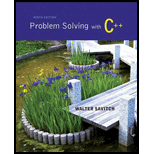
The computer player in

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- Write in C++ Alice is trying to monitor how much time she spends studying per week. She going through her logs, and wants to figure out which week she studied the least, her total time spent studying, and her average time spent studying per week. To help Alice work towards this goal, write three functions: min(), total(), and average(). All three functions take two parameters: an array of doubles and the number of elements in the array. Then, they make the following computations: min() - returns the minimum value in the array sum() - returns the sum of all the values in the array average() - returns the average of all the values in the array You may assume that the array will be non-empty. Function specifications: Function 1: Finding the minimum hours studied Name: min() Parameters (Your function should accept these parameters IN THIS ORDER): arr double: The input array containing Alice's study hours per week arr_size int: The number of elements stored in the array Return Value:…arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Implement function heads() that takes no input and simulates a sequence of coin flips.The simulation should continue as long as 'HEAD' is flipped. When the outcome of a flipis 'TAIL', the function should return the number of 'HEAD' flips made up to that point.For example, if the simulation results in outcomes 'HEAD', 'HEAD', 'HEAD', 'TAIL', thefunction should return 3. NOTE: Recall that random.choice(['HEAD', 'TAIL']) returns'HEAD' or 'TAIL' with equal probability.>>> heads()0 # TAIL is the outcome of the first coin flip>>> heads()2 # The coin flips were HEAD, HEAD, TAIL>>> heads()1 # The coin flips were HEAD, TAIL>>> heads()5 # The coin flips were HEAD, HEAD, HEAD, HEAD, HEAD, TAILarrow_forwardYour first task will be to write a simple movement simulator on a snakes and ladders board. For this questionyou can ignore the snakes and ladders and just simply assume you only have to deal with moving. You willneed to simulate rolling the die - this can be done by using the Python random module and the randint method.Your function play_game will take as input the length of the board (an integer), "play" the game by rolling the diemultiple times until the sum of rolls is larger or equal to the length of the board. (note: this is one of the possibleand simplest end rules). The function should return the total number of rolls required to finish the the particulargame that was played. Obviously this number will vary as it depends on the specific random rolls performedduring the movement simulation.arrow_forward
- Write the definitions of the member functions of the classes arrayListType and unorderedArrayListType that are not given in this chapter. The specific methods that need to be implemented are listed below. Implement the following methods in arrayListType.h: isEmpty isFull listSize maxListSize clearList Copy constructor Implement the following method in unorderedArrayListType.h insertAt Also, write a program (in main.cpp) to test your function.arrow_forwardSuppose that an array is passed as a parameter. How does this differ from the usual use of a value parameter? When an array is passed as a parameter, it is like passed by reference. A new array will be created in the called function and any changes to the new array will pass back to the original array. When an array is passed as a parameter, it is passed by value. So any changes to the parameter do not affect the actual argument. When an array is passed as a parameter, changes to the array affect the actual argument. This is because the parameter is treated as a pointer that points to the first component of the array. This is different from a value parameter (where changes to the parameter do not affect the actual argument). When an array is passed as a value, changes to the array affect the actual argument. This is because the parameter is treated as a pointer that points to the first component of the array. This is different from a parameter (where changes to the parameter do not…arrow_forwardIn C++ Language using Functions and Pointers PROBLEM: Consider the elevator in the Faculty of Engineering (5 storey). Write a program which accepts the floor number where a passenger (student/faculty/other personnel) will ride the elevator. The passenger can then input the target destination floor. SPECIFICATIONS: Implement the class diagram below: Inside the main method, create an instance of the class Elevator. Use the goUp or goDown methods to generate the program display.Passenger can ride in any floor (1,2,3,4 or 5). Program will prompt the user with the target destination floor. After the user input: Program will display the floors which the elevator passes through, and finally the destination floor. Elevator will not move if the input for destination floor is the same as the present floor. Program will notify the user and then terminate. Program will also terminate if the input destination is not 1,2,3,4 or 5. You’re free to format the input/output display. Observe proper…arrow_forward
- language is c++ sample output included with user input in boldarrow_forwardThis assignment is supposed to represent a group of students in a course. A group of students in a course will be assigned an assignment and produce a collection of assignment results. The results will be used to figure out grade statistics. The grade they receive for their work on the assignment is entirely dependant on the student's energy level. If the student works on many assignments without sleeping their grade will suffer. You will implement four classes (Assignment, AssignmentResult, Student, and Course), they will depend on each other in the order they are listed. Hint: Work on the methods in the order they are found in the documentation below, implement the getter and setter methods before the more complicated methods. Work on the Assignment class, AssignmentResult class, Student class and Course class in that order. Make sure you don't name your class variables the same name as you class's methods. In other words if you have method named id you cannot have a class…arrow_forwardUOWD Library is asking you to write a Java program that manages all the items in the Library. The library has books, journals, and media (DVD for example). All items have a name, author(s), and year of publication. A journal also has a volume number, while a media has a type (audio/video/interactive). The user of your program should be able to add an item, delete an item, change information of an item, list all items in a specific category (book, journal, or media), and print all items (from all categories). A menu asks the user which operation s/he wants to perform. Important: make use of collections, inheritance, interfaces, and exception handling wherever appropriate.arrow_forward
- Write a program to add members of two different classes using friend Function. Problem: Design: Code: Output:arrow_forwardMoving Between Rooms - Navigation In this assignment, you will be working with a given "rooms" dictionary and associated constants to create a simple text-based game. Your main task is to develop a function that allows the player to navigate through the rooms based on the given specifications. You need to implement the function found in the starter code to the right The function should take into account the following conditions: If the direction leads to an exit, set the next room to the exit and the message to "Goodbye". If the direction is invalid, set the next room to the current room and the message to "No such direction". If the direction is valid, but you cannot go that way, set the next room to the current room and the message to "You bumped into a wall". If the direction is valid and you can go that way, set the next room to the room in that direction and the message to "Empty". To help you understand how the function will be integrated into the gameplay loop, the following…arrow_forwardIN C++ USING CLASS Write a program in C++ that implements the game tic-tac-toe: Tic-Tac-Toe is a game played on a three-by-three board. Two players, X and O, alternate in placing their respective marks in the cells of this board, starting with player X. If either player succeeds in getting three of his or her marks in a row, column, or diagonal, then that player wins. In this homework, you should use the OOP concepts to implement the tic-tac-toe gamearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
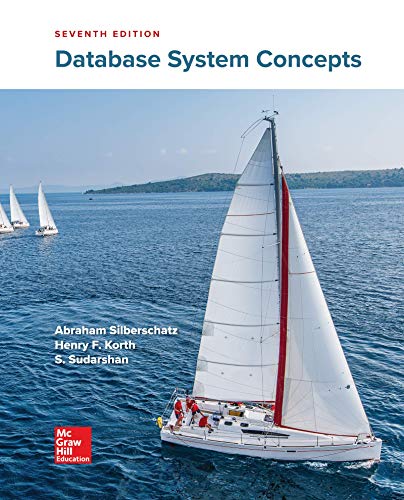
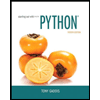
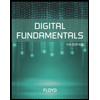
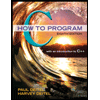
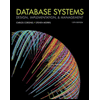
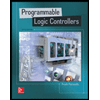