you'll be building a banking simulation that will involve gathering statistics, building tellers, receptionists, clients, and using event queues. I Programming with the Java Collection classes (a priority queue and an ordinary FIFO ( First In First Out) queue will be required) Design of classes Introduction to a pattern (Event queue) used very often in Discrete Event Simulation The general pattern of these types of simulations is this: we have a Clock.. which is simply an integer counter, and an Event queue in which we keep a list of events that will happen in the future. An event is something that changes the state of the system.. for example an arrival of a client, or a departure of a client from a particular server. The kinds of events we will have depend upon the specific problem. In this simulation we can identify initially five types of Events. Arrival of a client to the Bank (or the receptionist, it could be useful to separate these two events though) Departure of a client from the Receptionist. Arrival of a client to the Teller and Departure of a client from the Teller. Each event is has the following attributes: The time it will occur What is to be done when the event is processed. This can coded in a method process( ), which all events should have. Obviously each event type has different code in it's process(). The basic idea of the event list pattern is to use a list of events to control the simulation: We keep a list of events that will happen, ordered by increasing time of occurrence, (and that's why a priority queue is the ideal data structure to use (with smallest time = highest priority)) The algorithm now is simple and very general. While the Clock is less than the end of simulation time DO Remove an event from the event queue, Process it. (That is.. if it is an Arrival, do what must be done at an arrival, if it is a Departure... do what must be done at a departure.) Now is the time for some Object Oriented thinking. Instead of having a separate method that asks each event removed from the Event queue "What are you? If you are an Arrival I will do this, if you are a Departure I will do that.." it is much more useful to have each Event class handle its own processing in a process() method. Now we can just tell each event X to process itself by calling its own process method X.process( ). WHAT HAPPENS AT AN ARRIVAL? The process() method of an Arrival event should do the following (Teller is similar though it doesn't schedule a new Receptionist Arrival): 1. Move the clock to the time of the arrival event 2. 3. 4. 5. Create a client object with the current time of the clock as arrival time If the receptionist is busy then insert the client in the client queue for the receptionist ELSE (the receptionist is free i.e. it is null) place the client in the receptionist and, very important schedule a new RECEP_departure. To schedule a new RECEP_Departure you need to make one by a) calling the constructor of the RECEP _Departure class and b) to insert it in the event queue. The time when the new Departure will happen is determined by calling the function expo with a parameter which should be AVG_TIME_PER_TRANSACTION * number of courses to get a service time, and adding that to the current value of the clock. ( i.e. the departure will happen at time: NOW + a random service time) SCHEDULE a new Receptionist Arrival at time NOW + RandBox.expo (INTERARRIVAL_TIME) WHAT HAPPENS AT A RECEP_DEPARTURE? The process() method of a Departure event should do the following: 1. Move the clock to the time of the Departure event 2. Set the RECEPTIONIST to null 3. Schedule a TELLER_arrival of the client that is leaving the Receptionist 4. If the client queue is NOT empty then remove the first client, set him to the RECEPTIONIST and schedule a RECEP departure (as described above) Calculate the time the client has been in the queue (CLOCK - the client arrival time) and give that time to the statistics object WHAT HAPPENS AT A TELLER_DEPARTURE? Think about it Page 4 of 6 HOW DO WE PUT ALL THAT TOGETHER? Here's one way. Have one class (let's call it BankSim) which contains the Event queue, the client queue, a clock, and two variables (of type Client) RECEPTIONIST and TELLER. This class will have a constructor with parameters for the constants INTERARRIVAL TIME and AVG_TIME_PER_TRANSACTION. Class BankSim will have a method run(simulation_time) that will "run" the simulation. The algorithm of this method is simple: Schedule a first arrival While the clock is less than simulation_time do: o Remove an event from the Eventqueue Call it's process() method. Call methods that will give you the accumulated statistics Print them out PROBLEMS YOU WILL HAVE TO THINK ABOUT (...AND SOLVE) The process() methods of the Arrival and Departure classes need to be able to access the client Queue the Desk and the Event queue. WHAT GOES IN THE main() METHOD? Very simple.. public static void main(String[] args) } BankSims =new BankSim (120.0, 60.0); s.run(5*8*60*60); // seconds in 5 8-hr workdays } //end main()
