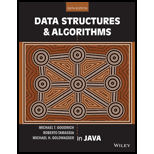
Data Structures and Algorithms in Java
6th Edition
ISBN: 9781118771334
Author: Michael T. Goodrich
Publisher: WILEY
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 1, Problem 23C
Write a short Java
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write a Java program to find continuous sub array of the given array whose sum is equal to a given number. For example, If {12, 5, 31, 9, 21, 8} is the given array and 45 is the given number, then you have to find continuous sub array in this array such that whose elements add up to 45. In this case, {5, 31, 9} is such sub array whose elements add up to 45.
Write a Java program that will accept an array of size 5 and accept whole numbers from console (as its arguments), print this array and find a minimum in it.
Write a java program to rearrange all the elements of an given array of integers so that all the odd numbers come before all the even numbers
Chapter 1 Solutions
Data Structures and Algorithms in Java
Ch. 1 - Prob. 1RCh. 1 - Suppose that we create an array A of GameEntry...Ch. 1 - Write a short Java method, isMultiple, that takes...Ch. 1 - Write a short Java method, isEven, that takes an...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that takes an integer n...Ch. 1 - Write a short Java method that counts the number...Ch. 1 - Prob. 9RCh. 1 - Prob. 10R
Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Prob. 12RCh. 1 - Modify the declaration of the first for loop in...Ch. 1 - Prob. 14CCh. 1 - Write a pseudocode description of a method for...Ch. 1 - Write a short program that takes as input three...Ch. 1 - Write a short Java method that takes an array of...Ch. 1 - Prob. 18CCh. 1 - Write a Java program that can take a positive...Ch. 1 - Write a Java method that takes an array of float...Ch. 1 - Write a Java method that takes an array containing...Ch. 1 - Prob. 22CCh. 1 - Write a short Java program that takes two arrays a...Ch. 1 - Modify the CreditCard class from Code Fragment 1.5...Ch. 1 - Modify the CreditCard class to add a toString()...Ch. 1 - Write a short Java program that takes all the...Ch. 1 - Write a Java program that can simulate a simple...Ch. 1 - A common punishment for school children is to...Ch. 1 - The birthday paradox says that the probability...Ch. 1 - (For those who know Java graphical user interface...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
What would be the output in Self-Test Exercise 15 if the first line were changed to the following? int firstCho...
Problem Solving with C++ (10th Edition)
Find the errors in the following code: 2. // Warning! This code contains an ERROR! if (average = 100) System.ou...
Starting Out with Java: Early Objects (6th Edition)
This standard library function returns a random floating-point number within a specified range of values. a. ra...
Starting Out with Python (3rd Edition)
In what year did the COBOL design process begin?
Concepts Of Programming Languages
(Pig Latin) Write a program that encodes English-language phrases into pig Latin. Pig Latin is a form of coded ...
C How to Program (8th Edition)
Describe two properties that each candidate key must satisfy.
Modern Database Management (12th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a java program to get the larger value between the first and last element of an array of an length 3 of integersarrow_forwardWrite a JAVA program that reads input an integer target and in output prints all elements greater than target from the array given below. Print -1 if all elements are less than the target value. int []Array = {67, 49, 11, 90, 123, 98, 8, 176, 34, 102};arrow_forwardWrite a java program to test if an array of integers contains an element 10 next to 10 or an element 20 next to 20, but not botharrow_forward
- Write a Java program to merge two given sorted array of integers and create a new sorted array.Input: N (size of array1), array1, M (size of array2), array2. 1 ≤ N, M ≤ 100.Output: New array of size N + M.arrow_forwardWrite a Java program to take input an integer n which is the size of an array and then take input n different integers which are the values of the array. Then check whether 1 appears in first or last position of the array. Given: n>=1arrow_forwardWrite a java program to swap the first and the last elements of the array without creating a new array. The array may have any length.arrow_forward
- Write a JAVA program to find the unique element which is present only once in the given array. All the remaining elements are repeated twice in the array. int []a = {2, 14, 6, 22, 2, 34, 22, 8, 6, 14, 8}; Use constant space and linear time.arrow_forwardWrite a java program to find all pairs of elements in an array whose sum is equal to given number.arrow_forwardWrite a Java method that takes the elements of a 2-dimensional array a (n * m), then itgives back the sum of the lines (separately) in an array b (n) and the product of thecolumns (separately) in an array c (m).arrow_forward
- FOR JAVA Write a Java method that takes two 2 dimensional int arrays (a and b) and a 2 dimensional boolean array (c) where all three arrays have the same size. Your method should return an array (result) such that, if a partic- ular element in c is true, then the corresponding (same indexed) element in result must be the multiplication of the the corresponding elements of a and b. If the element in c is false, then the the corresponding element in result must be the negative of multiplication of the the corresponding element in a and b. For example ifa = {{1,2,3},{4,5}},b = {{1,2,1},{0,2}} andc = {{true,false,true},{false,true}}, then the result should be result={{1, -4, 3}{0, 10}}.arrow_forwardWrite a Java program to implement a one-dimensional integer array of any size, add its elements and test whether the final sum is an odd or even. Sample array1: 25 3 80 9 25Sample output: EvenSample array2: 24 13 7 8 25Sample output: Oddarrow_forwardWrite a Java program to find all triplets equal to a given sum in an unsorted array of integersarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
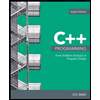
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License