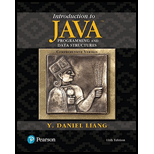
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 23, Problem 23.7PE
Program Plan Intro
Min-heap
Program Plan:
Filename: Sorting.java
- Import the required packages.
- Create a class “Sorting”:
- Define the main method
- Generate the heap and initialize the heap elements.
- Validate the size and the remove the elements from the heap.
- Define the main method
Filename: MinHeap.java
- Define the class “minHeap”
- Create a new array list.
- Create a method “MinHeap()”.
- Define a method “MinHeap()”.
- Loop that iterates to add all the elements into the heap.
- Define a method “add()”
- Add the objects into the list.
- Assign the current index value.
- Loop that validates the index value is greater than zero.
- Based on the requirements and condition defined swap is made on the list.
- Finally elements are added into the list
- Define the method “remove()”
- Validates the size of list.
- Initialize the remove object.
- Loop that iterates to validate the index value is greater the size of the list.
- Based on the requirements and condition defined swap is made on the list.
- Finally the required element is removed from the list.
- Define the method “getsize()”
- Used to return the size of the list.
- Define a method “MinHeap()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Project 4: Maze Solver (JAVA)
The purpose of this assignment is to assess your ability to:
Implement stack and queue abstract data types in JAVA
Utilize stack and queue structures in a computational problem.
For this question, implement a stack and a queue data structure. After testing the class, complete the depth-first search method (provided). The method should indicate whether or not a path was found, and, in the case that a path is found, output the sequence of coordinates from start to end.
The following code and related files are provided. Carefully ready the code and documentation before beginning.
A MazeCell class that models a cell in the maze. Each MazeCell object contains data for the row and column position of the cell, the next direction to check, and a bool variable that indicates whether or not the cell has already been visited.
A Source file that creates the maze and calls your depth-first search method.
An input file, maze.in, that may be used for testing.
You…
C++ CODE PLEASE
Using an array to represent the min-max heap structure , implement the following operations. (in c++ programming language)
1. buildHeap : Builds a min-max heap from a list of naturals read from standard input.
2. findMin and findMax : Returns the minimum (resp the maximum) element.
3. insertHeap : Inserts a new element into the min-max heap.
4. deleteMin and deleteMax : Deletes the minimum (resp the maximum) element.
NOTE: min-max should be in one heap structure only and not separate heap structure for min and max. Only one heap for both min and max. Root should be min level, the level below root should be max level,then level after that should again be min level and so on.
(IN JAVA) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:(i) insert, which takes an integer (a key) and inserts it in the binomial heap. Themethod returns true, if the key is successfully inserted and false otherwise. In thecase, if the array should be resized, use the method resizeArray (see below).(ii) getMin, which returns the minimal key in binomial heap. The implementation willbe also efficient…
Chapter 23 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 23.2 - Prob. 23.2.1CPCh. 23.2 - Prob. 23.2.2CPCh. 23.2 - Prob. 23.2.3CPCh. 23.3 - Prob. 23.3.1CPCh. 23.3 - Prob. 23.3.2CPCh. 23.3 - Prob. 23.3.3CPCh. 23.4 - Prob. 23.4.1CPCh. 23.4 - Prob. 23.4.2CPCh. 23.4 - What is wrong if lines 615 in Listing 23.6,...Ch. 23.5 - Prob. 23.5.1CP
Ch. 23.5 - Prob. 23.5.2CPCh. 23.5 - Prob. 23.5.3CPCh. 23.5 - Prob. 23.5.4CPCh. 23.6 - Prob. 23.6.1CPCh. 23.6 - Prob. 23.6.2CPCh. 23.6 - Prob. 23.6.3CPCh. 23.6 - Prob. 23.6.4CPCh. 23.6 - Prob. 23.6.5CPCh. 23.6 - Prob. 23.6.6CPCh. 23.6 - Prob. 23.6.7CPCh. 23.6 - Prob. 23.6.8CPCh. 23.6 - Prob. 23.6.9CPCh. 23.7 - Prob. 23.7.1CPCh. 23.7 - Prob. 23.7.2CPCh. 23.8 - Prob. 23.8.1CPCh. 23 - Prob. 23.1PECh. 23 - Prob. 23.2PECh. 23 - Prob. 23.3PECh. 23 - (Improve quick sort) The quick-sort algorithm...Ch. 23 - (Check order) Write the following overloaded...Ch. 23 - Prob. 23.7PECh. 23 - Prob. 23.8PECh. 23 - Prob. 23.10PECh. 23 - Prob. 23.11PECh. 23 - Prob. 23.12PECh. 23 - Prob. 23.13PECh. 23 - (Selection-sort animation) Write a program that...Ch. 23 - (Bubble-sort animation) Write a program that...Ch. 23 - (Radix-sort animation) Write a program that...Ch. 23 - (Merge animation) Write a program that animates...Ch. 23 - (Quicksort partition animation) Write a program...Ch. 23 - (Modify merge sort) Rewrite the mergeSort method...