you'll be building a banking simulation that will involve gathering statistics, building tellers, receptionists, clients, and using event queues. I Programming with the Java Collection classes (a priority queue and an ordinary FIFO ( First In First Out) queue will be required) Design of classes Introduction to a pattern (Event queue) used very often in Discrete Event Simulation The general pattern of these types of simulations is this: we have a Clock.. which is simply an integer counter, and an Event queue in which we keep a list of events that will happen in the future. An event is something that changes the state of the system.. for example an arrival of a client, or a departure of a client from a particular server. The kinds of events we will have depend upon the specific problem. In this simulation we can identify initially five types of Events. Arrival of a client to the Bank (or the receptionist, it could be useful to separate these two events though) Departure of a client from the Receptionist. Arrival of a client to the Teller and Departure of a client from the Teller. Each event is has the following attributes: The time it will occur What is to be done when the event is processed. This can coded in a method process( ), which all events should have. Obviously each event type has different code in it's process(). The basic idea of the event list pattern is to use a list of events to control the simulation: We keep a list of events that will happen, ordered by increasing time of occurrence, (and that's why a priority queue is the ideal data structure to use (with smallest time = highest priority)) The algorithm now is simple and very general. While the Clock is less than the end of simulation time DO Remove an event from the event queue, Process it. (That is.. if it is an Arrival, do what must be done at an arrival, if it is a Departure... do what must be done at a departure.) Now is the time for some Object Oriented thinking. Instead of having a separate method that asks each event removed from the Event queue "What are you? If you are an Arrival I will do this, if you are a Departure I will do that.." it is much more useful to have each Event class handle its own processing in a process() method. Now we can just tell each event X to process itself by calling its own process method X.process( ). WHAT HAPPENS AT AN ARRIVAL? The process() method of an Arrival event should do the following (Teller is similar though it doesn't schedule a new Receptionist Arrival): 1. Move the clock to the time of the arrival event 2. 3. 4. 5. Create a client object with the current time of the clock as arrival time If the receptionist is busy then insert the client in the client queue for the receptionist ELSE (the receptionist is free i.e. it is null) place the client in the receptionist and, very important schedule a new RECEP_departure. To schedule a new RECEP_Departure you need to make one by a) calling the constructor of the RECEP _Departure class and b) to insert it in the event queue. The time when the new Departure will happen is determined by calling the function expo with a parameter which should be AVG_TIME_PER_TRANSACTION * number of courses to get a service time, and adding that to the current value of the clock. ( i.e. the departure will happen at time: NOW + a random service time) SCHEDULE a new Receptionist Arrival at time NOW + RandBox.expo (INTERARRIVAL_TIME) WHAT HAPPENS AT A RECEP_DEPARTURE? The process() method of a Departure event should do the following: 1. Move the clock to the time of the Departure event 2. Set the RECEPTIONIST to null 3. Schedule a TELLER_arrival of the client that is leaving the Receptionist 4. If the client queue is NOT empty then remove the first client, set him to the RECEPTIONIST and schedule a RECEP departure (as described above) Calculate the time the client has been in the queue (CLOCK - the client arrival time) and give that time to the statistics object WHAT HAPPENS AT A TELLER_DEPARTURE? Think about it Page 4 of 6 HOW DO WE PUT ALL THAT TOGETHER? Here's one way. Have one class (let's call it BankSim) which contains the Event queue, the client queue, a clock, and two variables (of type Client) RECEPTIONIST and TELLER. This class will have a constructor with parameters for the constants INTERARRIVAL TIME and AVG_TIME_PER_TRANSACTION. Class BankSim will have a method run(simulation_time) that will "run" the simulation. The algorithm of this method is simple: Schedule a first arrival While the clock is less than simulation_time do: o Remove an event from the Eventqueue Call it's process() method. Call methods that will give you the accumulated statistics Print them out PROBLEMS YOU WILL HAVE TO THINK ABOUT (...AND SOLVE) The process() methods of the Arrival and Departure classes need to be able to access the client Queue the Desk and the Event queue. WHAT GOES IN THE main() METHOD? Very simple.. public static void main(String[] args) } BankSims =new BankSim (120.0, 60.0); s.run(5*8*60*60); // seconds in 5 8-hr workdays } //end main()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
in Java. Bank simulation only

Transcribed Image Text:you'll be building a banking simulation that will involve gathering statistics, building
tellers, receptionists, clients, and using event queues. I
Programming with the Java Collection classes (a priority queue and an ordinary FIFO ( First In First
Out) queue will be required)
Design of classes
Introduction to a pattern (Event queue) used very often in Discrete Event Simulation
The general pattern of these types of simulations is this: we have a Clock.. which is simply an integer
counter, and an Event queue in which we keep a list of events that will happen in the future. An event is
something that changes the state of the system.. for example an arrival of a client, or a departure of a
client from a particular server. The kinds of events we will have depend upon the specific problem.
