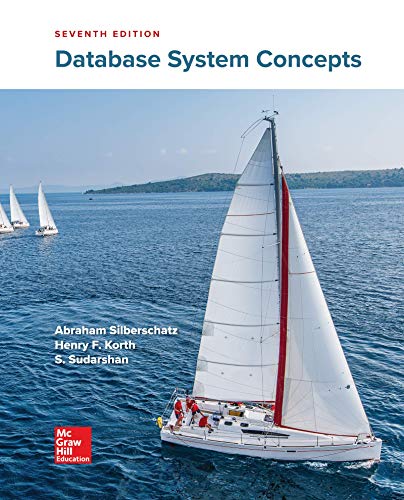
Your task for this assignment is to identify a spanning tree in a connected undirected weighted graph using C++.
1. Implement a spanning tree
2. Each input transaction represents an undirected edge of a connected weighted graph. The edge consists of two unequal uppercase letters representing graph vertices that the edge connects. Each edge has an assigned weight. The edge weight is a positive integer in the range 1 to 99.
The three values on each input transaction are separated by a single space. An input transaction containing the string “end-of-file” signals the end of the graph edge input. After the edge information is read, the spanning tree evaluation process begins. Use an adjacency matrix for recording input edges and show all code used to determine the spanning tree. The input data is assumed to be valid. There is no need to perform data validation on the input data.
3. After the edges of the spanning tree are determined, the tree edges are displayed on the console, one edge per output line, following the message: “Spanning tree:”. Each output line representing a tree edge contains edge vertices separated by space.
Sample input transactions are as follows: A B 2 A C 4 A D 10 A E 6 B C 3 B D 8 B E 1 C D 55 C E 7 D E 9 end-of-file Sample output expected after processing the above input will be as follows:
2 Spanning tree: A C B C A D D E 4.
The program will be run at the command prompt by navigating to the directory containing the executable version of the program after the program is compiled.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Write a program in three parts. The first part should create a linked list of 26 nodes. The second part should fill the list with the letters of the alphabet. The third part should print the contents of the linked list. NOT USE malloc, only use <iostream>. Do not use global variables. The use of global constants c++arrow_forwardTask 4: Matplotlib Import the plotting function by the command: import matplotlib.pyplot as plt Plotting multiple lines Compute the x and y coordinates for points on sine and cosine curves and plot them on the same graph using matplotlib. Add x and y labels to the graph as well. Note: Please provide PYTHON (ANACONDA) code for above task.arrow_forwardIn c++ please Example of Output: Input a string: ........ letters r b с f 2. Demonstrate by writing a program to calculate the frequency of every letter in an input string s. S e a. Your program should be able to input a string. b. Your message should be constructed from at least 50 letters. c. Calculate the frequency of each element in the string and store the results into a linked list. d. Your program should be able to print each letter and its respective frequency. e. Identify the letter with the highest frequency in your message. f. Sort the linked list in ascending order using an algorithm of your choice. Write a short justification of your choice. frequency 1 4 6 12 20 24 30arrow_forward
- Provide full C++ code Building a playlist (of songs) using a linked list and making some operations such as adding songs, removing songs, shuffling them, etc. (Some parts of this lab will closely follow lab 08 - it is imperative that you complete that lab before working on this project). A Node representing a song has the following data members (similar to struct Node of lab 08, except it doesn't have one member for storing data): string uniqueID string songName string artistName int songLength Node* nextNodePtr Your code must have the following three files: Playlist.h - Class declaration for a linked list of Nodes (very similar to lab 08 but with some changes to methods, as described below) with following private data members: private:Node* first;Node* last;int size; Playlist.cpp - Class definition main.cpp - main() function Checkpoint A For checkpoint A, you need to build a playlist (of songs) by readings data members of several songs from a file and printing them. Implement…arrow_forwardJava programming homework please helparrow_forwardin c++ codearrow_forward
- You will create two programs. The first one will use the data structure Stack and the other program will use the data structure Queue. Keep in mind that you should already know from your video and free textbook that Java uses a LinkedList integration for Queue. Stack Program Create a deck of cards using an array (Array size 15). Each card is an object. So you will have to create a Card class that has a value (1 - 10, Jack, Queen, King, Ace) and suit (clubs, diamonds, heart, spade). You will create a stack and randomly pick a card from the deck to put be pushed onto the stack. You will repeat this 5 times. Then you will take cards off the top of the stack (pop) and reveal the values of the cards in the output. As a challenge, you may have the user guess the value and suit of the card at the bottom of the stack. Queue Program There is a new concert coming to town. This concert is popular and has a long line. The line uses the data structure Queue. The people in the line are objects…arrow_forwardPlease help with C++Priority Queue question in C++ language. Sample output also in image. Thanks.arrow_forwardFor the given question use C language (DO NOT USE C++ OR JAVA). Write a complete C program to build an unordered Linked List with exactly 4 nodes, which contains randomly generated integer data. Program should also display all the data in the linked list by looping. Example : Start 15 8 24 17arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
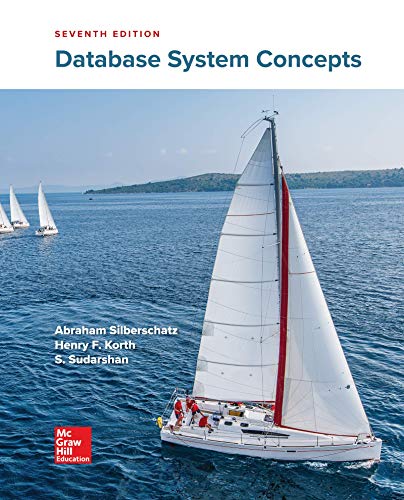
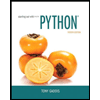
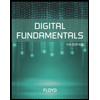
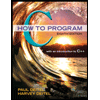
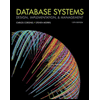
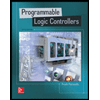