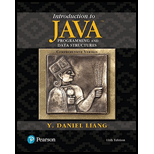
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21.7, Problem 21.7.1CP
Program Plan Intro
Program:
//import util package
import java.util.*;
//class definition
public class Example_1
{
//main method
public static void main(String[] args)
{
/* declare singleton set and add "Chicago" */
Set<String>set = Collections.singleton("Chicago");
//add Dallas into set
set.add("Dallas");
//print set
System.out.println("set is " + set);
}
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
df.Month.replace(({1:'January', 2:'February', 3:'March',4:'April', 5:'May', 6:'June', 7:'July', 8:'August',9:'September',10:'October',11:'November',12:'December'}), inplace=True)df
kindly explain what this code do in phyton
Not allowed to add variables in brackets next to originIndex
* @return index of the point that is closest to the origin, which is (0, 0) In * case of a tie, return the lowest index */ public int closestToOriginIndex() {
A feature of our Set ADT is that it is a collection that allows duplicate elements.
Chapter 21 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 21.2 - Prob. 21.2.1CPCh. 21.2 - Prob. 21.2.2CPCh. 21.2 - Prob. 21.2.3CPCh. 21.2 - Prob. 21.2.4CPCh. 21.2 - Prob. 21.2.5CPCh. 21.2 - Suppose set1 is a set that contains the strings...Ch. 21.2 - Prob. 21.2.7CPCh. 21.2 - Prob. 21.2.8CPCh. 21.2 - What will the output be if lines 67 in Listing...Ch. 21.2 - Prob. 21.2.10CP
Ch. 21.3 - Prob. 21.3.1CPCh. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores...Ch. 21.3 - Suppose you need to write a program that stores a...Ch. 21.3 - Prob. 21.3.5CPCh. 21.3 - Prob. 21.3.6CPCh. 21.4 - Prob. 21.4.1CPCh. 21.4 - Prob. 21.4.2CPCh. 21.5 - Prob. 21.5.1CPCh. 21.5 - Prob. 21.5.2CPCh. 21.5 - Prob. 21.5.3CPCh. 21.6 - Prob. 21.6.1CPCh. 21.6 - Prob. 21.6.2CPCh. 21.6 - Prob. 21.6.3CPCh. 21.6 - Prob. 21.6.4CPCh. 21.7 - Prob. 21.7.1CPCh. 21.7 - Prob. 21.7.2CPCh. 21 - Prob. 21.1PECh. 21 - (Display nonduplicate words in ascending order)...Ch. 21 - Prob. 21.3PECh. 21 - (Count consonants and vowels) Write a program that...Ch. 21 - Prob. 21.6PECh. 21 - (Revise Listing 21.9, CountOccurrenceOfWords.java)...Ch. 21 - Prob. 21.8PECh. 21 - Prob. 21.9PE
Knowledge Booster
Similar questions
- int* p: int a[3]{1, 2, 3}; p = a; What is the value of *(p+2)?arrow_forwardWhat is the set builder form for {7,8,9,10...}arrow_forwardFunction 1: draw_subregion Complete the implementation of draw_subregion. This function has the following parameters: my_turtle: A turtle object (which will do the drawing) polygon_points: A list of (x, y) points (i.e. a list of tuples) that defines the points of a polygon. This function should make the turtle draw the polygon given by the points. Make sure that you lift your pen before heading to the first point. You should also make sure you return to the very first point at the end (i.e. you will go to the first point in the list two times: once at the beginning, and once at the end). Language Pythonarrow_forward
- Doctor -signature:String -doctorID:int - medicine:Arraylist +Doctor(signature:String,doctorID:int) +PrescribeMedicine():void + salary () +checkRecords():void Medicine Pharmacist -medName:String -startTime:int -dosage :int -endTime:int -date_prescribed:int - medicine:Arraylist +Medicine(medName:String,-dosage :int,date_prescribed:int) +Pharmacist (startTime:int,endTime:int) +checkForConflict():double +confirm_prescription():String +getStartTime():int +getEndTime():int +setStartTime(time:int):void +setEndTime(time1:int).voidarrow_forward#include<iostream> #include<cstdlib> #include<string> #include<cstdio> using namespace std; const int TABLE_SIZE = 128; /* * HashEntry Class Declaration */ class HashEntry { public: int key; int value; HashEntry(int key, int value) { this->key = key; this->value = value; } }; /* * HashMap Class Declaration */ class HashMap { private: HashEntry **table; public: HashMap() { table = new HashEntry * [TABLE_SIZE]; for (int i = 0; i< TABLE_SIZE; i++) { table[i] = NULL; } } /* * Hash