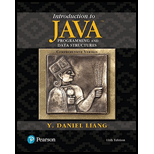
(Remove duplicates) Write a method that removes the duplicate elements from an array list of integers using the following header:
public static void removeDuplicate(ArrayList<Integer> list)
Write a test
Enter 10 integers: 34 5 3 5 6 4 33 2 2 4
The distinct integers are 34 5 3 6 4 33 2

Want to see the full answer?
Check out a sample textbook solution
Chapter 11 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Modern Database Management (12th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting out with Visual C# (4th Edition)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- . DO NOT REJECT THE QUESTION. ---- Write a Java program that asks the user to enter an array of integers in the main The program will ask the user for the number of integer elements to be put in the array, and then ask the user for each element of the array. The program then calls a method named isSorted() that accepts an array of integers and returns true if the list is in sorted (increasing) order and false otherwise. For example, if arrays named arr1 and arr2 store [10, 20, 30, 41, 56] and [2, 5, 3, 12, 10] respectively, the calls sorted(arr1) and isSorted(arr2) should return true and false respectively. Assume the array has at least one integer element. A one-element array is considered to be sorted.arrow_forwardnarmts Save Answer Write a Java program that uses ArrayList and Iterator. It should input from user the names and ages of your few friends in a loop and add into ArrayList. Finally, it should use Iterator to display the data in a proper format. (Hint- Lecture 02: Slide 8) Sample output: List of my Friends Enter name and age [friend# oj Khalid Al-shamri 22.5 Do you want to add another friend (y/n)? y Enter name and age [friend# 1] Rahsed Al-anazi 21.1 Do you want to add another friend (y/n)? y Enter name and age [friend# 2] Salem Al-mutairi 23.7 Do you want to add another friend (y/n)? n Here is the data you entered: 0. Khalid Al-shamri, 22.5 1. Rahsed Al-anazi, 21.1 2. Salem Al-mutairi, 23.7arrow_forwardMethod Details: public static void rotate(int[] array, boolean leftRotation, int positions) Rotates the provided array left if leftRotation is true; right otherwise. The number of positions to rotate is determined by positions. For example, rotating the array 10, 20, 7, 8 two positions to the left will update the array to 7, 8, 10, 20. Only arrays with 2 or more elements will be rotated. Hint: adding private methods that rotate an array one position to the left and one position to the right can help. Parameters: array - leftRotation - positions - Throws:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forward
- 2. (Average an array) Write two overloaded methods that return the average of an array with the following headers: public static int average (int[] array) public static double average (double[] array) Write a test program that prompts the user to enter ten double values, invokes this method, and displays the average value.arrow_forwardRotate Right k cells (use python) Consider an array named source. Write a method/function named rotateRight( source, k) that rotates all the elements of the source array to the right by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] rotateRight(source,3) After calling rotateRight(source,3), printing the array should give the output as: [ 40, 50, 60, 10, 20, 30]arrow_forwardShift Right k Cells (Use Python) Consider an array named source. Write a method/function named shifRight( source, k) that shifts all the elements of the source array to the right by 'k' positions. You must execute the method by passing an array and number of cells to be shifted. After calling the method, print the array to show whether the elements have been shifted properly. Example: source=[10,20,30,40,50,60] shiftRight(source,3) After calling shiftRight(source,3), printing the array should give the output as: [ 0,0,0,10,20,30 ]arrow_forward
- Exercise (Array and Method): Write a method called arrayToString(), which takes an int array and return a String in the form of {a1, a2, ..., an}. Take note that there is no comma after the last element. The method's signature is as follows: public static String arrayToString(int[] array) Also write a test driver to test this method (you should test on empty array, one-element array, and n-element array). Notes: This is similar to the built-in function Arrays.toString(). You could study its source code. Exercise (Array and Method): Write a boolean method called contains(), which takes an array of int and an int; and returns true if the array contains the given int. The method's signature is as follows: public static boolean contains(int[] array, int key) Also write a test driver to test this method. Exercise (Array and Method): Write a method called search(), which takes an array of int and an int; and returns the array index if the array contains the given int; or -1 otherwise. The…arrow_forward1. Code a method that will read in values from the user. 2. Add the following: Allow the user to enter any number of positive integers Store them in an array or array list Let the user enter -1 to end their list of values. Add error checking to make sure they enter positive values or a -1.arrow_forwardvoid mystery2(int list[], int length) { for (int i = 0; i < length - 1; i++) { if (i % 2 == 0) { list[i]++; } else { list[i]--; } }} For each array below, indicate what the array's contents would be after the function were called and passed that array as its parameter. use the following as input. {6, 3} {2, 4, 6} {1, 2, 3, 4} {2, 2, 2, 2, 2} {7, 3, 2, 0, 5, 1}arrow_forward
- 1.Answer the following questions: 1(a) Write a method to compute and return the value of maxEven and minOdd where maxEven is the largest even element of an int array and minOdd is the smallest odd element of the int array. The method is passed the int array and an int value that denotes the number of elements in the array. You may assume that the int array has at least 1 element in it. 1(b) Write a method to compute and return the value of countEven and countOdd where countEven is the number even elements in an int array and countOdd is the number of odd elements in the int array. The method is passed the int array and an int value that denotes the number of elements in the array. You may assume that the int array has at least 1 element in it.arrow_forward6. the grade is under 20 which is outlier, remove it from the array list. 7. Print array list using System.out.println() 8. Use indexOf to print index of 80. 9. Use get function. 10. What is the difference between get and index of? 11. Print the values of the array list using Iterator class. 12.. Delete all the values of the array list using clear function. 13. Print all the values of the array after you execute clear using System.out.println(). what is the result of using clear function? 14. What is the shortcoming of using array List?arrow_forward(python) 8. Create a new method called groupingEvens. This method will pass in 1 parameter and return only the even values in the list of values. This method will group together all of the even numbers in a list. INPUT: list of values OUTPUT: No output RETURN: any even numbers, as a list. *The list should be sorted*arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
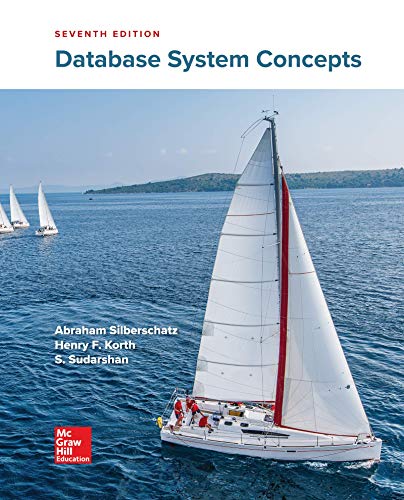
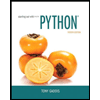
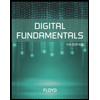
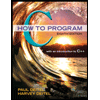
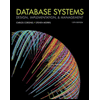
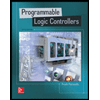