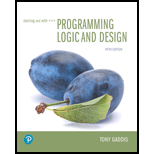
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
5th Edition
ISBN: 9780134801155
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 13, Problem 5PE
Program Plan Intro
Recursive Power Method
Program Plan:
- Global variable declaration:
- Initialize the variable “minimum” as “1”.
- Initialize the variable “maximum” as “100”.
- Define the “main()” function:
- Initialize the variable “number” is “0”.
- Initialize the variable “exp” is “0”.
- Get the input from the user and store it to the variable “number”.
- Check the value of “exp”
- If it is less than “minimum” or greater than “maximum”, then get the exponent “exp” from user.
- Call the function “recursivePower()” and pass the two arguments “number” and “exp”.
- Display the result on the output screen.
- Define the “recursivePower(x, y)” function:
- Check the value of “y”
- If it is equal to “0”, then returns “1”.
- Otherwise, call the function “recursivePower()” recursively along with the arguments “x” and the decremented value of “y”.
- Display the result on the output screen.
- Check the value of “y”
- Call the “main()” function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
7. Recursive Power Method
In Python, design a function that uses recursion to raise a number to a power. The function should accept two arguments: the number to be raised, and the exponent. Assume the exponent is a nonnegative integer.
Recursive Multiplication
Design a recursive function that accepts two arguments into the parameters x and y. The function should return the value of x times y. Remember, multiplication can be performed as repeated addition as follows:
7×4=4+4+4+4+4+4+4(To keep the function simple, assume that x and y will always hold positive nonzero integers.)
Recursive Power FunctionWrite a function that uses recursion to raise a number to a power. The function should accept two arguments: the number to be raised and the exponent. Assume that the exponent is a nonnegative integer. Demonstrate the function in a program.
SAMPLE RUN #0: ./recursiveExponent
Hide Invisibles
Highlight: Show Highlighted Only
2^3=8↵ 2^4=16↵ 3^3=27↵ 6^3=216↵ 7^7=823543↵ 10^9=1000000000↵
Chapter 13 Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Ch. 13.2 - It is said that a recursive algorithm has more...Ch. 13.2 - Prob. 13.2CPCh. 13.2 - What is a recursive case?Ch. 13.2 - What causes a recursive algorithm to stop calling...Ch. 13.2 - What is direct recursion? What is indirect...Ch. 13 - Prob. 1MCCh. 13 - A module is called once from a programs main...Ch. 13 - The part of a problem that can be solved without...Ch. 13 - Prob. 4MCCh. 13 - Prob. 5MC
Ch. 13 - Prob. 6MCCh. 13 - Any problem that can be solved recursively can...Ch. 13 - Actions taken by the computer when a module is...Ch. 13 - A recursive algorithm must _______ in the...Ch. 13 - A recursive algorithm must _____ in the base case....Ch. 13 - An algorithm that uses a loop will usually run...Ch. 13 - Some problems can be solved through recursion...Ch. 13 - It is not necessary to have a base case in all...Ch. 13 - In the base case, a recursive method calls itself...Ch. 13 - In Program 13-2, presented earlier in this...Ch. 13 - In this chapter, the rules given for calculating...Ch. 13 - Is recursion ever required to solve a problem?...Ch. 13 - When recursion is used to solve a problem, why...Ch. 13 - How is a problem usually reduced with a recursive...Ch. 13 - What will the following program display? Module...Ch. 13 - What will the following program display? Module...Ch. 13 - The following module uses a loop. Rewrite it as a...Ch. 13 - Prob. 1PECh. 13 - Prob. 2PECh. 13 - Recursive Array Sum Design a function that accepts...Ch. 13 - Prob. 4PECh. 13 - Prob. 5PECh. 13 - Ackermanns Function 7. Ackermanns Function is a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Recursive Multiplication Design a recursive function that accepts two arguments into the parameters x and y. The function should return the value of x times y. Remember, multiplication can be performed as repeated addition as follows: 7×4=4+4+4+4+4+4+4(To keep the function simple, assume that x and y will always hold positive nonzero integers.) IN Q BASIC LANGUAGEarrow_forwardRecursive PrintingDesign a recursive function that accepts an integer argument,n , and prints the numbers 1 up through n .arrow_forwardExponent y Catherine Arellano mplement a recursive function that returns he exponent given the base and the result. for example, if the base is 2 and the result is 3, then the output should be 3 because the exponent needed for 2 to become 8 is 3 (i.e. 23 = 8) nstructions: 1. In