In this simulation we can identify initially five types of Events.
Arrival of a client to the Bank (or the receptionist, it could be useful to separate these two events though)
Departure of a client from the Receptionist.
Arrival of a client to the Teller and
Departure of a client from the Teller.
Each event is has the following attributes:
The time it will occur
What is to be done when the event is processed. This can coded in a method process( ), which all
events should have. Obviously each event type has different code in it's process().
The basic idea of the event list pattern is to use a list of events to control the simulation:
We keep a list of events that will happen, ordered by increasing time of occurrence, (and that's why a
priority queue is the ideal data structure to use (with smallest time = highest priority))
The algorithm now is simple and very general.
While the Clock is less than the end of simulation time DO
Remove an event from the event queue,
Process it. (That is.. if it is an Arrival, do what must be done at an arrival, if it is a
Departure... do what must be done at a departure.)
Now is the time for some Object Oriented thinking.
Instead of having a separate method that asks each event removed from the Event queue "What are you?
If you are an Arrival I will do this, if you are a Departure I will do that.." it is much more useful to have
each Event class handle its own processing in a process() method. Now we can just tell each event X to
process itself by calling its own process method X.process( ).
![WHAT HAPPENS AT AN ARRIVAL?
The process() method of an Arrival event should do the following (Teller is similar though it doesn't schedule a new
Receptionist Arrival):
1. Move the clock to the time of the arrival event
2.
3.
4.
5.
Create a client object with the current time of the clock as arrival time
If the receptionist is busy then insert the client in the client queue for the receptionist
ELSE (the receptionist is free i.e. it is null) place the client in the receptionist and, very important schedule a
new RECEP_departure.
To schedule a new RECEP_Departure you need to make one by
a) calling the constructor of the RECEP _Departure class and
b) to insert it in the event queue. The time when the new Departure will happen is determined by calling the
function expo with a parameter which should be AVG_TIME_PER_TRANSACTION * number of courses to get a
service time, and adding that to the current value of the clock. ( i.e. the departure will happen at time: NOW +
a random service time)
SCHEDULE a new Receptionist Arrival at time NOW + RandBox.expo (INTERARRIVAL_TIME)
WHAT HAPPENS AT A RECEP_DEPARTURE?
The process() method of a Departure event should do the following:
1. Move the clock to the time of the Departure event
2. Set the RECEPTIONIST to null
3. Schedule a TELLER_arrival of the client that is leaving the Receptionist
4. If the client queue is NOT empty then remove the first client, set him to the RECEPTIONIST and schedule a
RECEP departure (as described above)
Calculate the time the client has been in the queue (CLOCK - the client arrival time) and give that time to the
statistics object
WHAT HAPPENS AT A TELLER_DEPARTURE?
Think about it
Page 4 of 6
HOW DO WE PUT ALL THAT TOGETHER?
Here's one way. Have one class (let's call it BankSim) which contains the Event queue, the client queue, a clock, and
two variables (of type Client) RECEPTIONIST and TELLER. This class will have a constructor with parameters for the
constants INTERARRIVAL TIME and AVG_TIME_PER_TRANSACTION.
Class BankSim will have a method run(simulation_time) that will "run" the simulation. The algorithm of this
method is simple:
Schedule a first arrival
While the clock is less than simulation_time do:
o
Remove an event from the Eventqueue
Call it's process() method.
