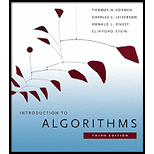
(a)
To explain the nodes that needs to change to insert a key k or delete a node y .
(a)

Explanation of Solution
For the insertion of the key k in the tree it first checks the ancestors of the tress then it traverses the children of that ancestor. The insertion causes some misbalancing situation so the tree needs to maintain the property of tree by changing the nodes.
The deletion of a node y is done by checking the children of the node y and the successor of the node y so that their children are placed into suitable node after deletion of the parent node y.
So, for the efficient execution of the operations it needs some changing in the ancestors of the node to accept the change by the insertion or deletion.
(b)
To gives an
(b)

Explanation of Solution
The algorithm for insertion in persistent tree is given below:
PERSISTENT-TREE-INSERT( T,k )
if
end if.
while
if
else
end if.
end while.
if
else
end if.
end.
The algorithm is used to insert a key k into the node. The algorithm checks the value of the nodes of the tree and then finds the suitable place to insert the key by comparing the value of key with the nodes of the tree.
The algorithm performs the insertion in such a way that it consider the root and start visiting the node of similar depth then it copy the nodes and then make new version of the tree with old and key k .
(c)
To explain the space required for the implementation of PERSISTENT-TREE-INSERT.
(c)

Explanation of Solution
The algorithm consists of the while loop that runs
The iterations of the algorithm run in constant time as they are just simple iteration or conditional iteration. For the purpose of storing all the h it needs some additional space equals to h .
Thus, the space and time required for the algorithm is depends upon the h and equals to
(d)
To prove that the PERSISTENT-TREE-INSERT would require
(d)

Explanation of Solution
The insertion of the a key into tree by using above algorithm is based on the fact that it first copy the original tree then it find the appropriate position for key and insert the key by addition key on the original tree.
The node that points to the appropriate position will copy and then it copy the ancestor of the node and so on until it copy all the connected nodes of the tree
The copying of a node takes constant time and one storage space for each node but there are total n nodes in the tree so it takes total time of n .
Thus, the algorithm takes total of
(e)
To explain the worse-case running time and space is
(e)

