STARTING OUT WITH C++ MPL
9th Edition
ISBN: 9780136673989
Author: GADDIS
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 17, Problem 11PC
Program Plan Intro
Generation of Subsets
Program Plan:
- Include the required header files
- Declare function prototypes.
- Define the “main()” function.
- Declare the required variables.
- Get the input from the user.
- If the user input is not in the range the condition exits the program.
- Create the list and call the function “get_Subsets ()”.
- Display the output.
- Define the overloaded stream insertion operator for
vector of int.- Display the open square bracket.
- If the number is in the range, display the number inside the bracket.
- Display the close square bracket.
- Return the output to the main function.
- Define the overloaded stream insertion operator for a list of generic type.
- Display the open square bracket.
- Create a list and declare the variable name “itr” for the list.
- While condition used to check if the “itr” is not equal to last number in the same list.
- If so, display the number.
- Increment the “itr”.
- Print the numbers separated by commas.
- Display the close square bracket.
- Return the output to the main function.
- Define the “get_subsets” vector function.
- Declare the list of subsets
- Start with a list of subsets of 1 to n that contains on the empty set.
- The “if” condition is used to check if the “n” is greater than “k” value.
- Temporarily used to extend the subset list by declaring the vector variables.
- While condition used to check if the “itr” is not equal to last number in the same list.
- Declare the vector variables.
- Push the value to the list.
- Increment the “itr” value.
- Set the “sub_setList” variable with the “big_List” value.
- Return the value to the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Introduction
For this assignment, you are to write a program which implements a Sorted List data structure using a circular
array-based implementation and a driver program that will test this implementation.
The Sorted List ADT is a linear collection of data in which all elements are stored in sorted order. Your
implementation has to store a single int value as each element of the list and support the following operations:
1. add(x) – adds the integer x to the list. The resulting list should remain sorted in increasing order. The time
complexity of this operation should be 0(N), where N is the size of the list.
2. removefirst() - deletes the first integer from the list and returns its value. The remaining list should
remain sorted. Time complexity of this operation should be 0(1).
3. removelast() – deletes the last integer from the list and returns its value. The remaining list should
remain sorted. Time complexity of this operation should be 0(1).
4. exists(x) – returns true if the…
1. a function that takes in a list (L), and creates a copy of L.
note: The function should return a pointer to the first element in the new L. [iteration and recursion].
2. a function that takes in 2 sorted linked lists, and merges them into a single sorted list.
note: This must be done in-place, and it must run in O(n+m).
Create a function set(v, i, j) that makes vector v contain the integers i through j. The original contents of v is deleted. The vector is left empty ifj < i. The argument v is a vector of integers.
Chapter 17 Solutions
STARTING OUT WITH C++ MPL
Ch. 17.1 - Prob. 17.1CPCh. 17.1 - Prob. 17.2CPCh. 17.1 - Prob. 17.3CPCh. 17.1 - Prob. 17.4CPCh. 17.2 - Prob. 17.5CPCh. 17.2 - Prob. 17.6CPCh. 17.2 - Why does the insertNode function shown in this...Ch. 17.2 - Prob. 17.8CPCh. 17.2 - Prob. 17.9CPCh. 17.2 - Prob. 17.10CP
Ch. 17 - Prob. 1RQECh. 17 - Prob. 2RQECh. 17 - Prob. 3RQECh. 17 - Prob. 4RQECh. 17 - Prob. 5RQECh. 17 - Prob. 6RQECh. 17 - Prob. 7RQECh. 17 - Prob. 8RQECh. 17 - Prob. 9RQECh. 17 - Write a function void printSecond(ListNode ptr}...Ch. 17 - Write a function double lastValue(ListNode ptr)...Ch. 17 - Write a function ListNode removeFirst(ListNode...Ch. 17 - Prob. 13RQECh. 17 - Prob. 14RQECh. 17 - Prob. 15RQECh. 17 - Prob. 16RQECh. 17 - Prob. 17RQECh. 17 - Prob. 18RQECh. 17 - Prob. 1PCCh. 17 - Prob. 2PCCh. 17 - Prob. 3PCCh. 17 - Prob. 4PCCh. 17 - Prob. 5PCCh. 17 - Prob. 6PCCh. 17 - Prob. 7PCCh. 17 - Prob. 8PCCh. 17 - Prob. 10PCCh. 17 - Prob. 11PCCh. 17 - Prob. 12PCCh. 17 - Running Back Program 17-11 makes a person run from...Ch. 17 - Read , Sort , Merge Using the ListNode structure...
