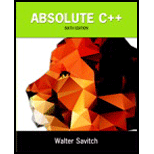
Concept explainers
Reread the code in Display 10.9. Then, write a class TwoD that implements the two-dimensional dynamic array of doubles using ideas from this display in its constructors. You should have a private member of type pointer to double to point to the dynamic array, and two int (or unsigned int) values that are MaxRows and MaxColS.
You should supply a default constructor for which you are to choose a default maximum row and column sizes and a parameterized constructor that allows the programmer to set maximum row and column sizes.
Further, you should provide a void member function that allows setting a particular row and column entry and a member function that returns a particular row and column entry as a value of type double.
Remark: It is difficult or impossible (depending on the details) to overload ( ) so it works as you would like for two-dimensional arrays. So simply use accessor and mutator functions using ordinary function notation.
Overload the + operator as a friend function to add two two-dimensional arrays. This function should return the TwoD object whose ith row, jth column element is the sum of the ith row, jth column element of the left-hand operand TWOD object and the ith row, jth column element of the right-hand operand TwoD object.
Provide a copy constructor, an overloaded operator z, and a destructor.
Declare class member functions that do not change the data as const members.

Program Plan:
- Define class named as TwoD and declare the variables for rows and columns.
- Declare a variable of pointer to double to point to the dynamic array.
- Define the default constructor and parameterized constructor to initialise rows and columns.
- Define the copy constructor that copies the values of one object array to another object array.
- Define operator + function declared as friend that add two dimensional arrays and return the sum.
- Use the setValue () function to set the values in the matrices m1 and m2 of double type.
- Finally write the main function to test the class TwoD.
Program Description:The purpose of the program is to set the values of two matrices of double type and add them to print the third matrix using the class TwoD and its constructors.
Explanation of Solution
Program:
//header files #include <iostream> usingnamespacestd; //Create Class TwoD classTwoD { //Private Data members private: intMaxRows; intMaxCols; //Declare array double&Twoarr; //Access Specifier public: //Create Default Constructor TwoD() { //chooses default rows and columns MaxRows=10; MaxCols=10; Twoarr=newdouble*[MaxRows]; for(int it =0; it <MaxRows;++it) { Twoarr[it]=newdouble[MaxCols]; } } //Create parameterised Constructor for setting the rows and columns TwoD(int rows,int cols) { //chooses Default rows and columns MaxRows= rows; MaxCols= cols; Twoarr=newdouble*[MaxRows]; for(int it =0; it <MaxRows;++it) { Twoarr[it]=newdouble[MaxCols]; } } //included the copy constructor TwoD(constTwoD&matrix) { //rows and columns assigned to the constructor object MaxRows=matrix.MaxRows; MaxCols=matrix.MaxCols; Twoarr=newdouble*[MaxRows]; for(int it =0; it <MaxRows;++it) { Twoarr[it]=newdouble[MaxCols]; for(intjt=0;jt<MaxCols;++jt) { Twoarr[it][jt]=matrix.Twoarr[it][jt]; } } } //Destructor ~TwoD() { for(int it =0; it <MaxRows;++it) { delete[]Twoarr[it]; } deleteTwoarr; } //member function for setting the values voidsetValue(int row,int col,doubleval) { Twoarr[row][col]=val; } //Friend function which overloads the "+" Operator friendTwoDoperator+(TwoD&m1,TwoD&m2) { TwoD*m3 =newTwoD(m1.MaxRows, m1.MaxCols); for(int it =0; it < m3->MaxRows;++it) { for(intjt=0;jt< m3->MaxCols;++jt) { //Performs addition m3->Twoarr[it][jt]= m1.Twoarr[it][jt]+ m2.Twoarr[it][jt]; } } return*m3; } //Void function to print the values void print() { for(int it =0; it <MaxRows;++it) { for(intjt=0;jt<MaxCols;++jt) { cout<<Twoarr[it][jt]<<""; } cout<<endl; } } }; intmain() { //declare variables intmaxRows; intmaxColumns; //Getting inputs from the user cout<<"Enter row Dimensions of the array:"<<endl; cin>>maxRows; cout<<"Enter Column Dimensions of the array:"<<endl; cin>>maxColumns; cout<<"&********************************"**lt;<endl; cout<<"Echoing the two-dimensional Array"<<endl; cout<<"&********************************"**lt;<endl; //passing parameters to the class objects TwoDm1(maxRows,maxColumns); TwoDm2(maxRows,maxColumns); for(int it =0; it <maxRows;++it) { for(intjt=0;jt<maxColumns;++jt) { m1.setValue(it,jt,0.2*(it +jt)); m2.setValue(it,jt,0.3*(it -jt)); } } //printing the results cout<<"&***********"**lt;<endl; cout<<"Matrix 1 "<<endl; cout<<"&***********"**lt;<endl; m1.print(); cout<<"&***********"**lt;<endl; cout<<"Matrix 2"<<endl; cout<<"&***********"**lt;<endl; m2.print(); //performs the addition operation TwoD m3 = m1 + m2; cout<<"&***********"**lt;<endl; cout<<"Addition "<<endl; cout<<"&***********"**lt;<endl; m3.print(); return0; }
Explanation:
First, TwoD class is defined along with all the required variables and constructors. Then, from the main ()function, the instances of the class is called, the rows of the array and the column of the array are entered by the user.
