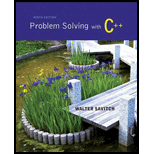
Problem Solving with C++ (9th Edition)
9th Edition
ISBN: 9780133591743
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 14, Problem 8PP
Program Plan Intro
Finding all permutations for a set
Program Plan:
- Include required file.
- Define the structure for node.
- Declare elements in “vector” type.
- Declare variable for next value in “NodeValue” type.
- Declare function for display permutations.
- Declare function for compute permutations with recursively.
- Declare function for display vector elements of set.
- Define main function.
- Call the function “displayPermutations” with one parameter.
- Define function “displayPermutations”.
- Create a pointer for node.
- Declare the set in “vector” type.
- Fill the set with first “n” whole elements.
- Call the function “displayVectorElements” to print the
vectors . - Then compute the permutation for given set by calling the function “recursivePermutations”.
- Performs “while” loop. This loop executes until the pointer is equal to “NULL”.
- Display the values in set by calling the function “displayVectorElements”.
- Then delete and move to the next value.
- Define function “recursivePermutations”.
- This function is used to returns a list holding all of the permutations of the given list of elements.
- In this function, first assign the pointer list to “NULL”.
- Then performs base case if the size of the vector element is “1”. Otherwise performs recursive case.
- Compute the permutations for smaller set of elements by recursively call the function “recursivePermutations”.
- Define function “displayVectorElements”.
- This function is used to display the elements of set.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In chess, a walk for a particular piece is a sequence of legal moves for that piece, starting from a square of your choice, that visits every square of the board. A tour is a walk that visits every square only once. (See Figure 5.13.)
5.13 Prove by induction that there exists a knight’s walk of an n-by-n chessboard for any n ≥ 4. (It turns out that knight’s tours exist for all even n ≥ 6, but you don’t need to prove this fact.)
Algorithm design with sorting. Each of n users spends some time on a social media site. For each i = 1, . . . , n, user i enters the site at time ai and leaves at time bi ≥ ai. You are interested in the question: how many distinct pairs of users are ever on the site at the same time? (Here, the pair (i, j) is the same as the pair (j, i)).Example: Suppose there are 5 users with the following entering and leaving times:
Then, the number of distinct pairs of users who are on the site at the same time is five: these pairs are (1, 2), (1, 3), (2, 3), (4, 6), (5, 6). (Drawing the intervals on a number line may make this easier to see).(a) Given input (a1 , b1),(a2 , b2), . . . ,(an, bn) as above in no particular order (i.e., not sorted in any way), describe a straightforward algorithm that takes Θ(n2)-time to compute the number of pairs of users who are ever on the site at the same time, and explain why it takes Θ(n2)-time. [We are expecting pseudocode and a brief justification for its…
Recursive filtering techniques are often used to reduce the computational complexity of
a repeated operation such as filtering. If an image filter is applied to each location in an
image, a (horizontally) recursive formulation of the filtering operation expresses the result
at location (x +1, y) in terms of the previously computed result at location (x, y).
A box convolution filter, B, which has coefficients equal to one inside a rectangular win-
dow, and zero elsewhere is given by:
w-1h-1
B(r, y,w, h) =
ΣΣΤ+ i,y + )
i=0 j=0
where I(r, y) is the pixel intensity of image I at (x, y). We can speed up the computation
of arbitrary sized box filters using recursion as described above. In this problem, you will
derive the procedure to do this.
(a) The function J at location (x,y) is defined to be the sum of the pixel values above
and to the left of (x,y), inclusive:
J(r, y) =
- ΣΣ14.0
i=0 j=0
Formulate a recursion to compute J(r, y). Assume that I(r, y) = 0 if r <0 or y < 0.
Hint: It may be…
Chapter 14 Solutions
Problem Solving with C++ (9th Edition)
Ch. 14.1 - Prob. 1STECh. 14.1 - Prob. 2STECh. 14.1 - Prob. 3STECh. 14.1 - Prob. 4STECh. 14.1 - Prob. 5STECh. 14.1 - If your program produces an error message that...Ch. 14.1 - Write an iterative version of the function cheers...Ch. 14.1 - Write an iterative version of the function defined...Ch. 14.1 - Prob. 9STECh. 14.1 - Trace the recursive solution you made to Self-Test...
