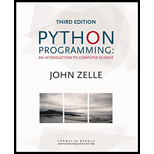
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Question
Chapter 2, Problem 3PE
Program Plan Intro
Computing average
Program Plan:
- Declare a main function. Inside the main function,
- Print the statement
- Get the three exam scores from the user.
- Calculate the average.
- Print the result.
- Call the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
kindly change the program following the procedure on the problem. please list the changes you have made on the program t..thank you
Implement the plus_one function as directed in the comment above the function. If the directions are unclear, run the code, and look at the expected answer for a few inputs. This function should work regardless of whether x is negative or not, and you should not use the ‘+’ symbol anywhere in your solution, nor any constants. To find the solution to this task, you should use your understanding of integer operations to find a way to add 1.
Hint: consider what happens when you do -x. Could you use it with other operations to get x+1?.
#include <stdio.h>#include <stdbool.h>#include <stdlib.h>
// BEGIN STRINGBUILDER IMPLEMENTATION
// This code is a very rudimentary stringbuilder-like implementation// To create a new stringbuilder, use the following line of code//// stringbuilder sb = new_sb();//// If you want to append a character to the stringbuilder, use the// following line of code. Replace whatever character you want to// append where the 'a' is.////…
Implement the FindText() function, which has two strings as parameters. The first parameter is the text to be found in the user provided sample text, and the second parameter is the user provided sample text. The function returns the number of instances a word or phrase is found in the string. In the PrintMenu() function, prompt the user for a word or phrase to be found and then call FindText() in the PrintMenu() function. Before the prompt, call cin.ignore() to allow the user to input a new string.Ex:
Enter a word or phrase to be found: more "more" instances: 5
Chapter 2 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 2 - Prob. 1TFCh. 2 - Prob. 2TFCh. 2 - Prob. 3TFCh. 2 - Prob. 4TFCh. 2 - Prob. 5TFCh. 2 - Prob. 6TFCh. 2 - Prob. 7TFCh. 2 - Prob. 8TFCh. 2 - Prob. 9TFCh. 2 - Prob. 10TF
Ch. 2 - Prob. 1MCCh. 2 - Prob. 2MCCh. 2 - Prob. 3MCCh. 2 - Prob. 4MCCh. 2 - Prob. 5MCCh. 2 - Prob. 6MCCh. 2 - Prob. 7MCCh. 2 - Prob. 8MCCh. 2 - Prob. 9MCCh. 2 - Prob. 10MCCh. 2 - Prob. 1DCh. 2 - Prob. 3DCh. 2 - Prob. 4DCh. 2 - Prob. 5DCh. 2 - Prob. 6DCh. 2 - Prob. 7DCh. 2 - Prob. 1PECh. 2 - Prob. 2PECh. 2 - Prob. 3PECh. 2 - Prob. 4PECh. 2 - Prob. 5PECh. 2 - Prob. 6PECh. 2 - Prob. 7PECh. 2 - Prob. 8PECh. 2 - Prob. 9PECh. 2 - Prob. 10PECh. 2 - Prob. 11PECh. 2 - Prob. 12PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- For this project, you will import the **time** and **random** modules. You will also install the **matplotlib** package and import from it the **pyplot** module. You will also import the wraps() function from the functools module for use in your decorator.Use the **time** module to write a decorator function named sort_timer that times how many seconds it takes the decorated function to run. Since sort functions don't need to return anything, have the decorator's wrapper function return that elapsed time.To get the current time, call time.perf_counter(). Subtracting the begin time from the end time will give you the elapsed time in seconds.Copy the code for bubble sort and insertion sort from Module 4 and decorate them with sort_timer. def bubble_sort(a_list): """ Sorts a_list in ascending order """ for pass_num in range(len(a_list) - 1): for index in range(len(a_list) - 1 - pass_num): if a_list[index] > a_list[index + 1]:…arrow_forwardAdd a validation function using Javascript that does the following 2 things: validates that password entered by the user and reentered password match. Note that minlength ensures it's a minimum of 8 of characters. Test thoroughly!!! Note that usually you would want to check that password meets some minimum requirements such as it includes both upper and lowercase letters and either a number or special character. In Javascript, we use regular expressions to do this. For this assignments, just make sure the password and reentered password match. validates that the username isn't already taken. Create an array of existing valid usernames. Include Harry, Elizabeth, and Shana.arrow_forwardOn python, use the matplotlib library and not any other library, to plot the following functions. Please comment every line on the codearrow_forward
