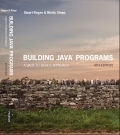
Explanation of Solution
Adding the “lastIndexOf” methods in “ArrayIntList” class:
//definition of "ArrayIntList" class
public class ArrayIntList
{
//declare the required variables
private int[] elementData;
private int size;
//refer the remaining methods in the textbook
//definition of "lastIndexOf" method
public int lastIndexOf(int value)
{
/*iterate "i" until it reaches the size of an array*/
for (int i = size - 1; i >= 0; i--)
{
//check the condition
if (elementData[i] == value)
{
//return the "i" value
return i;
}
}
//return the value
return -1;
}
}
Explanation:
In the above program, the “ArrayIntList” is the class name,
- Inside the “ArrayIntList” class, declare the “elementData”, and “size” variables as a private integer datatype.
- The “lastIndexOf” method is defined inside the “ArrayIntList” class with the integer value as the parameter.
- The “for” loop is used to iterate the value until it reaches size of an array.
- The “if” loop is used to check whether the array of element value is equal to passed value.
- The “ith” position of an array value is returned.
- The “if” loop is used to check whether the array of element value is equal to passed value.
- If the value is not in an array return the -1 value.
- The “for” loop is used to iterate the value until it reaches size of an array.
- The “lastIndexOf” method is defined inside the “ArrayIntList” class with the integer value as the parameter.
Want to see more full solutions like this?
Chapter 15 Solutions
EBK BUILDING JAVA PROGRAMS
- 1. Write a method called doubleListthat takes an ArrayList of strings as a parameter and replaces every string with two of that same string. For example, if the list stores the values ["how", "are", "you?"] before the method is called, it should store the values ["how", "how", "are", "are", "you?", "you?"] after the method finishes executing.In your program1, you also need to create a class namedProgram1.java, where you are going to construct some arralylists to test the doubleList within the static void main method. In the output, it will print out the original list and the new list after calling the doubleList method. 2.Write a method called filterRange that accepts an ArrayList of integers and two integer values min and max as parameters and removes all elements whose values are in the range min through max (inclusive). For example, if a variable calledlist stores the values [4, 7, 9, 2, 7, 7, 5, 3, 5, 1, 7, 8, 6, 7], the call of filterRange(list, 5, 7);should remove all values…arrow_forward1. Write a method countCommon that accepts two lists of integers as parameters and returns the number of unique integers that occur in both lists. Use one or more sets as storage to help you solve this problem. For example, if one list contains the values [3, 7, 3, –1, 2, 3, 7, 2, 15, 15] and the other list contains the values [–5, 15, 2, –1, 7, 15, 36], your method should return 4 because the elements –1, 2, 7, and 15 occur in both lists. Your method should return correct result with different lists as well. 2. Write a method maxLength that accepts a set of strings as a parameter and that returns the length of the longest string in the list. If your method is passed an empty set, it should return 0.In your project COSC241<Lab4>_<your username>, create Lab4_yourusername.java and declare proper lists and sets to call the two methods countCommon and maxLength. And your output should show the two methods work as expected.Make sure you have comments through your program.arrow_forwardyou are given a variable zipcode_list that contains a list. Write some code that assigns True to the variable duplicates if the there are two adjacent elements in the list that have the same value, but that otherwise assigns False to duplicates otherwise. use only variables k, zipcode_list, and duplicatesarrow_forward
- Write a method called stutter that replaces every value with two of that value. For example, if the list initially stores [42, 7, 0, –3, 15], after the call it should store [42, 42, 7, 7, 0, 0, –3, –3, 15, 15].arrow_forwardWrite the following method that partitions the list using thefirst element, called a pivot:public static int partition(int[] list)After the partition, the elements in the list are rearranged so all the elements beforethe pivot are less than or equal to the pivot, and the elements after the pivot aregreater than the pivot. The method returns the index where the pivot is located inthe new list. For example, suppose the list is {5, 2, 9, 3, 6, 8}. After the partition,the list becomes {3, 2, 5, 9, 6, 8}. Implement the method in a way that takes atmost list.length comparisons. See