Ch. 14.1 - Trace the recursive solution you made to Self-Test...Ch. 14.2 - What is the output of the following program?...Ch. 14.2 - Prob. 13STECh. 14.2 - Redefine the function power so that it also works...Ch. 14.3 - Prob. 15STECh. 14.3 - Write an iterative version of the one-argument...Ch. 14 - Prob. 1PCh. 14 - Prob. 2PCh. 14 - Write a recursive version of the search function...Ch. 14 - Prob. 4PCh. 14 - Prob. 5PCh. 14 - The formula for computing the number of ways of...Ch. 14 - Write a recursive function that has an argument...Ch. 14 - Prob. 3PPCh. 14 - Prob. 4PPCh. 14 - Prob. 5PPCh. 14 - The game of Jump It consists of a board with n...Ch. 14 - Prob. 7PPCh. 14 - Prob. 8PP
Knowledge Booster
Similar questions
- Complete the task using the return search algorithm: Task: The labyrinth is represented by a Boolean square matrix A8x8. The cell (i, j) is considered passable if the element ai, j is true and otherwise impassable. Write a program that checks if there is a path from adjacent horizontally and vertically passable cells of the maze, which starts in its upper left corner (0.0) and ends in its lower right corner (7.7). Operating instructions: 1) Compose a Boolean function Path (i, j), called with initial parameters (0,0); 2) If cell (i, j) is outside the matrix Path returns false; 3) If cell (i, j) coincides with (7,7) a path is found and Path returns true; 4) If cell (i, j) is impassable Path returns false (because it cannot exit the cell), otherwise (if the cell is passable) there is a path from it to cell (7,7), if there is a path from any of the cells adjacent to (i, j) next to cell (7,7). Adjacent cells per cell (i, j) are cells (i + 1, j), (i, j + 1), (i-1, j), (i, j-1).arrow_forwardthe drop down bar is the selection you have to choose from for each answerarrow_forwardCorrect answer will be upvoted else Multiple Downvoted. Don't submit random answer. Computer science. each individual has a rating chart portrayed by a variety of integers an of length n. You are currently refreshing the foundation, so you've made a program to pack these diagrams. The program functions as follows. Given an integer boundary k, the program takes the base of each adjoining subarray of length k in a. All the more officially, for a cluster an of length n and an integer k, characterize the k-pressure exhibit of an as a cluster b of length n−k+1, to such an extent that bj=minj≤i≤j+k−1ai For instance, the 3-pressure cluster of [1,3,4,5,2] is [min{1,3,4},min{3,4,5},min{4,5,2}]=[1,3,2]. A stage of length m is an exhibit comprising of m unmistakable integers from 1 to m in subjective request. For instance, [2,3,1,5,4] is a stage, however [1,2,2] isn't a change (2 shows up twice in the exhibit) and [1,3,4] is likewise not a stage (m=3 but rather there is 4 in the…arrow_forward
- Design an algorithm that takes an array containing n distinct natural numbers. A number k ≤ n and calculates the sum of the k largest numbers in the array. For example, if the array is {3, 7, 5, 12, 6} and k = 3, then the algorithm should return 25 (12+7+6). You may freely use standard data structure/s and algorithms from the course in your solutionarrow_forwardCorrect answer will be upvoted else downvoted. Computer science. You are given an exhibit a comprising of n (n≥3) positive integers. It is realized that in this exhibit, every one of the numbers with the exception of one are something very similar (for instance, in the cluster [4,11,4,4] all numbers aside from one are equivalent to 4). Print the list of the component that doesn't rise to other people. The numbers in the cluster are numbered from one. Input The main line contains a solitary integer t (1≤t≤100). Then, at that point, t experiments follow. The main line of each experiment contains a solitary integer n (3≤n≤100) — the length of the exhibit a. The second line of each experiment contains n integers a1,a2,… ,an (1≤ai≤100). It is ensured that every one of the numbers aside from one in the an exhibit are something very similar. Output For each experiment, output a solitary integer — the list of the component that isn't equivalent to other people.arrow_forwardA magic square is a square array of non-negative integers where the sums of the numbers on each row,each column, and both main diagonals are the same. An N × N occult square is a magic square with Nrows and N columns with additional constraints:• The integers in the square are between 0 and N, inclusive.• For all 1 ≤ i ≤ N, the number i appears at most i times in the square.• There are at least two distinct positive integers in the square.For example, the following is a 5 × 5 occult square, where the sums of the numbers on each row, eachcolumn, and both main diagonals are 7:0 0 0 3 42 4 0 0 10 0 3 4 05 0 0 0 20 3 4 0 0For a given prime number P, you are asked to construct a P × P occult square, or determine whether nosuch occult square exists.InputInput contains a prime number: P (2 ≤ P ≤ 1000) representing the number of rows and columns in theoccult square.OutputIf there is no P × P occult square, simply output -1 in a line. Otherwise, output P lines, each contains Pintegers…arrow_forward