- Rewrite Exercise 3.4 using the following func tion to return the area of a pentagon: def area(s):arrow_forwardplease write the python code to implement without error for this problem. I am stuck.arrow_forwardImplement the function total_price, which takes in a list of prices of individual products and needs to find the total price. Unfortunately, any product that is priced greater than or equal to $20 has a 50 percent tax, so include that in the final price. Try to do this in one line! Cast your final answer to an integer to avoid floating point precision errors. For example, if x contains your final answer, return int(x)! def total_price(prices): Finds the total price of all products in prices including a 50% tax on products with a price greater than or equal to 20. >>> total_price ([5, 20, 30, 71) 87 total_price([8, 4, 3]) >>> total_price ([10, 100, 4]) 164 >>> 15 "*** YOUR CODE HERE ***"arrow_forward
- Consider again a list of fridge temperatures that should be kept within a certain range, as described in Question 9. Write a program that prints the percentage of readings that are outside the allowed range (0 to 5 degrees Celsius), as shown in the examples below. Note that the percentage is printed as an integer, rounded down, and that there is a space before the percentage sign. You need to decompose this problem into sub-problems and instantiate and combine the corresponding patterns. If you need to compute the length or sum of a list, you may use the list operations len() and sum() instead of programming them from scratch using the counting and aggregator patterns. Use variable names appropriate to the problem at hand instead of the generic names used by the patterns. Use the get_input() operation to get the input list. For example: Input Result [5, 4, 3, 2, -1, 0] 16 % of values are outside the range [4.7, 5.2] 50 % of values are outside the range…arrow_forwardWrite a function called has_duplicates that takes a string parameter and returns True if the string has any repeated characters. Otherwise, it should return False. Implement has_duplicates by creating a histogram using the histogram function above. Do not use any of the implementations of has_duplicates that are given in your textbook. Instead, your implementation should use the counts in the histogram to decide if there are any duplicates. Write a loop over the strings in the provided test_dups list. Print each string in the list and whether or not it has any duplicates based on the return value of has_duplicates for that string. For example, the output for "aaa" and "abc" would be the following. aaa has duplicatesabc has no duplicates Print a line like one of the above for each of the strings in test_dups. test_dups = ["zzz","dog","bookkeeper","subdermatoglyphic","subdermatoglyphics"]arrow_forwardImplement a function, countVowels, which takes in a string and counts the number of vowels that are contained within it. Make sure that both capital vowels and lowercase vowels are counted. Your starter code contains a main function, which asks for user input and outputs the number of vowels. You will need to implement the user input for the string. Sample Runs (user input is italicized and underlined): Enter a phrase: Hello World!Number of vowels: 3 Enter a phrase: PROGRAMMING IS EXCELLENT.Number of vowels: 7 #include <string>#include <iostream>using namespace std; int countVowels(string word); int main(){ string phrase; cout << "Enter a phrase: "; // To do: implement user input cout << "Number of vowels: " << countVowels(phrase) << endl;} // To do: Implement countVowels int countVowels(string word){}arrow_forward
- Imagine if the survey contained two sheets in the same book. Perhaps sheet 1 isshoes and sheet 2 is dresses.The work now doubles.What about more than two sheets?You get the picture. This needs some sort of automation.Write ALGORITHM FOR MERGING SHEETSarrow_forwardcan you add a screenshot showing that you are writing and running the code in Matlab/Octave.arrow_forwardAnswer the given question with a proper explanation and step-by-step solution. Below is your function add(t) from 6.4 which adds all items in the tuple t and returns the result. This function is unprotected and will fail whenever the tuple t contains an item that is not a number. Run the program to see it. Protect your function with the try-except statement! If for whatever reason the addition fails, display the error message and return None. Do not change the main program. Expected output: Tuple of values: (1, 'two', 3)unsupported operand type(s) for +=: 'int' and 'str'Sum of the values: None def add(t):"""Adds all values in the tuple t""" result = 0 for x in t:result += x return result # Main program (do not change):values = (1, 'two', 3)print("Tuple of values:", values)print("Sum of the values:", add(values))arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
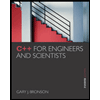
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
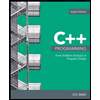
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
What Are Data Types?; Author: Jabrils;https://www.youtube.com/watch?v=A37-3lflh8I;License: Standard YouTube License, CC-BY
Data Types; Author: CS50;https://www.youtube.com/watch?v=Fc9htmvVZ9U;License: Standard Youtube License