Knowledge Booster
Similar questions
- Write a function called genericSort() that takes in a numeric or integer vector, sorts it, and returns the indices of the sorted values. It should also print an error if the input is a character. For example, genericSort(c(1,3,7,5)) should return the vector (1,2,4,3). Programming in Rarrow_forwardCan you help with with the following questions, regarding population genetics and the Wright Fisher model in python? 1. Go through the given WFtrajectory function and give a short explanation of what each line of code does. Pay attention to the variables in the function and explain what they contain. 2. Make a new version of the WFtrajectory function which does exactly the same thing except that instead of appending to a list it initialises a numpy vector with zeroes and fills the vector with a for loop instead of a while loop. Make a plot of the output from a few example trajectories.arrow_forwardQUESTION: NOTE: This assignment is needed to be done in OOP(c++/java), the assignment is a part of course named data structures and algorithm. A singly linked circular list is a linked list where the last node in the list points to the first node in the list. A circular list does not contain NULL pointers. A good example of an application where circular linked list should be used is a items in the shopping cart In online shopping cart, the system must maintain a list of items and must calculate total bill by adding amount of all the items in the cart, Implement the above scenario using Circular Link List. Do Following: First create a class Item having id, name, price and quantity provide appropriate methods and then Create Cart/List class which holds an items object to represent total items in cart and next pointer Implement the method to add items in the array, remove an item and display all items. Now in the main do the following Insert Items in list Display all items. Traverse…arrow_forward
- In C++ Question A linked list is said to contain a cycle if any node is visited more than once while traversing the list. Given a pointer to the head of a linked list, determine if it contains a cycle. If it does, return 1. Otherwise, return 0. Example: head refers to the list of nodes 1 → 2 → 3 → 1 → NULL. There is a cycle where node 3 points back to node 1, so return 1. Function Description: Complete the has cycle function provided in the moodle assignment folder. It has the following parameter: • SinglyLinkedListNode pointer head: a reference to the head of the list. Returns: • int: 1 if there is a cycle or 0 if there is not. Note: if the list is empty, head will be null. Input Format: The code stub reads from stdin and passes the appropriate argument to your function. The custom test cases format will not be described for this question due to its complexity. Expand the section for the main function and review the code if you would like to figure out how to create…arrow_forwardTROUBLESHOOT my PYTHON code, please :) The code of a sequential search function is shown on textbook page 60. In fact, if the list is already sorted, the search can halt when the target is less than a given element in the list. For example, given my_list = [2, 5, 7, 9, 14, 27], if the search target is 6, the search can halt when it reaches 7 because it is impossible for 6 to exist after 7. Define a function linearSearchSorted, which is used to search a sorted list. This function displays the position of the target item if found, or 'Target not found' otherwise. It also displays the elements it has visited. To test your function, search for these targets in the list [2, 5, 7, 9, 14, 27]: 2, 6, 14, 27 and 28. Expected output: List: [2, 5, 7, 9, 14, 27] Search target: 2 Elements visited: 2 Target found at position 0 Search target: 6 Elements visited: 2 5 7 Target not found Search target: 14 Elements visited: 2 5 7 9 14 Target found at position 4 Search target: 27 Elements visited: 2…arrow_forwardExercise B: "Matrix Addition" For this exercise you will design and implement a function that takes two matrix arguments and computes their sum (if and only if a sum can actually be computed, returning an empty list otherwise). Two matrices can only be added together if they have exactly the same dimensions. If the dimensions are acceptable, then the matrix sum will have the same dimensions as either of the operands, and the value of each element in the matrix sum is itself the sum of the corresponding elements in the operand matrices. And although it does makes sense to test rectangularity before attempting addition, you need not perform this step (since you did it above). In order to complete this task, you will need to: ensure you know how matrix addition can be performed² ● Your submission for this exercise:arrow_forward
- [] [] partite_sets In the cell below, you are to write a function "partite_sets (graph)" that takes in a BIPARTITE graph as its input, and then returns a single list whose two entries are the partite sets of the graph as lists (the order of the sets outputed does not matter). After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (partite_sets({"A" : ["B", "C"], "B" : partite_sets({"A" : ["B", "C"], "B" : ["A"], "C" : ["A"]}), ["A", "D"], "C" : ["A", "D"], "D" : ["B", "C"]})) This should return [["B","C"], ["A"]] [["A","D"], ["B","C"]] (the order in which the entries appear does not matter) Python Pythonarrow_forward6. Write a function DOT-PRODUCT that takes two lists, each list has the same number of elements, and produces the dot product of the vectors that they represent. For example, (DOT-PRODUCT '(1.2 2.0 -0.2) '(0.0 2.3 5.0)) -> 3.6 Hint: The key for designing a lisp function is using recursion.arrow_forwardExercise G -- Implement a function halves that takes a list of integers and divides each element of the list by two (using the integer division operator //) NOTE use the map function combined with a lambda expression to do the division with a neat solution halves : List Int -> List Int halves xs = [ ] --remove this line and implement your halves function herearrow_forward
- Old MathJax webview Old MathJax webview In Java Some methods of the singly linked list listed below can be implemented efficiently (in different respects) (as opposed to an array or a doubly linked list), others not necessarily which are they and why? b. Implement a function to add an element before the first element. c. Implement a function to add an item after the last one element. d. Implement a function to output an element of the list. e. Implement a function to output the entire list. f. Implement a function to output the number of elements. G. Implement a function to delete an item. H. Implement a function to clear the entire list. I. Implement functionality to search for one or more students by first name, last name, matriculation number or course of study. J. Implement functionality to sort the records of the student, matriculation number and course according to two self-selected sorting methods.arrow_forwardPython data structures: write a function that takes in a list (L) as input, and returns the number of inversions. An inversion in L is a pair of elements x and y such that x appears before y in L, but x > y. Your function must run in -place and implement the best possible algoritm of time complexity. def inversions(L: List) -> int:'''finds and returns number of inversions'''arrow_forwardWrite the following data structures in R: Vectors, Matrices, Arrays, Lists, and Data Frames. Access R Studio. Then, demonstrate how to work with each data structure as outlined below. Vectors Create a vector as a sequence of number 1-15. Create a vector as a sequence of numbers 1-15 that increment by 0.1. Demonstrate how missing data is represented as NA in vectors (use the na() and anyNA() functions). Utilize the conversion between modes "coercion" in R to perform an "implicit coercion" on the following: a) xx ß p(2.5, "g"), b) xx ß p(TRUE, 4) and c) xx ß p("g", TRUE). Control how vectors are coerced explicitly using the as.<class_name>() functions (numeric and character). Matrices Create a column-wise 5 x 8 matrix. Check that the matrices are vectors with a class attribute of matrix by using class() and tyepof(). Create a matrix by transforming a 5 x 8 vector into a matrix. Arrays Create an array of movies that contains the Top 10 movies of 2020. Lists Construct a list…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
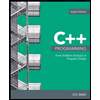
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning