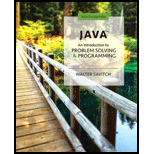
Java: An Introduction to Problem Solving and Programming (8th Edition)
8th Edition
ISBN: 9780134462035
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 12, Problem 10PP
Program Plan Intro
“GroupHolder” class
Program Plan:
- Import required package.
- Define “Main” class.
- Define “GroupHolder” class
- Create an array list “items” from “GroupHolder” class.
- Method definition for “findGroupNodeFor”
- Declare variable using “GroupNode” type.
- Set node “n” to “null”.
- Set “found” to “false”.
- Check condition using “while” loop
- Compute next node.
- If “n” equal to “s”, then set “found” to “true”.
- If found, then returns the value of “n”.
- Otherwise, returns null.
- Method definition of “addItem”.
- Call “findGroupNodeFor” method and store it to a variable “n”.
- If “n” is null, then add it to “items”.
- Method definition for “getRepresentative”.
- Call “findGroupNodeFor” method and store it to a variable “n”.
- Check condition using “while” loop.
- Set node to link value at node
- Finally returns the data at node.
- Set node to link value at node
- Method definition of “getAllRepresentatives”.
- Create an array list.
- Add data to “items”.
- Finally returns the result.
- Method definition of “inSameGroup”.
- Assign representative for string “s1” and store result to “r1”.
- Assign representative for string “s2” and store result to “r2”.
- Returns result.
- Method definition of “union”
- Assign representative for string “s1” and store result to “r1”.
- Assign representative for string “s2” and store result to “r2”.
- Check condition.
- Find group node for “r1” and “r2”.
- Create “GroupNode” class.
- Declare required variables.
- Create constructor for “GroupNode” class.
- Create parameterized for “GroupNode” class.
- Define main function.
- Create object “g” from “GroupHolder” class.
- Add item to “g” by calling the method “addItem”.
- Display groups by calling the method “getAllRepresentatives”.
- Join groups by calling the method “union”.
- Display groups after performing unions operations.
- Check “a” and “e” is in same group by calling method “inSameGroup”.
- Check “a” and “f” is in same group by calling method “inSameGroup”.
- Define “GroupHolder” class
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
For any element in keysList with a value greater than 100, print the corresponding value in itemsList, followed by a space. Ex: If keysList = {42, 105, 101, 100} and itemsList = {10, 20, 30, 40}, print: 20 30
Since keysList.at(1) and keysList.at(2) have values greater than 100, the value of itemsList.at(1) and itemsList.at(2) are printed.
#include <iostream>#include <vector>using namespace std;
int main() {const int SIZE_LIST = 4;vector<int> keysList(SIZE_LIST);vector<int> itemsList(SIZE_LIST);unsigned int i;
for (i = 0; i < keysList.size(); ++i) {cin >> keysList.at(i);}
for (i = 0; i < itemsList.size(); ++i) {cin >> itemsList.at(i);}
/* Your solution goes here */
cout << endl;
return 0;}
Please help me with this problem using c++.
How is a map different from other Java data structures?
A
Each data element connects to the next element in the map.
B
It's a data structure that stores data pairs: keys and their associated values.
C
Each data element can connect to multiple related elements in a map.
D
It's a data structure that keeps its data sorted.
Please solve this question in JAVA|
Write a Java program that asks the user to enter items
until the user chooses to stop, stores them into links
(nodes), and chains the links together into a linked
list. You will need to make the following changes:
• Your linked list should store information about
Customers' Transactions that contain three
fields: customer name (string), customer number
(int), and transaction description (string).
• Instead of putting all of your code inside the
main source code file, you should create the
following functions:
1. addToStart(Item x)
2. addToEnd(Item x)
3. insertAt(Item x, int n)
4. remove(int customer_number)
5. reverse)
6. printList)
Instead of simply asking the user if they want to
add a new link, your main program should offer
the user a menu of options:
