Your task is to implement a function that, given an error tolerance err_tol (a floating-point value bet
Your task is to implement a function that, given an error tolerance err_tol (a floating-point value between 0 and 1) computes and prints the required input parameters for each
Requirements: Function signature (no return value). Be sure to import the Python modules you created for Parts 1, 2, and 3, e.g., import p1arch.
def pi_allpi(err_tol: float)
Notice that when you run your code repeatedly with the same err_tol input, you get the same values for Archimedes and Wallis (i.e., they are deterministic), but you keep getting different answers for Monte Carlo (non-deterministic). This is because Monte Carlo uses pseudo-random numbers for the darts' positions, and so gets different sets of points with every run.
To make sure you get the same result every time you run, you can initialize the pseudorandom number generator with a fixed seed first, before calling your p3mc.pi_mc function, e.g., random.random(10). This is a common technique used for testing non-deterministic computations.
Part 1
#p1arch
import math
def pi_arch(num_sides: int) -> float:
"""(int) -> float
>>>pi_arch(8)
3.0614674589207183
>>>pi_arch(16)
3.121445152258052
>>>pi_arch(100)
3.141075907812829
"""
inner_angle_b = 360.0 / num_sides
half_angle_a = inner_angle_b/ 2
one_half_side_s = math.sin(math.radians(half_angle_a))
side_s = one_half_side_s * 2
polygon_circumference= num_sides * side_s
pi = polygon_circumference / 2
return pi
Part 2
#p2 Wallis
def pi_wallis(num_pairs: int) ->float
"""(int) -> float
>>>wallis(100)
3.1337874906281575
>>>wallis(1000)
3.1308077460303785
>>>wallis(10000)
3.141514118681855
"""
acc = 1 # initialize accumulator to 1 for multiplication
num = 2 # numerator starts at 2
for a_pair in range(pairs):
left_term = num / (num - 1) # denominator is numerator - 1
right_term = num / (num + 1) # denominator is numerator + 1
acc = acc * left_term * right_term # compute running product
num = num + 2 # prepare for next term
pi = acc * 2
return pi
Part 3
#p3mc (monte carlo)
import random
from math import sqrt
def monte_pi(num_darts: int) -> float:
"""
>>>round(monte_pi(2000_000), 2)
3.14
"""
in_circle=0
for dart in range(num_darts):
x = random.random()
y= random. random()
if sqrt(x**2 + y**2) < 1.0:
in_circle +=1
pi=in_circle / num_darts * 4
return pi

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

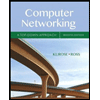
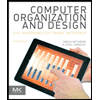
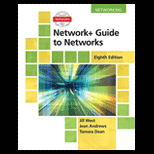
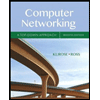
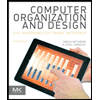
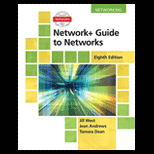
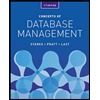
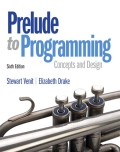
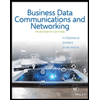