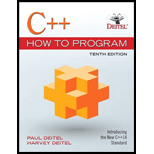
C++ How to Program (10th Edition)
10th Edition
ISBN: 9780134448237
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Game of Chess in C++
The objective of this assignment is to practice concepts related to inheritance, overriding, polymorphism, and abstract classes, to program the basics of a chess playing program.
About:
Chess is played on an 8 X 8 board where the initial placement of pieces. The white king is on e1, and the black king is on e8. Each chess piece can move in a specific way. Follow a simplified version. Most importantly, ignore an important rule in chess: Moving any piece in a way that puts your own king in check is illegal. Since we don't know what check means, for us a move is legal if the piece we are moving has an empty square to move to or can capture (replace) an opponent's piece (including their king).
The king does not move at all. Nor can it castle the queen and knight can't move either.
A pawn in the initial position may move one or two squares vertically forward to an empty square but cannot leap over any piece. Subsequently it can move only one square vertically forward…
Nutritional information (classes/constructors) PYTHON ONLY
Complete the FoodItem class by adding a constructor to initialize a food item. The constructor should initialize the name (a string) to "None" and all other instance attributes to 0.0 by default. If the constructor is called with a food name, grams of fat, grams of carbohydrates, and grams of protein, the constructor should assign each instance attribute with the appropriate parameter value.
The given program accepts as input a food item name, fat, carbs, and protein and the number of servings. The program creates a food item using the constructor parameters' default values and a food item using the input values. The program outputs the nutritional information and calories per serving for both food items.
Ex: If the input is:
M&M's10.034.02.01.0
where M&M's is the food name, 10.0 is the grams of fat, 34.0 is the grams of carbohydrates, 2.0 is the grams of protein, and 1.0 is the number of servings, the output is:…
SUBJECT: OOPPROGRAMMING LANGUAGE: C++
ALSO ADD SCREENSHOTS OF OUTPUT.
Write a class Distance to measure distance in meters and kilometers. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen.
Write another class Time to measure time in hours and minutes. The class should have appropriate constructors for initializing members to 0 as well as user provided values. The class should have display function to display the data members on screen.
Write another class which has appropriate functions for taking objects of the Distance class and Time class to store time and distance in a file.
Make the data members and functions in your program const where applicable
Chapter 11 Solutions
C++ How to Program (10th Edition)
Ch. 11 - Exercises11.3 (Composition as an Alternative to...Ch. 11 - (Inheritance Advantage) Discuss the ways in which...Ch. 11 - (Protected vs. Private Base Classes) Some...Ch. 11 - Prob. 11.6ECh. 11 - Prob. 11.7ECh. 11 - (Quadrilateral Inheritance Hierarchy) Draw an...Ch. 11 - Package Inheritance Hierarchy} Package-delivery...Ch. 11 - (Account Inheritance Hierarchy) Create an...
Knowledge Booster
Similar questions
- Task-1: Both computer and software engineering programs blend the broad engineering discipline courses with the computer science specific courses. Hence, it is a good idea to represent the "course" as an object before attempting to write a program to help students get register to the courses. The programmer can think of the abstraction of a course object as the representation of title, code, and credit values only. Write a class declaration for the course object that includes a constructor, a set and a get member function for each data member, and a member function that returns the course information upon request. For instance, for this course it should prepare a string like “COMP218 - Object-Oriented Programming". a) Use C++-strings for the representation of string of characters. b) Use C-strings for the representation of string of characters.arrow_forwardPROGRAMMING LANGUAGE :C++ QUESTION; You need to store hiring date and date of birth for Managers and Employees. You need to create a Date class for this purpose. Create objects of Date class in Manager and Employee classes to store respective dates. You need to write print function in Manager and Employee classes as well to print all information of Managers and Employees. You need to perform composition to implement this task. Create objects of Manager and Employee classes in main function and call print function for both objects. ______________________________________________________________ note : print the specific part of code in following ... Print Date class here: Print updated Manager class here: Print updated Employee class here: Print main function here:arrow_forwardInstructions-Java Assignment is to define a class named Address. The Address class will have three private instance variables: an int named street_number a String named street_name and a String named state. Write three constructors for the Address class: an empty constructor (no input parameters) that initializes the three instance variables with default values of your choice, a constructor that takes the street values as input but defaults the state to "Arizona", and a constructor that takes all three pieces of information as input Next create a driver class named Main.java. Put public static void main here and test out your class by creating three instances of Address, one using each of the constructors. You can choose the particular address values that are used. I recommend you make them up and do not use actual addresses. Run your code to make sure it works. Next add the following public methods to the Address class and test them from main as you go: Write getters and…arrow_forward
- Python Program: Auction Housearrow_forwardprogramming language :c++ make a program in c++ to store hiring date and date of birth for Managers and Employees. You need to create a Date class for this purpose. Create objects of Date class in Manager and Employee classes to store respective dates. You need to write print function in Manager and Employee classes as well to print all information of Managers and Employees. You need to perform composition to implement this task. Create objects of Manager and Employee classes in main function and call print function for both objects. Print Date class here. Print updated Manager class here. Print updated Employee class here. Print main function here.arrow_forward(Java) The Abstract Art Class Write an abstract class as follows: The class is named Art It inherits from the Comparable interface It has a private String member variable named name It has a private String member variable named artist It has a private int member variable called year It has a default constructor that assigns the values "No name" to name, "No artist" to artist and -1 to the year. This default constructor calls the three argument constructor. It has a three-argument constructor to assign values to the name, artist and year variables. It has a copy constructor that makes a copy of another non-null Art object It has getters and setters for the name, artist and year variables It has a toString() method that creates a string of artist, with name and year tabbed once on subsequent lines (see sample output) It has an equals method that compares this Art to another Object It has a compareTo method that compares in this order: 1) artist, 2) name, 3) year This class contains no…arrow_forward
- Object-Oriented Programming - Java programming.arrow_forward(java) The Painting Subclass Write class as follows: The class is named Painting, and it inherits from the Art class. It has a private String member variable named medium It has a default constructor that assigns the values "No name" to name, "No artist" to artist, -1 to year, and "No medium" to the medium variable. This default constructor calls the four-argument constructor. It has a four-argument constructor to assign values to the name, artist, year, and medium variables. It has a getter and settersfor the medium variable. It has a toString() method This class contains no other methods Make sure to include your name, the name of this class, our course number, and the Activity number in a Javadoc comment at the top. Make sure to write a Javadoc comment for each of these methods.arrow_forwardPythonarrow_forward
- c++ questionarrow_forwardProblem (Online Address Book): Using classes, design an online address book to keep track of the names, addresses, phone numbers, and dates of birth of family members, close friends, and certain business associates. Define a class addressType that can store a street address, city, state, and ZIP code. Use the appropriate functions to print and store the address. Also, use constructors to automatically initialize the member variables. Define a class extPersonType using the class personType with following members and methods: firstname, lastname, and accessors and mutators, print to display, and constructors. Define a class dateType for month, day and year as members with its accessors, mutators, and constructors) Design a class addressType. Add a member variable to this class to classify the person as a family member, friend, or business associate. Also, add a member variable to store the phone number. Add (or override) the functions to print and store the appropriate information.…arrow_forwardC++: OOP---Please comment the OOP concept. Thank you!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
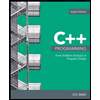
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning