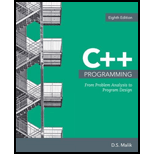
Explanation of Solution
The class dateType has a friend function declared in its definition. The friend function is then defined with two parameters of dateType and a bool return type. The logic of the function is explained with the in-lined comments in the program given below.
//class definition
class dateType
{
//declare the friend function in the class definition
friend bool before(dateType d1, dateType d2);
public:
void setDate(int month, int day, int year);
int getDay() const;
int getMonth() const;
int getYear() const;
void printDate() const;
bool isLeapYear(int) const;
dateType(int month = 1, int day = 1, int year = 1900);
private:
int dMonth; //variable to store the month
int dDay; //variable to store the day
int dYear; //variable to store the year
};
//define the friend function
bool before(dateType d1, dateType d2){
//if the year of first object is strictly less
//than the year of second object return true
//else if the year of the first object is
//strictly more than the year of the second
//object return false else if there is a tie
//then compare the months
if (d1...

Want to see the full answer?
Check out a sample textbook solution
Chapter 13 Solutions
C++ Programming: From Problem Analysis to Program Design
- Question P .Write the implementation (.cpp file) of the Acc2 class of the previous exercise. The full specification of the class is: An data member named sum of type integer. A constructor that accepts no parameters. THe constructor initializes the data member sum to 0. A function named getSum that accepts no parameters and returns an integer. getSum returns the value of sum . Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forward1. Short questions: a. What is the difference between having a function return type being "const dataType &" and it being "dataType&"? b. When is it necessary to make a function or a class a friend of another class? c. List all who can access: the private member of a class protected member of a class public member of a class d. What is difference between static data and a regular data of a class?arrow_forwardI have the basis of a program that works as an inventory, it reads the contents of a file and displays them and their quantity in three places, listed below are the things that I need to add to the program below. Provided is the current code. 1) Convert your structure into a class. For this exercise, you can leave the data as public (otherwise you would have to change the input and output functions). 2) Write a member function show_all that prints all the information for one record - name, cost, markup, and the three inventory numbers. 3) Add a user option S that lets the user see all the information for all the items in the inventory, using the show_all member function. Print a header so the user knows what each column means, and format the output to appear in columns. Hint: do a setwidth() before *each* cout. Pick widths that make sense for name, cost, markup, and the three inventory numbers. Contents of the file: Contents of Inventory.txt Red delicious apples1.00 25 6 8…arrow_forward
- All of the functions in the class A(n)_ are virtual functions. Let us know what you think by filling in the gaps.arrow_forwardWrite the definition of class StorageFile and a test main() function. Class StorageFile does not contain inline function definitions. I. Write the class declaration for StorageFile and declare (no definitions) the following members: 1. A private member named location of type string. 2. A public default constructor. 3. A public non-default constructor with one parameter of type string. 4. A destructor 5. An accessor (getter) for location. II. Define the following members ONLY. Note that these definitions are placed outside the class' declaration. 1. Default constructor to initialize location to "none" 2. Non-default constructor to initialize location using the constructor's parameter. 3. The accessor (getter) for location II. Define main() to perform the following steps: 1. Create an instance of type StorageFile using the default constructor 2. Create an instance of type StorageFile using the non-default constructor, use "new" for the parameter. 3. Extract and print the value of…arrow_forwardSuppose you are writing a class called Student that has two private members, std::string name and int perm. Write the function prototype (function declaration) only for a default constructor for Student. For full credit, include the semicolon that terminates the prototype, but do not write the body of the constructorarrow_forward
- Write the definitions of the functions to implement the operations for the class dayType as defined in Programming. Also, write a program to test various operations on this class.arrow_forwardWrite C++ program: In this program, we will create a class called Camel. Below, we will describe what will define a Camel. You will also create a main function in which you will create objects of type Camel to test the functionality of your new user-defined type. In the main, you will create enough objects of your new type(s) in order to adequately test their functions. It is up to you to do this properly. Your Camel class is to contain the following:Member variables:• a float for weight• a short for age (months)• a string for the name• a char for gender• a bool to indicate whether alive (or not) Member functions:• a default constructor that creates a dead camel• a constructor that you can pass values to so as to establish its gender, weight, age, and name; it will default to alive.• a print function that will output to the screen the attributes of that camel in a nice, easy-to-read format.• an age_me function that returns nothing but increments the object's age.• an eat function that…arrow_forwardA(n)_ is a class that only has pure virtual functions. Fill in the blanks with your response.arrow_forward
- A friend function has access to class? all class members, is public members only, is not protected members only, is not private members, is not and a member of thearrow_forwardWrite a program in java a class Box having three private data members (width, depth, height) The class has three constructors which are having no parameter – for setting values to zero or null. having three parameters for assigning values to height, width, depth respectively. Overload the above constructor and use this keyword to set the values of width, height & depth. Provide getters/setters for data members. Write a function calculateVolume() which calculates the volume of the box. Write test Application that demonstrates the Box class by calling all the three constructors and method, creating a Create Box object, and then displaying the Box’s width , height, length and volumearrow_forwardIN C++, create a class called Employee that includes three pieces of information as data members—a first name (type string), a last name (type string) and a monthly salary (type int). Your class should have a constructor that initializes the three data members. Provide a set and a get function for each data member. If the monthly salary is not positive, set it to 0. Write a test program that demonstrates class Employee’s capabilities. Create two Employee objects and display each object’s yearly salary. Then give each Employee a 10 percent raise and display each Employee’s yearly salary again.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
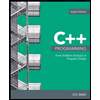