Knowledge Booster
Similar questions
- (In java) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:-> delMin, which deletes the minimal key from the binomial heap. The method returnstrue, if the minimal key is successfully deleted and false otherwise (if the binomialheap is empty).-> resizeArray, which extends the array. The method is needed, for example, whenyou find out that you need an extra place in your array when inserting new…arrow_forward(In java) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:-> delMin, which deletes the minimal key from the binomial heap. The method returnstrue, if the minimal key is successfully deleted and false otherwise (if the binomialheap is empty).-> resizeArray, which extends the array. The method is needed, for example, whenyou find out that you need an extra place in your array when inserting new…arrow_forward(In java) In this task, a binomial heap should be implemented. A binomial heap is implemented byan array with its elements being binomial trees: on the first place there is the binomial treeB0 with one element, on the second place there is the binomial tree B1 with two elements,on the third place there is the binomial tree B2 with four elements,. . . Each binomial treeis implemented recursively using the class BinomialNode. In this class there is nothing toimplement. Methods in this class are needed for the implementation of the methods in theclass BinomialHeap. More precisely, in the class BinomialHeap you should implement thefollowing methods:(i) insert, which takes an integer (a key) and inserts it in the binomial heap. Themethod returns true, if the key is successfully inserted and false otherwise. In thecase, if the array should be resized, use the method resizeArray (see below).(ii) getMin, which returns the minimal key in binomial heap. The implementation willbe also efficient…arrow_forward
- Please use Python for this question: Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty, False otherwise [] – return the item at a given location, [0] is at…arrow_forwardPlease be thorough with explanation (a) Consider implementing a stack as a class that relies on a singly linked list, maintaining pointers to both the start and end of the singly linked list in the main object. You consider two possibilities. The first is to push (insert) to the beginning and pop (remove) from the beginning of the linked list. The second is to push to the end and pop from the end of the linked list. Is one of these possibilities better than the other? Briefly explain your answer.arrow_forwardQ2: Stack stores elements in an ordered list and allows insertions and deletions at one end.The elements in this stack are stored in an array. If the array is full, the bottom item is dropped from the stack. In practice, this would be equivalent to overwriting that entry in the array. And if top method is called then it should return the element that was entered recently Please do it in C++ and use stack class(header file), not premade functionarrow_forward
- 1 : 2 O Implementing Stack In this part, you will implement a growable stack using arrays in C++. You start with an array of size = 1. Every time the stack gets full, you copy the data to an array of double the previous size, and the new array now represents the stack. To do so, you will have to use a dynamic array (using pointers) and allocate memory using the new operator. • Write a C++ class MyStack in file MyStack.cpp that implements the above stack with follow- ing public methods. - void push(int item) : Pushes the item onto the top of the stack. - int pop() : Removes the element at the top of the stack and returns it. - int peek() : Returns the top element without removing from top of the stack. bool empty() : Returns true is the stack is empty and false otherwise. All other methods or variables in your implementation should be private.arrow_forwardHeap (C PROGRAM) Given a maxheap (keeps the largest value at the root), which has 4 functions push(h,v), v<-pop(h), new(h), and b<-isNotEmpty(h), where h is a heap, v is the value pushed/popped on the heap, new creates a new empty heap, isNotEmpty tests the heap, and b is a Boolean. What will the pseudocode below print? new(h); push(h, 10); push(h, 200); push(h, 0); push(h, 999); push(h, 5); while (isNotEmpty(h)) do {print(pop(h),” “);} print(“\n”);arrow_forwardGoal implementing stack functions, use stack to reverse input, search for palindrome strings and substrings [chapter 4] Deliverables تسليم ملفين 1- PDF file or MS-Word with the - Your name, AHU ID, class time - Output Explanation for any problems you faced and how did you solve it 2- Source code d Individuals [ Groups of { } members ] Any Work Style Grade Task 1: General guidelines Use the class notes to write a program for a stack #inlcude You need to : 1- Write the functions of push, pop, top , isEmpty , isFull 2- Show how the stack works (in the main() you need to call those functions you implemented ) using namespace std; int max=5; int stack(max]; int top=0; void push(int val) { // TODO : write your code here Int pop(){ } bool isEmpty(){ } bool isFull(){ Int top(){ Int main(){ Int x=0; push(3); push(4); x=top(); Return 0; wEarrow_forward
- Question #7. SORTED LIST NOT UNSORTED C++ The specifications for the Sorted List ADT state that the item to be deleted is in the list. • Rewrite the specification for Deleteltem so that the list is unchanged if the item to be deleted is not in the list. • Implement Deleteltem as specified in (a) using an array-based. Implement Deleteltem as specified in (a) using a linked implementation. • Rewrite the specification for Deleteltem so that all copies of the item to be deleted are removed if they exist. • Implement Deleteltem as specified in (d) using an array-based. • Implement Deleteltem as specified in (d) using a linked implementation.arrow_forward(Circular linked lists) This chapter defined and identified various operations on a circular linked list.a. Write the definitions of the class circularLinkedList and its member functions. (You may assume that the elements of the circular linked list are in ascending order.)b. Write a program to test various operations of the class defined in (a).arrow_forward. Mark the following as True and False Non-predictive parsers use stack and parsing table to parse the input. In FOLLOW set, $ sign is used to indicate the end of stack. Left recursion and left factoring are used to construct predictive parsers. Bottom-up parsers are also called LR parsers. In constant propagation and constant folding, values of variables are replaced with constant value. The input to the code generator is a parse tree. Intermediate code eliminates the need of a new compiler for every unique machine by keeping the synthesis part same for all the compilers.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
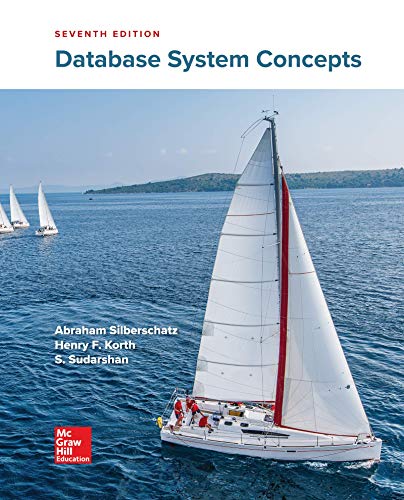
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
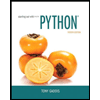
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
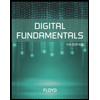
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
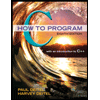
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
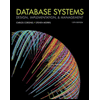
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
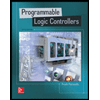
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education