liveexample.pearsoncmg.com/dsanimation/QuickSortNeweBook.html for an animation of the implementation. Write atest program that prompts the user to enter the size of the list and the contents ofthe list and displays the list after the partition. Here is a sample run. Enter list size: 8 ↵EnterEnter list content: 10 1 5 16 61 9 11 1 ↵EnterAfter the partition, the list is 9 1 5 1 10 61 11 16arrow_forwardJava Write a java program that calls a method called removeZeroes that takes as a parameter anArrayList of integers and eliminates any occurrences of the number 0 from the list. For example,if the list stores the values [0, 4, 5, 0, 6, 0, 1] before the method is called, it should store the values [4, 5, 6, 1] after the method finishes executing. Print the ArrayList before and after the method call.arrow_forward
- Complete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forwardWrite a method called compareSum to be considered in a class outside the KWArrayList class. This method has a parameter list of class type KWArrayList . The method finds the summation of even elements for the first half of list and the summation of odd elements for the second half of list, then compares between the two summations and returns the greater sum. Assume the length of List is even. Method heading: public static int compareSum( KWArrayList<Integer> list) Before run: list= 10 5 50 3 14 33 20 5 (note: the numbers in bold represent the first half) After run: list= 3 5 50 14 33 20 5 Sum1 (for the first half) = 60 Sum2 (for the second half) = 38 Returned value is 60arrow_forwardWrite a Java application CountryList. In the main method, do the following:1. Create an array list of Strings called countries.2. Add "Canada", "India", "Mexico", "Peru" in that order.3. Use the enhanced for loop to print all countries in the array list, one per line.4. Add "Spain" at index 15. Replace the element at index 2 with "Vietnam". You must use the set method.6. Replace the next to the last element with "Brazil". You must use the set method. You willlose one point if you use 3 in the set method. Do this in a manner that would replace thenext to the last element, no matter the size of the array list.7. Remove the object "Canada" Do not remove at an index. Your code should work if"Canada" was at a different location. There is a version of remove method that willremove a specific object.8. Get and print the first element followed by "***"9. Call method toString() on countries to print all elements on one line.10. Use the enhanced for loop to print all countries in the array list,…arrow_forward
- Create the Median class that has the method calculateMedian that calculates the median of the integers passed to the method. Use method overloading so that this method can accept anywhere from two to five parameters. How to calculate medianThe median can be thought of as the "middle" number in an ordered list of numbers. There are two numbers in the "middle", the median is the average of these two numbers. Method Call Return Value m.calculateMedian(3, 5, 1, 4, 2) 3.0 m.calculateMedian(8, 6, 4, 2) 5.0 m.calculateMedian(9, 3, 7) 7.0 m.calculateMedian(5, 2) 3.5 Hint Finding the median requires that the numbers be in order. You can use the method Arrays.sort(myArray); to sort an array of integers.arrow_forwardWrite a static method called flip2 that takes an ArrayList of Strings as a parameter and then flips each successive pair of values in the list. For example, suppose that a variable called list stores the following sequence of values: ["aa", "bb", "cc", "DDD", "happy", "snow"] and we make the following call: flip (list); Afterwards the list should store the following sequence of values: ["bb", "aa", "DDD", "cc", "snow", "happy"] If the list has extra values that are not part of a pair, those values are unchanged. For example, if the list had instead stored: ["aa", "bb", "cc", "DDD", "happy", "snow", "hometown"] The result would have been: ["bb", "aa", "DDD", "cc", "snow", "happy", "hometown"]arrow_forwardQuestion 2: In a demo class, write a public static method called removeLastOccurenceTen that receives a reference parameter named list of type LLNode of integer values. Your method should remove the node that contains the last occurrence of the number 10 in the list, if exist. For example, if the list contains: list 4 10 1 5 10 2 10 7 Then after calling the method removeMaxNode, the list became: list 4 10 1 5 10 2 7arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
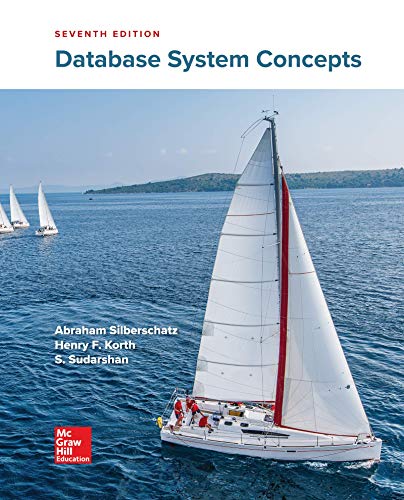
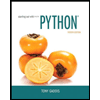
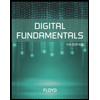
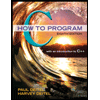
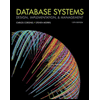
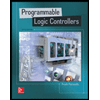