- Write an algorithm that uses an adjacency matrix, A[n][n], to determine if a digraph contains the back edge of one of its edges. (The back edge of an edge (i, j) is the edge (j, i).) 2.arrow_forwardPython: Graph Colouring A simple method to find a colouring of a graph, with vertices {0,1,…,?−1}, is the following greedy algorithm: For ? in 0, 1, 2, ..., n - 1: Find the smallest colour (positive integer) ? which is not a colour of any neighbour of ?. Assign ? as the colour of ?. i) Implement the method above as a function greedy_colouring which takes a networkx Graph G (with vertices {0,1,…,?−1}) and returns a list C of the colours of G, where C[i] is the colour of vertex ?. ii) As a test, the Hoffman-Singleton graph (nx.hoffman_singleton_graph()) should require 6 colours using this method. If you have a graph G, you can draw it with coloured vertices using the code below: ten_colours = ['#CC6677', '#332288', '#DDCC77', '#117733', '#88CCEE', '#882255', '#44AA99', '#999933', '#AA4499', '#DDDDDD'] nx.draw_networkx(G, node_color=[ten_colours[i - 1] for i in greedy_colouring(G)], with_labels=False) iii) One way of refining the…arrow_forwardExecute the following using Array Implementation. Use diagrams in showing your final answer A ENQUEUE(A) ENQUEUE(B) ENQUEUE(C) DEQUEUE() ENQUEUE(A) ENQUEUE(B) ENQUEUE(C) DEQUEUE() DEQUEUE() ENQUEUE(A) DEQUEUE() ENQUEUE(B) ENQUEUE(C) B PUSH (x, S) PUSH (y, S) PUSH (z, S) POP (S) PUSH (x, S) PUSH (y, S) POP (S) POP (S) C INSERT(Alice, 1, ) INSERT(Bob, 2, L) INSERT(Karen, 3, L) INSERT(Ram, 4, L) DELETE( 2, L) What will happen to your array?arrow_forward
- You are given a list of randomly arranged numbers, for example (11,7,18,5,17,13). The triple (11,7, 5) is called the "inversion triple" because (5<7<11) in terms of value, while the index of 5 in the list is greater than the index of 7, and the index of 7 is greater than 11. Therefore, we can find 2 inversions in such list as follows: (11,7,5), and (18,17,13). Your main task is to find the total number of inversions in any given list. a) Design a brute-force algorithm to return the number of possible inversions, and analyse the complexity of your solution b) Develop a python code to implement your brute-force algorithm. [The marks depend on the correctness of the code, indentation, comments, test-case] c) Design a more efficient algorithm to do the same task with less complexity, and analyse the complexity of your solution. [Important instruction to be followed: Create an arbitrary unsorted list of 8 characters and use it to provide full explanation of how your proposed…arrow_forwardGiven an array A[] of size n. The task is to find the largest element in it. Example 1: Input: n = 5 A[] = {1, 8, 7, 56, 90} Output: 90 Explanation: The largest element of given array is 90.arrow_forwardThere are m towns in a straight line, with a road joining each pair of consecutive towns. Legends say that an ordinary person in one of these towns will become a hero by completing a sequence of n quests. The first quest will be completed in their home town, but after each quest they may complete their next quest either in the same town or after moving to a neighbouring town.For example, if n = 5 and m = 4, a resident of town 2 may become a hero as follows:• begin in town 2 for quest 1,• then move to town 3 for quest 2,• stay in town 3 for quest 3,• return to town 2 for quest 4, and• move to town 1 for quest 5.Design an algorithm which runs in O(nm) time and finds the total number of waysto complete n quests.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
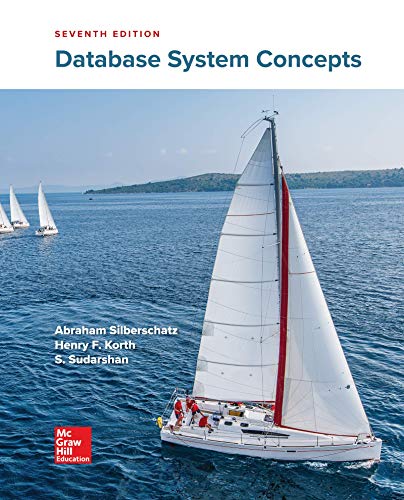
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
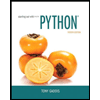
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
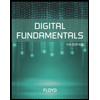
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
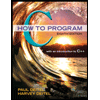
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
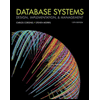
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
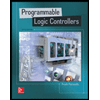
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education