Explanation of Solution
The insertion or deletion of the key into tree cause dis-balancing in the tree so it needs to maintain the tree by changing the ancestors and children of the ancestors.
The algorithm allocates space of
For the finding the appropriate position it need to compare and check the values of node and for worse case it is equal to total nodes in
The operation is considering the ancestor of the node where the key is going to be inserted or deleted, the node with their children and ancestors are considered that is another subtree and for the worse case the height of that subtree is equal to the height of tree that is
Therefore, the total space and time requires for the operation is equals to
Want to see more full solutions like this?
Chapter 13 Solutions
Introduction to Algorithms
- Please help. How to solve this on Java language by using only Dynamic Programming? Thank you. We are given a set A of integers. Check whether we can partition A into three subsets with equal sums. It is important to note that all elements should be included in the equally divided subsets; no element should be left out, and none of the elements should be repeated in the subsets.For example: A={4,7,6,2,10,7,10,2} has such a partition: {4,2,10}, {7,7,2},{6,10}Input: A set, A, of integersOutput: if we can partition A into three subsets with equal sums, print subsets otherwise present a suitable message.arrow_forwardConsider a list L = {387, 690, 234, 435, 567, 123, 441}. Here, the number ofelements n = 7, the number of digits l = 3 and radix r = 10. This means that radixsort would require 10 bins and would complete the sorting in 3 passes. illustrates the passes of radix sort over the list. It is assumed that each key is thrown into the bin face down. At the end of each pass, when the keys are collected from each bin in order, the list of keys in each bin is turned upside down to be appended to the output listarrow_forwardFor a linked version of insertion sort, since there is no movement of data, thereis no need to start searching at the end of the sorted sublist. Instead, we shalltraverse the original list, taking one entry at a time and inserting it in the properposition in the sorted list. The pointer variable last_sorted will reference the end ofalgorithm the sorted part of the list, and last_sorted->next will reference the first entry thathas not yet been inserted into the sorted sublist. We shall let first_unsorted alsopoint to this entry and use a pointer current to search the sorted part of the list tofind where to insert *first_unsorted. If *first_unsorted belongs before the currenthead of the list, then we insert it there. Otherwise, we move current down thelist until first_unsorted->entry <= current->entry and then insert *first_unsortedbefore *current. To enable insertion before *current we keep a second pointertrailing in lock step one position closer to the head than current.…arrow_forward
- Create a set-based implementation of an abstract data type with the following operations. insert(S, x) Insert x into the set S. delete(S, x) Delete x fromthe set S. member(S, x) Return true if x ∈ S, false otherwise. position(S, x) Return the number of elements of S less than x. concatenate(S, T) Set S to the union of S and T, assuming every element in S is smaller than every element of T. All operations on sets with n elements must finish in O(log n) time.arrow_forwardTOKEN is a priority queue for organizing n data items with m priority numbers.TOKEN is implemented as a two-dimensional array TOKEN[1:m, 1:p], where p isthe maximum number of elements with a given priority. Execute the followingoperations on TOKEN [1:3, 1:2]. Here, INSERT(“xxx”, m) indicates the insertion ofitem “xxx” with priority number m, and DELETE() indicates the deletion of the firstamong the high priority items.i) INSERT(“not”, 1)ii) INSERT(“and”, 2)iii) INSERT(“or”, 2)iv) DELETE()v) INSERT (“equ”, 3)arrow_forwardWe will be looking at a problem known as the partition problem. The partition problem is to determineif a given set can be partitioned into two subsets such that the sum of elements in both subsets is thesame. This is a variant of the 0-1 knapsack problem.You would notice that the code only returns True or False. Suppose now you need to change thecode using Backtracking such that it returns the numbers of the subsets.Please explain your codes.# Returns true if there exists a sublist of list `nums[0…n)` with the given sum def subsetSum(nums, total): n = len(nums) # `T[i][j]` stores true if subset with sum `j` can be attained # using items up to first `i` items T = [[False for x in range(total + 1)] for y in range(n + 1)] # if the sum is zero for i in range(n + 1): T[i][0] = True # do for i'th item for i in range(1, n + 1): # consider all sum from 1 to total for j in range(1, total + 1): # don't include the…arrow_forward
- A set is a container that stores a collection of sorted unique elements. When we use a sorted array to implement Set ADT, the time complexity of find(x), insert(x) and remove(x) are all O(logn). Group of answer choices True Falsearrow_forwardImplement the three self-organizing list heuristics: Count – Whenever a record is accessed it may move toward the front of the list if its number of accesses becomes greater than the record(s) in front of it. Move-to-front – Whenever a record is accessed it is moved to the front of the list. This heuristic only works well with linked-lists; because, in arrays the cost of shifting all the records down one spot every time you move a record to the front is too expensive. Transpose – whenever the record is accessed swap it with the record immediately in front of it. Compare the cost of each heuristic by keeping track of the number of compares required when searching the list. Additional Instructions Use the SelfOrderedListADT abstract data type and the linked-list files I have provided to implement your self-ordered lists. You may incorporate the author’s linked list implementation via inheritance or composition, which ever makes the most sense to you (I will not evaluate that aspect of…arrow_forwardGiven an array that represents Breadth First Search or BFS traversal of a Complete Binary Search Tree, implement a recursive void method to print the preOrder traversal of the same Complete Binary Search Tree. You do not need to construct the BST. A Complete Binary Search Tree is a BST in which every level, except possibly the last, is completely filled, and all nodes are as far left as possible. Following is an example of a Complete Binary Search Tree: A. BFS traversal array (level by level from left to right): bfs[] = {50, 35, 55, 30, 45} B. PreOrder traversal (middle, left, right) : 50 35 30 45 55 Your recursive method "void convertBFStoPreOrder" will get an array A as an input and will print B. Hints: In the given BFS traversal array, bfs[], if i is the index of a parent, 2i+1 will give you the index of the left child, and 2i+2 will give you the index of the right child. "Assume that input array is always correct and represent a correct BFS traversal of a Complete BST."…arrow_forward
- Code in Java Using the binary search tree (BST) data structure, we can sort a sequence of n elements by first calling aninsertion procedure for n times to maintain a BST, and then performing an Inorder-Tree-Walk on theBST to output the elements in sorted order. In this programming assignment, you will implement in Java this sorting algorithm using BST, by usingthe improved insertion procedure. Note that each node of a BST is an object containing four attributes:key (for the value of an element), lef t (for its left child), right (for its right child), and p (for its parent).Please add a static counter to track the number of key comparisons made by your algorithm. Your programwill output the following1. The size of the input array,2. The input array,3. The list of array elements after sorting, and4. The number of key comparisons made.arrow_forwardGiven the following sets: U= {1,2,3,4,5,6,7,8,}, A={1,4,5,7}, B= {2,5,6,7,}, and C= {3,4,6,7} Complete the following set operations: a. A U(BUC) b. (A N (B N C))' . c. (A N B) U ( A N C) d. (A N B')U (A N C')arrow_forwardWrite an algorithm to implement the frequency count self-organizing list heuristic, assuming that the list is implemented using an array. In particular, write a function FreqCount that takes as input a value to be searched for and which adjusts the list appropriately. If the value is not already in the list, add it to the end of the list with a frequency count of one. Write an algorithm to implement the move-to-front self-organizing list heuristic, assuming that the list is implemented using an array. In particular, write a function MoveToFront that takes as input a value to be searched for and which adjusts the list appropriately. If the value is not already in the list, add it to the beginning of the list. Write an algorithm to implement the transpose self-organizing list heuristic, assuming that the list is implemented using an array. In particular, write a function Transpose that takes as input a value to be searched for and which adjusts the list appropriately. If the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
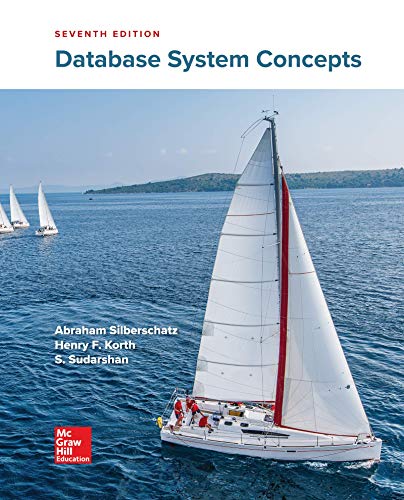
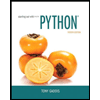
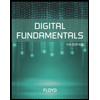
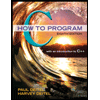
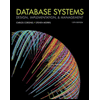
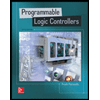