1. Add a new link to the end
2. Add a new link to the beginning
3. Insert an element at index n into the list.
4. Remove a link from the list
5. Reverse the list
6. Print out the entire list
7. Quit…
Chapter 12 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Ch. 12.1 - Suppose aList is an object of the class...Ch. 12.1 - Prob. 2STQCh. 12.1 - Prob. 3STQCh. 12.1 - Prob. 4STQCh. 12.1 - Can you use the method add to insert an element at...Ch. 12.1 - Prob. 6STQCh. 12.1 - Prob. 7STQCh. 12.1 - If you create a list using the statement...Ch. 12.1 - Prob. 9STQCh. 12.1 - Prob. 11STQ
Ch. 12.1 - Prob. 12STQCh. 12.2 - Prob. 13STQCh. 12.2 - Prob. 14STQCh. 12.2 - Prob. 15STQCh. 12.2 - Prob. 16STQCh. 12.3 - Prob. 17STQCh. 12.3 - Prob. 18STQCh. 12.3 - Prob. 19STQCh. 12.3 - Write a definition of a method isEmpty for the...Ch. 12.3 - Prob. 21STQCh. 12.3 - Prob. 22STQCh. 12.3 - Prob. 23STQCh. 12.3 - Prob. 24STQCh. 12.3 - Redefine the method getDataAtCurrent in...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.3 - Repeat Question 25 for the method...Ch. 12.4 - Revise the definition of the class ListNode in...Ch. 12.4 - Prob. 30STQCh. 12.5 - What is the purpose of the FXML file?Ch. 12.5 - Prob. 32STQCh. 12 - Repeat Exercise 2 in Chapter 7, but use an...Ch. 12 - Prob. 2ECh. 12 - Prob. 3ECh. 12 - Repeat Exercises 6 and 7 in Chapter 7, but use an...Ch. 12 - Write a static method removeDuplicates...Ch. 12 - Write a static method...Ch. 12 - Write a program that will read sentences from a...Ch. 12 - Repeat Exercise 12 in Chapter 7, but use an...Ch. 12 - Write a program that will read a text file that...Ch. 12 - Revise the class StringLinkedList in Listing 12.5...Ch. 12 - Prob. 12ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 14ECh. 12 - Write some code that will use an iterator to...Ch. 12 - Prob. 17ECh. 12 - Revise the method selectionSort within the class...Ch. 12 - Repeat the previous practice program, but instead...Ch. 12 - Repeat Practice Program 1, but instead write a...Ch. 12 - Write a program that allows the user to enter an...Ch. 12 - Write a program that uses a HashMap to compute a...Ch. 12 - Write a program that creates Pet objects from data...Ch. 12 - Repeat the previous programming project, but sort...Ch. 12 - Repeat the previous programming project, but read...Ch. 12 - Prob. 9PPCh. 12 - Prob. 10PPCh. 12 - Prob. 11PPCh. 12 - Prob. 12PPCh. 12 - Prob. 13PPCh. 12 - Prob. 14PPCh. 12 - Prob. 15PP
Knowledge Booster
Similar questions
- For any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardIn Java, Explain when it might be preferable to use a map instead of a set.arrow_forwardA map is a container that stores a collection of ordered pairs, each pair consists of a key and a value, <key, value>. Keys must be unique. Values need not be unique, so several keys can map to the same values. The pairs in the map are sorted based on keys. Group of answer choices True Falsearrow_forward
- Tour.java Create a Tour data type that represents the sequence of points visited in a TSP tour. Represent the tour as a circular linked list of nodes, one for each point in the tour. Each Node contains two references: one to the associated Point and the other to the next Node in the tour. Each constructor must take constant time. All instance methods must take time linear (or better) in the number of points currently in the tour. To represent a node, within Tour.java, define a nested class Node: private class Node { private Point p; private Node next; } Your Tour data type must implement the following API. You must not add public methods to the API; however, you may add private instance variables or methods (which are only accessible in the class in which they are declared). public class Tour // Creates an empty tour. public Tour() // Creates the 4-point tour a→b→c→d→a (for debugging). public Tour(Point a, Point b, Point c, Point d) // Returns the number of points in this tour. public…arrow_forwardCreate Code in C++ For String searching algorithms – Boyer-Moore and Aho-Corasick – to find the occurrences of a particular string within a large body of text. For example, you might take a webserver log file and use an appropriate data structure to track how many times each page has been accessed.arrow_forwardA chain letter starts with a person sending a letter out to 10 others. Each person is asked to send the letter out to 10 others, and each letter contains a list of the previous six people in the chain. Unless there are fewer than six names in the list, each person sends one dollar to the first person in this list, removes the name of this person from the list, moves up each of the other five names one position, and inserts his or her name at the end of this list. If no person breaks the chain and no one receives more than one letter, how much money will a person in the chain ultimately receive? O 10,000 dollars O 1,000,000 dollars O 500,000 dollars O 100,000 dollarsarrow_forward
