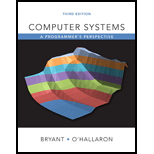
Explanation of Solution
Modified code from figure 10.5:
The modified code for “cpfile.c” is shown below:
//Include header file
#include <stdio.h>
#include "csapp.h"
//Main function
int main(int argc, char* argv[])
{
//Declare "int" variable
int n;
//Create the object for read buffer of type
rio_t rio;
//Declare "MAXLINE" for memory
char buf[MAXLINE];
/* Check the command line arguments. If the command line contains two argument, then copy the infile to standard output */
if (argc == 2)
{
/* Store the descriptor number in "fd" */
int fd = Open(argv[1], O_RDONLY, 0);
while ((n = Rio_readn(fd, buf, MAXBUF)) != 0)
Rio_writen(STDOUT_FILENO, buf, n);
//Exit the process
exit(0);
}
/* Otherwise copy a text file from standard input to...

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Computer Systems: A Programmer's Perspective (3rd Edition)
- A criticism of the break and continue statements is that each is unstructured. These statements can always be replaced by structured statements. Describe in general how you’d remove any break statement from a loop in a program and replace it with some structured equivalent. [Hint: The break statement leaves a loop from within the body ofthe loop. Another way to leave is by failing the loop-continuation test. Consider using in theloop-continuation test a second test that indicates “early exit because of a ‘break’ condition.”] Use the technique you developed here to remove the break statement from the program (c++ language)arrow_forwardA criticism of the break and continue statements is that each is unstructured. These statements can always be replaced by structured statements. Describe in general how you’d remove any break statement from a loop in a program and replace it with some structured equivalent. [Hint: The break statement leaves a loop from within the body ofthe loop. Another way to leave is by failing the loop-continuation test. Consider using in theloop-continuation test a second test that indicates “early exit because of a ‘break’ condition.”]arrow_forwardA loc-cs.org/~chu/DataStructures/H 19 Special Hint for Taking User's Input import java.util.Scanner; /** * This is an example to show you that * the method nextInt() only flushes the number to the variable * and leave the "END OF LINE" symbol in the input buffer. * If you have next input looking for "END OF LINE", the system will not wait for user's input and just take the "END OF LINE" symbol from input buffer. * So, the solution is flush "END OF LINE" out before you 大 * expect an input from user. * @author Valerie Chu * @version August 24, 2020 */ public class Example { public static void main(String[] args) { Scanner input = new Scanner (System.in); String [] item = new String[2]; int (] num = new int[2]; for (int i=0; i<2; i++) { System.out.println("Which item are you shopping for?"); item[i] = input.nextLine(); System.out.printf("How many \"%s\" do you need?\n", item[i]); num[i] = input.nextInt(); input.nextLine(); //Flush the "END OF LINE" symbol out…arrow_forward
- Attach output with code compilation else dislike 234D5.arrow_forwardModify the code to print the last occurrences of x ?arrow_forwardWrite a Matlab program in a script file that finds and displays all the numbers between 700 and 2999, whose product of digits is 6 times the sum of the digits (e.g. 2864 since 2*8*6*4 = 6(2+8+6+4)). You may use a loop in the script. For extra credit, print out also the number of times that the product and sum were the same! (5 pts)arrow_forward
- Encrypt the message 001100001010 using Simplified DES (as defined in the book in section 4.2) and key 111000101. [Hint: After one round, the output is 001010010011.] If you do this by hand, it is sufficient to only do 3 rounds instead of 8 rounds. If you write a program, you should carry out the full implementation. Please provide your code.arrow_forwardWrite a program that converts an ASCII text string from memory into all lowercase and stores the output to memory. The input string can contain uppercase and lowercase letters as well as spaces, punctuation (period, comma, question mark, exclamation point), and decimal digits. The input string will be null-terminated (that is, end with a 0 byte) and the output string is also to be null-terminated. (Observe: a 0 byte is different than the ASCII code for decimal digit 0 , which is 0x30.) Example: Convert the string "IN 2023, faLL stARTs on September 23." to "in 2023, fall starts on september 23.".arrow_forwardWhat is the correct output of the following example?arrow_forward
- In this assignment, you will create a Java program to search recursively for files in a directory.• The program must take two command line parameters. First parameter is the folder to search for. Thesecond parameter is the filename to look for, which may only be a partial name.• If incorrect number of parameters are given, your program should print an error message and show thecorrect format.• Your program must search recursively in the given directory for the files whose name contains the givenfilename. Once a match is found, your program should print the full path of the file, followed by a newline.• You can implement everything in the main class. You need define a recursive method such as:public static search(File sourceFolder, String filename)For each subfolder in sourceFolder, you recursively call the method to search.• A sample run of the program may look like this://The following command line example searches any file with “Assignment” in its name%java FuAssign7.FuAssignment7…arrow_forwardWrite a C++ program that will do this: [loop could conceivably go on forever, and should work for any legitimate grade values entered] SAMPLE run: What grade did you get on quiz 1 ? 95 Letter grade for quiz 1 is: A Are there any more quizs? y What grade did you get on quiz 2 ? 75 Letter grade for quiz 2 is: C Are there any more quiz? y What grade did you get on quiz 3 ? 85 Letter grade for quiz 3 is: B Are there any more quiz? n Your overall average is B (85). [program ends]arrow_forwardA program evaluates binary arithmetic expressions that are read from an input file. All of the operands are integers, and the only operators are +, -, *, and 7. In writing the program, the programmer forgot to include a test that checks whether the right-hand operand in a division expression equals zero. When will this oversight be detected by the computer? (A) At compile time (B) While editing the program (C) As soon as the data from the input file is read (D) During evaluation of the expressions (E) When at least one incorrect value for the expressions is outputarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
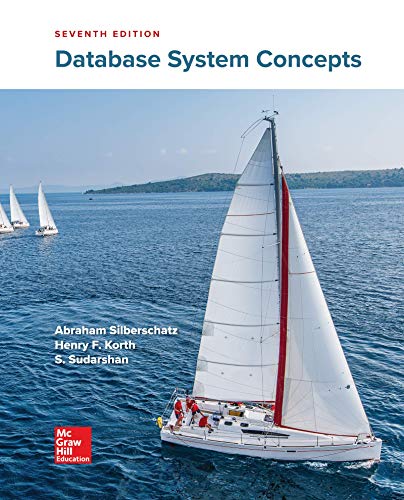
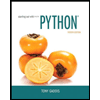
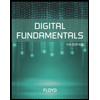
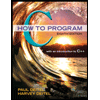
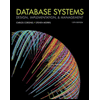
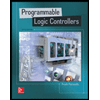