The two dimensional array is echoed randomly. Two matrices of given order are generated. The sum of these two matrices are obtained and displayed on the output screen.
Output Screenshot:
Want to see more full solutions like this?
Chapter 10 Solutions
Absolute C++
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (4th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Problem Solving with C++ (10th Edition)
Starting Out with Python (4th Edition)
Java How To Program (Early Objects)
- Write a program that will sort a prmiitive array of data using the following guidelines - DO NOT USE VECTORS, COLLECTIONS, SETS or any other data structures from your programming language. The codes for both the money and currency should stay the same, the main focus should be on the main file code (Programming language Java) Create a helper function called 'RecurInsSort' such that: It is a standalone function not part of any class from the prior lab or any new class you feel like creating here, Takes in the same type of parameters as any standard Insertion Sort with recursion behavior, i.e. void RecurInsSort(Currency arr[], int size) Prints out how the array looks every time a recursive step returns back to its caller The objects in the array should be Money objects added or manipulated using Currency references/pointers. It is OK to print out the array partially when returning from a particular step as long as the process of sorting is clearly demonstrated in the output. In…arrow_forwardA) Consider the generic print() method, write a method that takes an array of E, where E must be Comparable or extend a type that is Comparable, and returns a reference to the greatest object in the array. In this case, the greatest value is one for which compareTo() would return 0 or more for any other object in the array. If there is more than one possible largest value (these would have to be equal to each other but greater than any other objects in the array), it does not matter which one you choose. B) Write a generic Java queue class (a plain queue, not a priority queue). Call it GenericQueue, because the JDK already has an interface called Queue. The class must be able to create a queue of objects of any reference type. Consider the GenericStack class shown in the lecture notes for some hints. Like the Stack, the GenericQueue should use an underlying ArrayList<E>. Write these methods and any others you find useful: enqueue() adds an E to the queue peek() returns a…arrow_forwardWrite a program that uses two classes. The first class is called “dAta” and holds x and y coordinates of a point in 2-d space called p1. The second class is called “cOmpute” and holds an array of two pointers to the “dAta” class. The “cOmpute” class has a function, lOad(float x, float y, int n), which loads x and y coordinate data into the array at index n. It also has a function, sLope(), which computes the slope of the line connecting the two array data coordiantes. It also has a function, pRint(), which prints the slope result to the screen. Implement a divide by zero exception using throw in the sLope() nd/or pRint() function as appropriate. The exception should, when caught, print “Slope calculation error…” to the screen and exit the function. The program should load the array with some example points and print the slope to the screen using the class functions.arrow_forward
- develop a class productsales with static array 'sales' of size 12 where 0th index stores january.1st index stores sales in february....so on11th index stores sales in december. create a static findmaximumsalesmonth()which prints the month in which maximun sales are done and test this above fuction main() from the same classarrow_forwardDefine a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): vector<StatePair <int, string>> zipCodeState: ZIP code - state abbreviation pairs vector<StatePair<string, string>> abbrevState: state abbreviation - state name pairs vector<StatePair<string, int>> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 #include<iostream>#include <fstream>#include <vector>#include <string>#include "StatePair.h"using namespace…arrow_forwardDefine a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): vector<StatePair <int, string>> zipCodeState: ZIP code - state abbreviation pairs vector<StatePair<string, string>> abbrevState: state abbreviation - state name pairs vector<StatePair<string, int>> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 #include<iostream>#include <fstream>#include <vector>#include <string>#include "StatePair.h"using namespace…arrow_forward
