Your program will have a class called FlightController that is going to be derived from two base classes which are called LaunchController and LandingController. The FlightController class inherits all accessible data fields and methods from the LandingController and LaunchController classes.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Your
_________________________________________________________________
//i need more explanations :(
#include<iostream>
#include<algorithm>
using namespace std;
class flight{
protected:
string flight_name;
int launch_hour;
int launch_min;
int land_hour;
int land_min;
int duration;
string state;
public:
flight(string state="Idle"){
this->state=state;
}
void check();
int get_launch_hour();
int get_launch_min();
int get_land_hour();
int get_land_min();
int get_duration();
string get_state();
void set_state(string);
void set_duration(int);
void calculate_landing();
};
int flight::get_duration(){
return duration;
}
void flight::set_duration(int a){
duration=a;
}
int flight::get_launch_hour(){
return launch_hour;
}
int flight::get_launch_min(){
return launch_min;
}
int flight::get_land_hour(){
return land_hour;
}
int flight::get_land_min(){
return land_min;
}
string flight::get_state(){
return state;
}
void flight::set_state(string s){
state=s;
}
void flight::calculate_landing(){
int time;
launch_hour*=60;
time=duration+launch_hour+launch_min;//time=610
land_hour=time/60;//land hour=10
land_min=time-land_hour*60;
launch_hour/=60;
}
void flight::check(){
}
class flight_schedule:public flight{
};
class flight_controller:public flight_schedule{
};
class launch_controller:public flight_controller{
protected:
int launch_hour;
int launch_min;
int launch_hour1;
int launch_min1;
public:
};
class landing_controller:public flight_controller{
protected:
int land_hour;
int land_min;
int land_hour1;
int land_min1;
public:
};
int main(){
flight_schedule *ft[3]={new flight_schedule,new flight_schedule,new flight_schedule};
for( int i=0 ; i<3; i++ )
{
cout<<"Enter the name of the flight: ";
ft[i].get_state();
cout<<"Enter the departure time of the plane:";
ft[i].get_launch_hour();
ft[i].get_launch_min();
cout<<"Enter the duration of the flight in minutes:";
ft[i].get_duration();
cout<<endl<<endl;
}
}

![cmpe225_hw1 (1).pdf
2 / 6
135%
+
Assignment
Write a program that will track certain information about the flights of aircrafts. You
will create your program by referencing the structure given in the diagram (1). Class
definitions, access specifiers, data members and member functions in your program must be
coherent with the diagram. Certain essential variables for operations such as loops, flag
values, counts etc. are not given in the diagram therefore you can define them as your own
extra variables in the program.
LaunchController
LandingController
-launchHour1: int
- launchHour2: int
- launchMin1: int
landHour1: int
- landHour2: int
- landMin1: int
- launchMin2: int
landMin2: int
+ launchSchedule(FlightSchedule" ): void
+ landingSchedule(FlightSchedule" ): void
Extends
Extends
FlightController
# flight_schedule: FlightSchedule
+ FlightController()
+ flights: FlightSchedule"
Flight
|- flightName: string
|- launchHour: int
- launchMin: int
-landHour: int
-landMin: int
FlightSchedule
duration: int
- flights[]: Flight
- state: string
+ method(type): type
+ getlaunchHour(): int
+ getLaunchMin(): int
+ getlandHour(): int
+ getLandMin(): int
+ getState(): string
+ setState(string): void
+ calculatelanding(): void](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F15a145e8-7f8f-437c-aa3d-ad03340b8212%2F1a8add4d-66e3-4aee-9cdd-2896b68828fd%2Fxli7gd_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 5 images

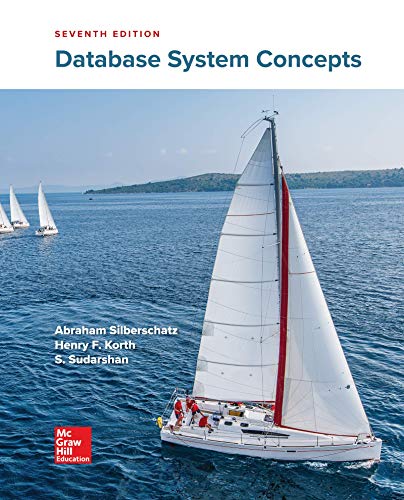
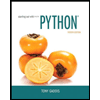
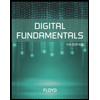
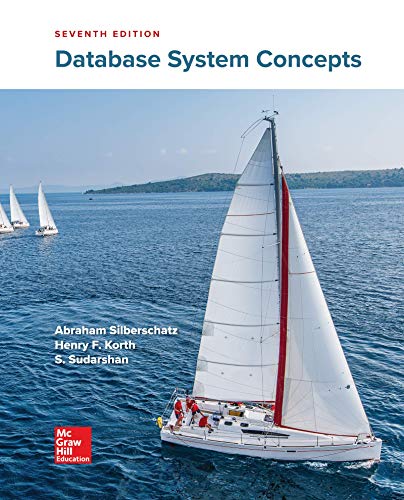
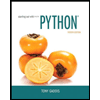
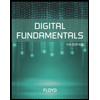
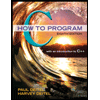
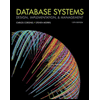
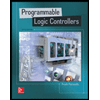