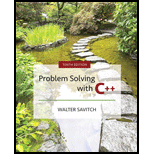
Explanation of Solution
Recursive function for checking palindrome:
The recursive function definition for checking the given string is a palindrome or not is shown below:
/* Function definition for checkPalindromeRecursion function */
bool checkPalindromeRecursion(string str)
{
/* If length is "0" or "1", then return "true" */
if(str.length() == 0 || str.length() == 1)
return true;
/* Check the first character*/
if(isdigit(str.at(0)))
{
/* If the first character is equal to the last character, then */
if(str.at(0) == str.at(str.length()-1))
/* Recursively call the function "checkPalindromeRecursion" with remaining characters */
return checkPalindromeRecursion(str.substr(1, str.length()-2));
}
//Otherwise
else
{
//For checking the lower characters
if(tolower(str.at(0)) == tolower(str.at(str.length()-1)))
return checkPalindromeRecursion(str.substr(1, str.length()-2));
}
//Otherwise returns "false"
return false;
}
Explanation:
The above function is used to check the given string is a palindrome or not using recursive function.
- If the length of string is “0” or “1”, then returns “true”.
- Check the first digit of given string. If it is, then check if the first character is equal to the last character, then recursively call the function “checkPalindromeRecursion” for remaining characters in given string.
- Otherwise, return “false” that is for not palindrome string.
Complete Executable code:
The complete code is implemented for checking the palindrome is shown below:
//Header file
#include <iostream>
#include <iomanip>
//For standard input and output
using namespace std;
/* Function definition for checkPalindromeRecursion function */
bool checkPalindromeRecursion(string str)
{
/* If length is "0" or "1", then return "true" */
if(str...

Want to see the full answer?
Check out a sample textbook solution
Chapter 14 Solutions
Problem Solving with C++ (10th Edition)
- Recursive Palindrome! Recall that a palindrome is a string that reads the same forward and backward. Write a recursive function is_palindrome (s:str) -> bool to check whether a string s is a palindrome. Here's a hint: think about how you can use is_palindrome(t), where t is a substring of s, to help you decide whether s is a palindrome. Your Answer: 1 # Put your answer here 2 Submitarrow_forwardWrite a function that outputs a string passed into the function in reverse. Use recursion to achieve this.arrow_forwardWrite a recursive function to print all the permutations of a string. For example, for the string abc, the printout is:abcacbbacbcacabcba(Hint: Define the following two functions. The second function is a helper function.def displayPermuation(s):def displayPermuationHelper(s1, s2): The first function simply invokes displayPermuation(" ", s). The secondfunction uses a loop to move a character from s2 to s1 and recursively invokes t with a new s1 and s2. The base case is that s2 is empty and prints s1 to the console.)Write a test program that prompts the user to enter a string and displays all its permutations.arrow_forward
- In Kotlin, Write a higher-order function that takes two arguments and returns a String. The first argument is a String and the second is a function that takes a Char and returns a Boolean. Your function should return a String containing those of the characters in the original String for which the function returns true. Use a tail-recursive helper function. Remember to make sure you get the tail recursion right by using the tailrec annotation. Test the functionarrow_forwardWrite a recursive function, vowels, that returns the number of vowels in a string. Also, write a program to test your function.arrow_forwardIn Kotlin, Write and test a recursive function called harm with an expression body that takes an Int argument n and calculates the sum of 1/1 ... 1/n. For example, harm(3) should return about 1.833333333. You do not need to worry about integer division.arrow_forward
- Write a program that asks the user to enter a binary string and you must use this binary string as an input to your functions. These functions that are to be created will be recursive functions that return the decimal number equivalent of your binary string input. For this exercise, you will develop 2 different ways of solving this problem: • Implementation 1: A recursive function with two inputs: a binary string, and a number. (Hint: You can think of this number as the index that allows you to access each element in your sequence.) • Implementation 2: A recursive function with only one input: the binary string. Conditions: Do implementation 1 if the binary string starts with a '0'. Otherwise, do implementation 2 if the binary string starts with '1'. Before you return a value in your recursive function's base case, print a statement that reveals the identity of the function that is currently being used.arrow_forwardOnly typing answers please.arrow_forwardWrite a recursive function that takes as a parameter a nonnegative integer and generates the following pattern of stars. If the nonnegative integer is 4, the pattern generated is as follows: **** *** ** * * ** *** **** Also, write a program that prompts the user to enter the number of lines in the pattern and uses the recursive function to generate the pattern. For example, specifying 4 as the number of lines generates the preceding pattern.arrow_forward
- A recursive function is a function defined in terms of itself via self-referential expressions. This means that the function will continue to call itself and repeat its behavior until some condition is met to return a result. a- Write a python recursive function prod that takes x as an argument, and returns the result where, result=1*1/2*1/3*….*1/n b- Include a screenshot that shows a python program that uses the above function and prints the rounded result to three decimal places after prompting the user to enter a number, x. Use x=3. N.B: The code should be included in your answer.arrow_forwardin kotlin Write two versions of a recursive palindrome check function. One version should have a block body, and the other should have an expression body. Review the lecture slide about the substring function first. Use this algorithm: if the length of the string is less than 2, return true else if the first and last characters are not equal (!=) return false else return the result of a recursive call with the substring from the second character (the one at index 1) to the second to last character. Use a main that uses a loop to test the palindrome function using this list of strings: val ss = listOf("", "A", "AA", "AB", "AAA", "ABA", "ABB", "AAAA", "AABA", "ABBA", "ABCBA", "ABCAB")arrow_forwardA palindrome is a sentence that contains the same sequence of letters reading it either forwards or backwards. A classic example is '1\.ble was I, ereI saw Elba." Write a recursive function that detects whether a string is apalindrome. The basic idea is to check that the first and last letters of thestring are the same letter; if they are, then the entire string is a palindromeif everything between those letters is a palindrome.There are a couple of special cases to check for. If either the first orlast character of the string is not a letter, you can check to see if the restof the string is a palindrome with that character removed. Also, when youcompare letters, make sure that you do it in a case-insensitive way.Use your function in a program that prompts a user for a phrase andthen tells whether or not it is a palindrome. Here's another classic fortesting: '1\. man, a plan, a canal, Panama!"arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
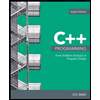