- Write a c++ program in which you have to Create a linklist which will take data from user for 5 students including their name, mobile#, age, and marks. After that display the data in Disp()function And check which student got the highest marks.arrow_forwardwrite a program for a library automation which gets the isbn number, name, author and publication year of the books in the library. the status will be filled by the program as follows: if publication year before 1985 the status is reference else status is available, the information about the books should be stored inside a linked list. the program should have a menu and the user inserts, displays, and deletes the elements from the menu by write a selecting options. the following data structure should be used. struct list char isbn[ 20 ]: char namei 20 ]. char authori 20 ]: int year; char status[200j: struct list "next, jinfo, wedoad n un posn ag pinogs nuu sulnogoj l press 1. to insert a book press 2. to display the book list press 3. to delete a book from listarrow_forwardSuppose we would like to create a data structure for holding numbers that canbe accessed either in the order that they were added or in sorted order. Weneed nodes having two references. If you follow one trail of references, you getthe items in the order they were added. If you follow the other trail of references, you get the items in numeric order. Create a class DualNode that wouldsupport such a data structure. Do not write the data structure itself.arrow_forward
- In this assignment, you will create a Linked List data structure variant called a “Circular Linked List”. The Node structure is the same as discussed in the slides and defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; } For the Circular Linked List, its class definition is as follows: public class CircularLinkedList { public int currentSize; public Node current; } In this Circular Linked List (CLL), each node has a reference to an existing next node. When Node elements are added to the CLL, the structure looks like a standard linked list with the last node’s next pointer always pointing to the first. In this way, there is no Node with a “next” pointer in the CLL that is ever pointing to null. For example, if a CLL has elements “5”, “3” and “4” and “current” is pointing to “3”, the CLL should look like: Key observations with this structure: The currentSize is 3 meaning there…arrow_forwardWAP c# program creates a string, s1, which deliberately leaves space for a name, much like you’d do with a letter you plan to run through a mail merge. We add two to the position where we find the comma to make sure there is a space between the comma and the name.arrow_forwardFor this project you will be implementing a new data structure called LinkedGrid. This is a linked type data structure version of a 2D array. The LinkedGrid can support any combination of dimensions (n x n, n x m, or m x n, where n > m) This data structure has a singly linked system which allows you to traverse the grid in multiple directions in addition to allowing you to retrieve specific rows and columns. The following diagram gives a rough visual of this data structure. NOTE: Your LinkedGrid MUST work with any number of nodes, the picture only shows twelve as an idea. You may not hardcode only twelve nodes... There should be no public functions other than the ones given to you You may implement any other private functions you feel are necessary to help you implement the given constructors / public functions Complete ALL methods with "TO DO" in LinkedGrid.java import java.util.ArrayList; public class LinkedGrid<E>{private int numRows;private int numCols; private…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
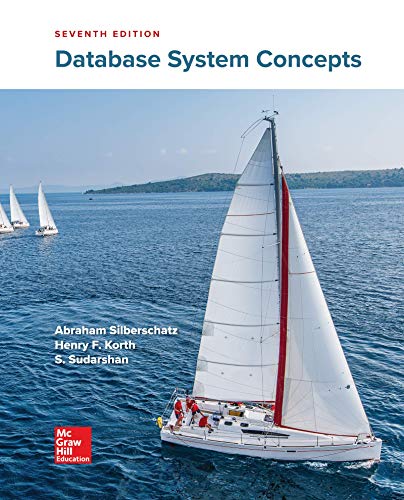
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
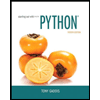
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
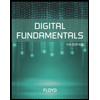
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
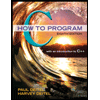
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
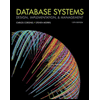
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
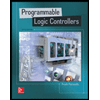
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education