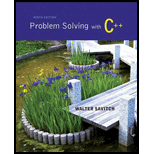
This Practice
File user.cpp:
namespace Authenticate
{
void inputUserName()
{
do {
cout << “Enter your username (8 letters only)” <<
endl;
cin >> username;
} while (!isValid());
}
string getUserName()
{
return username;
}
}
Define the username variable and the isValid() function in the unnamed namespace so the code will compile. The isValid() function should return true if username contains exactly eight letters. Generate an appropriate header file for this code.
Repeat the same steps for the file password.cpp, placing the password variable and the isValid() function in the unnamed namespace. In this case, the isValid() function should return true if the input password has at least eight characters including at least one nonletter:
File password.cpp:
namespace Authenticate
{
void inputPassword()
{
do {
cout << “Enter your password (at least 8 characters “
<<
“and at least one nonletter)” << endl;
cin >> password;
} while (!isValid());
}
string getPassword()
{
return password;
}
}
At this point you should have two functions named isValid(), each in different unnamed namespaces. Place the following main function in an appropriate place. The program should compile and run.
int main()
{
inputUserName();
inputPassword();
cout << “Your username is ” << getUserName() <<
“ and your password is: ” <<
getPassword() << endl;
return 0;
}
Test the program with several invalid usernames and passwords.

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Starting Out with Python (3rd Edition)
Starting out with Visual C# (4th Edition)
Concepts of Programming Languages (11th Edition)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
Concepts Of Programming Languages
Modern Database Management (12th Edition)
- Write the code in python Create an application that can create, display, search and maintain the book information for a bookstore. Requirements: 1) you need to use functions to write code for each menu item. 2) Information of books will be saved in a file, e.g,, books.txt. 3)User can add any number of books to the booklist. There can be 5 books or 500 books. In order for your program to handle it without any changes in the code, you cannot hardcode the number of books in your program.arrow_forwardpython function that creates and saves data in a file. the saved data represents exam grades. in the function , you will create n random numbers in the range 1-100, where n is the number of students. The function can be called as follows: createFile(filename, n) main function, in which the user inputs the file name and the number of students, then the main calls function createfile. the main should preform validation for n (should be > 0), and the filename(should end with .txt)arrow_forwardanswer in python Design and write a program that calculates and displays the number of minutes over the monthly contract minutes that a cell phone user incurred. The program should allow users to enter into a file minutes allowed in a month, minute used during a month and minutes over during a month for 12 months. The program reads the data from the file. The program validates the data as follows: - The minimum minutes allowed should be at least 200, but not greater than 800. Validate data accordingly. - The minutes used must be over 0. Validate data accordingly. Once correct data is read, the program should calculate the number of minutes over the minute allowed. If minutes were not over, print a message that they were not over the limit. If minutes were over, for every minute over, a .20 fee should be added to the monthly contract rate of 74.99. Be sure not to add the .20 fee for minutes 1 to the number of minutes allowed, but rather just minutes over. Display in a file the number…arrow_forward
- JAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY HOMEWORK ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #10. Word Counter (page 610) Write a program that asks the user for the name of a file. The program should display the number of words that the file contains. Input Validation: Make sure the file exists, before proceeding. Let the user type quit in order to exit the program. If the file is empty, the word count should be 0. input1.txt this is a test input2.txt thisisatest input3.txtEmpty input4txt this this is is a a test test Test Case 1 Please enter the file name or type QUIT to exit:\ninput1.txtENTERTotal number of words: 4\n Test Case 2 Please enter the file name or type QUIT to exit:\ninput2.txtENTERTotal number of words: 4\n Test Case 3 Please enter the file name or type QUIT to exit:\ninput3.txtENTERTotal number of words: 0\n…arrow_forwardC programming language Criteria graded: Declare file pointers Open file Read from file Write to file Close file Instructions: Write a segment of code that opens a file called “numbers.txt” for reading. This file is known to have 10 numbers in it. Read in each number and print a ‘*’ to the screen if the number is even. If the number is odd, do nothing. Conclude by closing this file.arrow_forwardFinish this program from the code posted below! Note: There should be two files Main.py and Contact.py You will implement the edit_contact function. In the function, do the following: Ask the user to enter the name of the contact they want to edit. If the contact exists, in a loop, give them the following choices Remove one of the phone numbers from that Contact. Add a phone number to that Contact. Change that Contact's email address. Change that Contact's name (if they do this, you will have to remove the key/value pair from the dictionary and re-add it, since the key is the contact’s name. Use the dictionary's pop method for this!) Stop editing the Contact Once the user is finished making changes to the Contact, the function should return. Code:from Contact import Contactimport pickledef load_contacts():""" Unpickle the data on mydata.dat and save it to a dictionaryReturn an empty dictionary if the file doesn't exist """try:with open("mydata.dat", 'rb') as file:return…arrow_forward
- Word List File Writer: Write a program that asks the user how many words they would like to write to a file, andthen asks the user to enter that many words, one at a time. The words should be writtento a file (in python)arrow_forwardC PROGRAMMING MOST IMPORTANT THING THE PASSWORD HAS TO BE READ FROM A TEXT FILE!!! I have a program that mimics the functions of an ATM. I need to create a program that checks the users passcode from a text file and if correct lets the user continue otherwise prints "Incorrect password" and then lets the user try the passcode again. So, there is a textfile named passcode.acc and the program reads the passcode from the first line of the textfile. The first line of the textfile is 1234 and this is the passcode that lets the user in. So to clarify I have other functions of the ATM done I just need to how to do the passcode checking section to the beginning.arrow_forwardC-Programming Write a program that tells you how many words in a text file are unique (meaning the word only appears once in the text file). List of words in text file:…arrow_forward
- File redirection allows you to redirect standard input (keyboard) and instead read from a file specified at the command prompt. It requires the use of the < operator (e.g. java MyProgram < input_file.txt) True or Falsearrow_forwardHere are the two programs which you may choose from to do this program: 1. Using Files - Student Line Up Modify the Student Line Up program described in Programming Challenge 14 which states: "A teacher has asked all of her students to line up according to their first name. For example, in one class Amy will at the front of the line, and Yolanda will be at the end. Write a program that prompts the user to enter the number of students in the class, then loops to read that many names. Once all of the names have been read, it reports which student would be at the front of the line and which one would be at the end of the line. You may assume that no two students have the same name. You are now to do this program reading names from a file. Names should be read in until there is no more data to be read. I am providing for you a file named LineUp.txt for you to use in writing this program. I suggest that you write it first without the file and then modify to add the file.arrow_forwardThis Python Lab 9 Lab: Write a file copying program. The program asks for the name of the file to copy from (source file) and the name of the file to copy to (destination file). The program opens the source file for reading and the destination file for writing. As the program reads each line from the source file and it writes the line to the destination file. When every line from the source file has been written to the destination file, it close both files and print “Copy is successful.” In the sample run, “add.py” is the source file and “add-copy.py” is the destination file. Note that both “add-copy.py” is identical to “add.py” because “add-copy.py” is a copy of “add.py”. Sample run: Enter file to copy from: add.py Enter file to copy to : add-copy.py Copy is successful. Source file: add.py print("This program adds two numbers") a = int(input("Enter first number: ")) b = int(input("Enter second number: ")) print(f"{a} + {b} = {a+b}") Destination file:…arrow_forward
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
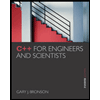
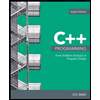
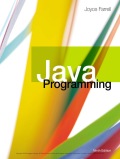