the code editor, you are provided with a main() function that asks the user for two integer inputs: 1. The first integer is the base 2. The second integer is the result 2. Furthermore, you are provided with the getExponent() function. The details of this function are the following: 1. Return type - int 2. Name - getExponent 3. Parameters 1. int - base 2. int - result 4. Description - this recursive function returns the exponent 5. Your task is to add the base case and the general case so it will work Score: 0/5 Overview 1080 main.c exponent.h 1 #include 2 #include "exponent.h" 3 int main(void) { 4 int base, result; 5 6 printf("Enter the base: "); scanf("%d", &base); 7 8 9 printf("Enter the result: ");…arrow_forward
- Recursive functions are ones that repeat themselves repeatedly.arrow_forward*C Language The greatest common divisor of integers x and y is the largest integer that divides both x and y. Write a recursive function GCD that returns the greatest common divisor of x and y. The GCD of x and y is defined as follows: If y is equal to zero, then GCD(x, y) is x; otherwise GCD(x, y) is GCD(y, x % y) where % is the remainder operator.arrow_forwardJAVA CODE PLEASE Recursive Functions Practice l by CodeChum Admin Create a recursive function named fun that prints the even numbers from 1 to 20 separated by a space in one line. In the main function, call the fun function. An initial code is provided for you. Just fill in the blanks. Output 2·4·6·8·10·12·14·16·18·20arrow_forward
- A recursive function must have a to end the recursion. recursive call base case O value boolean conditionarrow_forwardJAVA CODE PLEASE Recursive Functions Quiz by CodeChum Admin Create a recursive function named sequence that accepts an integer n. This function prints the first n numbers of the Fibonacci Sequence separated by a space in one line Fibonacci Sequence is a series of numbers in which each number is the sum of the two preceding numbers. In the main function, write a program that accepts an integer input. Call the sequence function by passing the inputted integer. Input 1. One line containing an integer Output Enter·a·number:·5 0·1·1·2·3arrow_forwardTopic: Recursive Function A bank increases the interest rate it gives to its customers by 1% every month the money stays in the bank. Write the recursive function that calculates the total amount of money at the end of the maturity date, based on the condition below. The parameters of the function: capital, initial interest rate and maturity date(months) Note: Codes should be written in C programming language.arrow_forward
- Recursive Power MethodWrite a method called powCal that uses recursion to raise a number to a power. The method should accept two arguments: The first argument is the exponent and the second argument is the number to be raised (example” powCal(10,2) means 210). Assume that the exponent is a nonnegative integer. Demonstrate the method in a program called Recursive (This means that you need to write a program that has at least two methods: main and powCal. The powCal method is where you implement the requirements above and the main method is where you make a method call to demonstrate how your powCal method work).arrow_forwardProblem Statement for Recursive Sum of Numbers Program Here is a simple recursive problem: Design a function that accepts a positive integer >= 1 and returns the sum of all the integers from 1 up to the number passed as an argument. For example, if 10 is passed as an argument, the function will return 55. Use recursion to calculate the sum. Write a second function which asks the user for the integer and displays the result of calling the function. Part 1. Understand the Problem To design a recursive function, you need to determine at least one base case (the base case returns a solution) and a general case (the general case calls the function again but passes a smaller version of the data as a parameter). To make this problem recursive think about it like this: If the integer is 1, the function will return 1. If the integer is 2, the function will return 1 + 2 = 3. If the integer is 3, the function will return 1 + 2 + 3 = 6. . . . For this problem, we will be sending in the "last"…arrow_forward3. Recursive Lines Write a recursive function that accepts an integer argument, n. The function should display n lines of asterisks on the screen, with the first line showing 1 asterisk, the second line showing 2 asterisks, up to the nth line which shows n asterisks.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
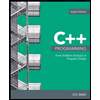
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning