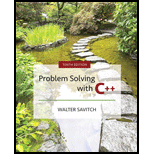
Problem Solving with C++ (10th Edition)
10th Edition
ISBN: 9780134448282
Author: Walter Savitch, Kenrick Mock
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 13.2, Problem 10STE
Program Plan Intro
Stack:
- A stack denotes a data structure that retrieves data in reverse of order in which data is stored.
- The top items present in the stack are to be removed first.
- It is called as a last in first out data structure.
- It could be used for keeping track of function calls in a program.
- The insertion as well as removal of elements takes place at same end.
- The upper part of stack where insertion and removal takes place is known as “top”.
- The part opposite to “top” is termed as “base” of stack.
- It provides an ordering based on length of time in collection.
- The newer items will be near top whereas older items are near base.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Refer to page 75 for graph-related problems.
Instructions:
• Implement a greedy graph coloring algorithm for the given graph.
• Demonstrate the steps to assign colors while minimizing the chromatic number.
•
Analyze the time complexity and limitations of the approach.
Link [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440 AZF/view?usp=sharing]
Refer to page 150 for problems on socket programming.
Instructions:
• Develop a client-server application using sockets to exchange messages.
•
Implement both TCP and UDP communication and highlight their differences.
• Test the program under different network conditions and analyze results.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]
Refer to page 80 for problems on white-box testing.
Instructions:
•
Perform control flow testing for the given program, drawing the control flow graph (CFG).
• Design test cases to achieve statement, branch, and path coverage.
• Justify the adequacy of your test cases using the CFG.
Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qo Hazb9tC440 AZF/view?usp=sharing]
Chapter 13 Solutions
Problem Solving with C++ (10th Edition)
Ch. 13.1 - Suppose your program contains the following type...Ch. 13.1 - Suppose that your program contains the type...Ch. 13.1 - Prob. 3STECh. 13.1 - Prob. 4STECh. 13.1 - Prob. 5STECh. 13.1 - Prob. 6STECh. 13.1 - Prob. 7STECh. 13.1 - Suppose your program contains type definitions and...Ch. 13.1 - Prob. 9STECh. 13.2 - Prob. 10STE
Ch. 13.2 - Prob. 11STECh. 13.2 - Prob. 12STECh. 13.2 - Prob. 13STECh. 13 - The following program creates a linked list with...Ch. 13 - Re-do Practice Program 1, but instead of a struct,...Ch. 13 - Write a void function that takes a linked list of...Ch. 13 - Write a function called mergeLists that takes two...Ch. 13 - In this project you will redo Programming Project...Ch. 13 - A harder version of Programming Project 4 would be...Ch. 13 - Prob. 6PPCh. 13 - Prob. 8PPCh. 13 - Prob. 9PPCh. 13 - Prob. 10PP
Knowledge Booster
Similar questions
- Refer to page 10 for problems on parsing. Instructions: • Design a top-down parser for the given grammar (e.g., recursive descent or LL(1)). • Compute the FIRST and FOLLOW sets and construct the parsing table if applicable. • Parse a sample input string and explain the derivation step-by-step. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qoHazb9tC440 AZF/view?usp=sharing]arrow_forwardRefer to page 20 for problems related to finite automata. Instructions: • Design a deterministic finite automaton (DFA) or nondeterministic finite automaton (NFA) for the given language. • Minimize the DFA and show all steps, including state merging. • Verify that the automaton accepts the correct language by testing with sample strings. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forwardRefer to page 60 for solving the Knapsack problem using dynamic programming. Instructions: • Implement the dynamic programming approach for the 0/1 Knapsack problem. Clearly define the recurrence relation and show the construction of the DP table. Verify your solution by tracing the selected items for a given weight limit. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS3IZ9qoHazb9tC440AZF/view?usp=sharing]arrow_forward
