPALynda Robison with 3.2 GPA then the program output is: Henry Cabot (GPA: 3.5) Brenda Stern (GPA: 2.0) Jane Flynn (GPA: 3.9) Lynda Robison (GPA: 3.2) Students: 4 course.java import java.util.ArrayList; // Class representing a studentpublic class Course { private ArrayList<Student> roster; // Collection of Student objects public Course() {…arrow_forwardPLEASE USE PYTHON and don't make the code overly complicated and lengthy. Thanks. (Second time I am posting this) Create a KeyIndex class with the following properties : Fields: An array of integers. Note: You can maintain another instance variable if needed (but you can’t use more than one). Constructor: This constructor takes an array of integers a and populates array k with the element in a as indices into k. Note: make sure the build-up of your array k supports negative and non-distinct integers. Methods: This method searches for the value val within the array and returns true if found or false otherwise. sort () Description: This method will return the sorted form of the array that had been passed into the constructor. NOTE: Create a tester class or write tester statements to check whether the methods in your KeyIndex class work properlyarrow_forward
- please use the name, phonenumber and phonebookentry to build this. The three parallel arrays are gone — replaced with a single array of type PhonebookEntry. You should be reading in the entries using the read method of your PhonebookEntry class (which in turn uses the read methods of the Name and PhoneNumber classes). Use the equals methods of the Name and PhoneNumber classes in your lookup and reverseLookup methods. Use the toString methods to print out information. Make 100 the capacity of your Phonebook array Throw an exception (of class Exception) if the capacity of the Phonebook array is exceeded. Place a try/catch around your entire main and catch both FileNotFoundExceptions and Exceptions (remember, the order of appearance of the exception types in the catch blocks can make a difference). Do not use BufferedReader while(true) breaks The name of your application class should be Phonebook. Also, you should submit ALL your classes (i.e., Name, Strip off public from all your class…arrow_forwardComplete the Course class by implementing the findStudentHighestGpa() method, which returns the Student object with the highest GPA in the course. Assume that no two students have the same highest GPA. Given classes: Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three private fields: first name, last name, and GPA. (Hint: GetGPA() returns a student's GPA.) Ex: If the following students and their GPA values are added to a course: Henry Nguyen with 3.5 GPABrenda Stern with 2.0 GPALynda Robison with 3.2 GPASonya King with 3.9 GPA then the findHighestStudent() method returns a student and the program output is: Top student: Sonya King (GPA: 3.9)Code:Course.java: import java.util.ArrayList; //create class Student //create class Courseclass Courses{ private ArrayList<Student> roster; // collection of Student object // class constructor…arrow_forwardFor the first part of this lab, copy your working ArrayStringList code into the GenericArrayList class.(already in the code) Then, modify the class so that it can store any type someone asks for, instead of only Strings. You shouldn't have to change any of the actual logic in your class to accomplish this, only type declarations (i.e. the types of parameters, return types, etc.) Note: In doing so, you may end up needing to write something like this (where T is a generic type): T[] newData = new T[capacity]; ...and you will find this causes a compiler error. This is because Java dislikes creating new objects of a generic type. In order to get around this error, you can write the line like this instead: T[] new Data = (T[]) new Object[capacity] This creates an array of regular Objects which are then cast to the generic type. It works and it doesn't anger the Java compiler. How amazing! Once you're done, screenshot or save your code for checkin later. For the second part of the lab,…arrow_forward
- Use the right loop for the right assignment, using all the follow- ing loops: for, while without hasNext(), while with hasNext() and do-while. So I cannot use array. it has to be done in java.arrow_forwardPlease help solve this with java i posted it before and got rejected :( Modify the Show class such that it implements the Comparable interface of java.lang. Remember to add the concrete type Show between <> when you implement Comparable. Note the syntax error that occurs and understand why it occurs. 2. Implement (override) the compareTo method in the Show class such that it compares the showsaccording to their rates: returns 1 if the first show’s rate is greater than the second, -1if the first show’s rate is less than the second, and 0 if they are equal. (First show is “this”, second show is o) 3. Define an interface named Ratable, that has the method updateRate as shown in the UML diagram, in a file named Ratable.java. 4. Modify the Show class such that it implements the Ratable interface. Note the syntax error that occurs in the updateRate method and understand why it occurs. 5. Modify the updateRate method in the Show class such that it returns a double value that represents…arrow_forwardWrite the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below. Implement the following methods in arrayListType.h: isEmpty isFull listSize maxListSize clearList Copy constructor Implement the following method in unorderedArrayListType.h insertAt Also, write a program (in main.cpp) to test your function.arrow_forward
- (Sort ArrayList) Write the following method that sorts an ArrayList: public static <E extends Comparable<E>> void sort(ArrayList<E> list) Write a test program that prompts the user to enter 10 integers, invokes this method to sort the numbers, and displays the numbers in increasing order. Sample Run Enter 10 integers: 3 4 12 7 3 4 5 6 4 7 The sorted numbers are 3 3 4 4 4 5 6 7 7 12 Class Name: Exercise19_09arrow_forwardComplete the Course class by implementing the courseSize() method, which returns the total number of students in the course. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex. For the following students: Henry Bendel 3.6 Johnny Min 2.9 the output is: Course size: 2 public class LabProgram { public static void main (String [] args) { Course course = new Course(); // Example students for testing course.addStudent(new Student("Henry", "Bendel", 3.6)); course.addStudent(new Student("Johnny", "Min", 2.9)); System.out.println("Course size: " + course.courseSize()); } } public class Student { private String first; // first name private String last; // last name private…arrow_forwardUse NetBeans Note for all the above User-Defined Classes: • Provide appropriate validation code so the right values get populated in the instance variables. For example, the payrate should not be negative. Write a Java application (Client) program with a static method called generateEmployees( ) that returns a random list of 10 different types of Employee objects. You would use an ArrayList to store the employee objects that will be returned. Use a for loop to populate randomly different types of employee objects with some random data. You could possibly think of a range of values like 1 – 4. If random value is 1, create a HourlyEmployee object with some randomly generated data, if 2, a SalariedEmployee object with some random data and so on. I would leave it to your ingenuity to generate and populate these different Employee objects with other data like name etc. As these objects are generated, add them to your data structure (array or ArrayList that you are using). Finally, the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
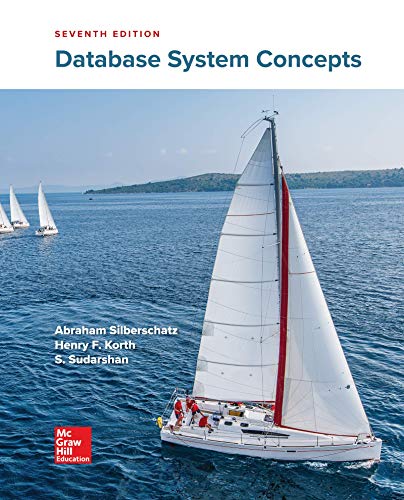
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
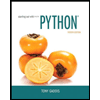
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
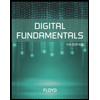
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
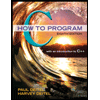
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
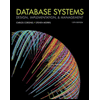
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
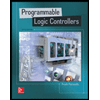
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education