Function */ int HashFunc(int key) { return key % TABLE_SIZE; } /* * Insert Element at a key */ void Insert(int key, int value) { int hash = HashFunc(key); while (table[hash] != NULL && table[hash]->key != key)…arrow_forward#include<iostream> #include<cstdlib> #include<string> #include<cstdio> using namespace std; const int TABLE_SIZE = 128; /* * HashEntry Class Declaration */ class HashEntry { public: int key; int value; HashEntry(int key, int value) { this->key = key; this->value = value; } }; /* * HashMap Class Declaration */ class HashMap { private: HashEntry **table; public: HashMap() { table = new HashEntry * [TABLE_SIZE]; for (int i = 0; i< TABLE_SIZE; i++) { table[i] = NULL; } } /* * Hash Function */ int HashFunc(int key) { return key % TABLE_SIZE; } /* * Insert Element at a key */ void Insert(int key, int value) { int hash = HashFunc(key); while (table[hash] != NULL && table[hash]->key != key)…arrow_forward
- Write set {1, 5, 15, 25} in set-builder form. Select one: a. {x: either x=1 or x=5n, where n is a real number} b. {x: x=5n, where n is an integer} c. {x: either x=1 or x=5n, where n is a positive integer} d. Nonearrow_forwardWrite Set values of data members using default, parameterized and copy constructor in c++ Note: Dont Copy From Google And paste Code here Dont Send Code Screenshortarrow_forwardCell Phones Records In this part, you are required to write a program, using linked lists, that manipulates a set of records of cell phones and performs some operations on these records. I) The CellPhone class has the following attributes: a serialNum (long type), a brand (String type), a year (int type, which indicates manufacturing year) and a price (double type). It is assumed that brand name is always recorded as a single word (i.e. Motorola, SonyEricsson, Panasonic, etc.). It is also assumed that all cellular phones follow one system of assigning serial numbers, regardless of their different brands, so no two cell phones may have the same serial number. You are required to write the implementation of the CellPhone class. Beside the usual mutator and accessor methods (i.e. getPrice(), setYear()) the class must have the following: (a) Parameterized constructor that accepts four values and initializes serialNum, brand, year and price to these passed values; (b) Copy constructor,…arrow_forward
- this is my code and the question need to be continued. #include <iostream>using namespace std; class Course{private: string name; string *studentList; int size, capacity=10;public: Course(){ name = "TBD"; size = 0; studentList = new string[capacity]; }Course(string s){ name = s; size = 0; studentList = new string[capacity]; }void setName(string s){ name = s; }getter/accessor for variable name string getName(){ return name; }}; int main(){ Course a, b("Yankee"); cout << a.getName() << endl; a.setName("Mets"); cout << a.getName() << endl; cout << b.getName() << endl; return 0;} this is the Question:: Continue with Team class: a) Copy the previous program to a new file.b) Implement the addMember, removeMember and…arrow_forwardConsider the set A = {1, 2, 11, {121}, 22, 1212, (111, 212, 112}} Enter the value of Al. (Note: Enter a number).arrow_forwardPlease help me in C++. Here are a few requirements:only using void DoublyList::selecSuitor()don't forget to update the countdon't forget to delete the nodeThank you !!!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
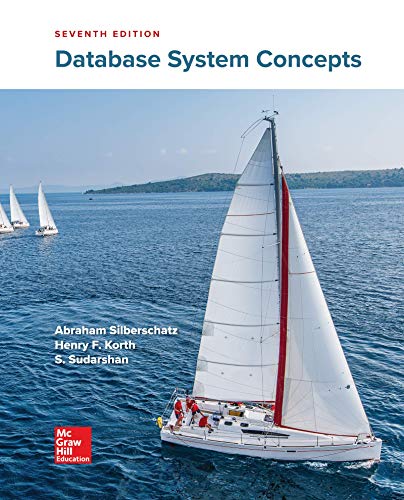
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
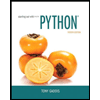
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
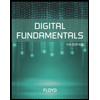
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
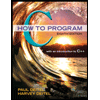
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
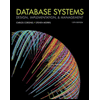
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
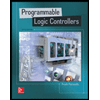
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education