- Refer to page 70 for problems related to process synchronization. Instructions: • • Solve a synchronization problem using semaphores or monitors (e.g., Producer-Consumer, Readers-Writers). Write pseudocode for the solution and explain the critical section management. • Ensure the solution avoids deadlock and starvation. Test with an example scenario. Link: [https://drive.google.com/file/d/1wKSrun-GlxirS31Z9qo Hazb9tC440AZF/view?usp=sharing]arrow_forward15 points Save ARS Consider the following scenario in which host 10.0.0.1 is communicating with an external SMTP mail server at IP address 128.119.40.186. NAT translation table WAN side addr LAN side addr (c), 5051 (d), 3031 S: (e),5051 SMTP B D (f.(g) 10.0.0.4 server 138.76.29.7 128.119.40.186 (a) is the source IP address at A, and its value. S: (a),3031 D: (b), 25 10.0.0.1 A 10.0.0.2. 1. 138.76.29.7 10.0.0.3arrow_forward6.3A-3. Multiple Access protocols (3). Consider the figure below, which shows the arrival of 6 messages for transmission at different multiple access wireless nodes at times t=0.1, 1.4, 1.8, 3.2, 3.3, 4.1. Each transmission requires exactly one time unit. 1 t=0.0 2 3 45 t=1.0 t-2.0 t-3.0 6 t=4.0 t-5.0 For the CSMA protocol (without collision detection), indicate which packets are successfully transmitted. You should assume that it takes .2 time units for a signal to propagate from one node to each of the other nodes. You can assume that if a packet experiences a collision or senses the channel busy, then that node will not attempt a retransmission of that packet until sometime after t=5. Hint: consider propagation times carefully here. (Note: You can find more examples of problems similar to this here B.] ☐ U ப 5 - 3 1 4 6 2arrow_forward
- Just wanted to know, if you had a scene graph, how do you get multiple components from a specific scene node within a scene graph? Like if I wanted to get a component from wheel from the scene graph, does that require traversing still? Like if a physics component requires a transform component and these two component are part of the same scene node. How does the physics component knows how to get the scene object's transform it is attached to, this being in a scene graph?arrow_forwardHow to develop a C program that receives the message sent by the provided program and displays the name and email included in the message on the screen?Here is the code of the program that sends the message for reference: typedef struct { long tipo; struct { char nome[50]; char email[40]; } dados;} MsgStruct; int main() { int msg_id, status; msg_id = msgget(1000, 0600 | IPC_CREAT); exit_on_error(msg_id, "Creation/Connection"); MsgStruct msg; msg.tipo = 5; strcpy(msg.dados.nome, "Pedro Silva"); strcpy(msg.dados.email, "pedro@sapo.pt"); status = msgsnd(msg_id, &msg, sizeof(msg.dados), 0); exit_on_error(status, "Send"); printf("Message sent!\n");}arrow_forward9. Let L₁=L(ab*aa), L₂=L(a*bba*). Find a regular expression for (L₁ UL2)*L2. 10. Show that the language is not regular. L= {a":n≥1} 11. Show a derivation tree for the string aabbbb with the grammar S→ABλ, A→aB, B→Sb. Give a verbal description of the language generated by this grammar.arrow_forward
- 14. Show that the language L= {wna (w) < Nь (w) < Nc (w)} is not context free.arrow_forward7. What language is accepted by the following generalized transition graph? a+b a+b* a a+b+c a+b 8. Construct a right-linear grammar for the language L ((aaab*ab)*).arrow_forward5. Find an nfa with three states that accepts the language L = {a^ : n≥1} U {b³a* : m≥0, k≥0}. 6. Find a regular expression for L = {vwv: v, wЄ {a, b}*, |v|≤4}.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
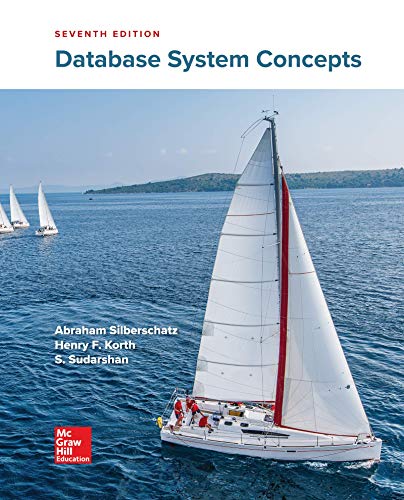
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
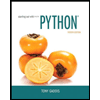
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
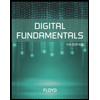
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
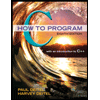
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
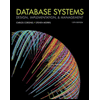
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
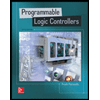
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education