- Define a class StatePair with two template types (T1 and T2), constructors, mutators, accessors, and a PrintInfo() method. Three vectors have been pre-filled with StatePair data in main(): vector<StatePair <int, string>> zipCodeState: ZIP code - state abbreviation pairs vector<StatePair<string, string>> abbrevState: state abbreviation - state name pairs vector<StatePair<string, int>> statePopulation: state name - population pairs Complete main() to use an input ZIP code to retrieve the correct state abbreviation from the vector zipCodeState. Then use the state abbreviation to retrieve the state name from the vector abbrevState. Lastly, use the state name to retrieve the correct state name/population pair from the vector statePopulation and output the pair. Ex: If the input is: 21044 the output is: Maryland: 6079602 -main.cpp: #include<iostream> #include <fstream> #include <vector> #include <string> #include "StatePair.h"…arrow_forwardImplement the function below. void swap(int pos1, int pos2){} Initial code to be completed: class ArrayList : public List { int* array; int index; int capacity; void dyn_all_add(){ int cap = ceil(capacity * 1.5); array = (int*)realloc(array,cap * sizeof(int)); capacity = cap; } void dyn_all_rem(){ int cap = capacity - (capacity/3); array = (int*)realloc(array,cap * sizeof(int)); capacity = cap; } public: // CONSTRUCTOR ArrayList() { capacity = 4; array = (int*)malloc(capacity); index = 0; } int add(int num) { if (index == capacity){ dyn_all_add(); } *(array + index) = num; index++; return index; } int get(int pos){ if (pos-1 < index){ return *(array + pos-1); } return -1; } int size(){ return index; }…arrow_forwardQ2: Write aprogram in CT' using OOP to create a class (A) , that contain the iöllowingIUnction:— (drawl) to drawa line Of Stars (Then create a class (B) , with public visibility mode inherit from class (A) ,contain the following function:(draw2) to draw the shape bellow using the tilnction (drawl),arrow_forward
- Write a program to create array of objects for the same to access these. Also, make use of member functions to accept values and print the name, age, designation and salary.arrow_forwardSuppose we implement the += operator as shown in the following. What goes wrong with b += b ? void bag::operator +=(const bag& addend) // Library facilities used: cassert{ size_type i; // An array index assert(size( ) + addend.size( ) <= CAPACITY); for (i = 0; i < addend.used; ++i) { data[used] = addend.data[i]; ++used; }} Group of answer choices If we activate b += b, then the private member variable size is the same variable as addend.size. The size( ) + addend.size( ) is less than CAPACITY. So the program after the assert statement will be executed. If we activate b += b, then the private member variable size is the same variable as addend.size. The size( ) + addend.size( ) is larger than CAPACITY. So the program after the assert statement will not have chance to be executed. If we activate b += b, then the private member variable used is the same variable as addend.used. Each iteration of the loop adds 1 to used, and hence…arrow_forwardDesign and implement a class called RandomArray, which has an integer array. The constructor receives the size of the array to be allocated, then populates the array with random numbers from the range 0 through the size of the array. Methods are required that return the minimum value, maximum value, average value, and a String representation of the array values. Document your design with a UML Class diagram. Create a separate driver class that prompts the user for the sample size, then instantiates a RandomArray object of that size and outputs its contents and the minimum, maximum, and average values.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
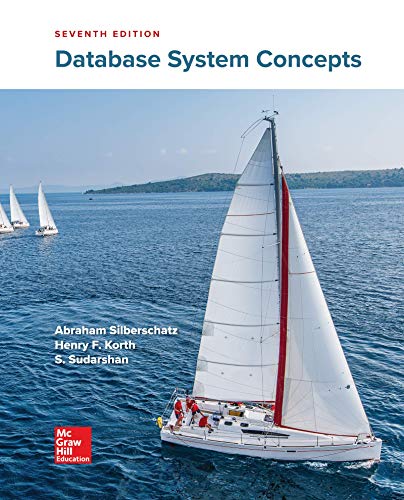
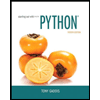
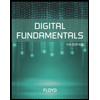
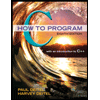
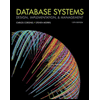
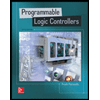