Call methods that will give you the accumulated statistics
Print them out
PROBLEMS YOU WILL HAVE TO THINK ABOUT (...AND SOLVE)
The process() methods of the Arrival and Departure classes need to be able to access the client Queue the Desk and
the Event queue.
WHAT GOES IN THE main() METHOD?
Very simple..
public static void main(String[] args)
}
BankSims =new BankSim (120.0, 60.0);
s.run(5*8*60*60); // seconds in 5 8-hr workdays
} //end main()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5d82705f-57fb-47b5-bb9c-82d4805aa4d0%2Fd7f85827-c4de-4fc8-823b-dd4d871a1271%2Fppgljmj_processed.png&w=3840&q=75)
Transcribed Image Text:WHAT HAPPENS AT AN ARRIVAL?
The process() method of an Arrival event should do the following (Teller is similar though it doesn't schedule a new
Receptionist Arrival):
1. Move the clock to the time of the arrival event
2.
3.
4.
5.
Create a client object with the current time of the clock as arrival time
If the receptionist is busy then insert the client in the client queue for the receptionist
ELSE (the receptionist is free i.e. it is null) place the client in the receptionist and, very important schedule a
new RECEP_departure.
To schedule a new RECEP_Departure you need to make one by
a) calling the constructor of the RECEP _Departure class and
b) to insert it in the event queue. The time when the new Departure will happen is determined by calling the
function expo with a parameter which should be AVG_TIME_PER_TRANSACTION * number of courses to get a
service time, and adding that to the current value of the clock. ( i.e. the departure will happen at time: NOW +
a random service time)
SCHEDULE a new Receptionist Arrival at time NOW + RandBox.expo (INTERARRIVAL_TIME)
WHAT HAPPENS AT A RECEP_DEPARTURE?
The process() method of a Departure event should do the following:
1. Move the clock to the time of the Departure event
2. Set the RECEPTIONIST to null
3. Schedule a TELLER_arrival of the client that is leaving the Receptionist
4. If the client queue is NOT empty then remove the first client, set him to the RECEPTIONIST and schedule a
RECEP departure (as described above)
Calculate the time the client has been in the queue (CLOCK - the client arrival time) and give that time to the
statistics object
WHAT HAPPENS AT A TELLER_DEPARTURE?
Think about it
Page 4 of 6
HOW DO WE PUT ALL THAT TOGETHER?
Here's one way. Have one class (let's call it BankSim) which contains the Event queue, the client queue, a clock, and
two variables (of type Client) RECEPTIONIST and TELLER. This class will have a constructor with parameters for the
constants INTERARRIVAL TIME and AVG_TIME_PER_TRANSACTION.
Class BankSim will have a method run(simulation_time) that will "run" the simulation. The algorithm of this
method is simple:
Schedule a first arrival
While the clock is less than simulation_time do:
o
Remove an event from the Eventqueue
Call it's process() method.
Call methods that will give you the accumulated statistics
Print them out
PROBLEMS YOU WILL HAVE TO THINK ABOUT (...AND SOLVE)
The process() methods of the Arrival and Departure classes need to be able to access the client Queue the Desk and
the Event queue.
WHAT GOES IN THE main() METHOD?
Very simple..
public static void main(String[] args)
}
BankSims =new BankSim (120.0, 60.0);
s.run(5*8*60*60); // seconds in 5 8-hr workdays
} //end main()
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
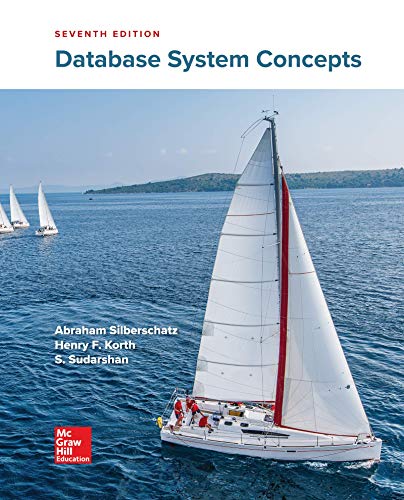
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
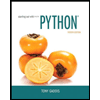
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
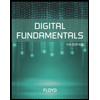
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
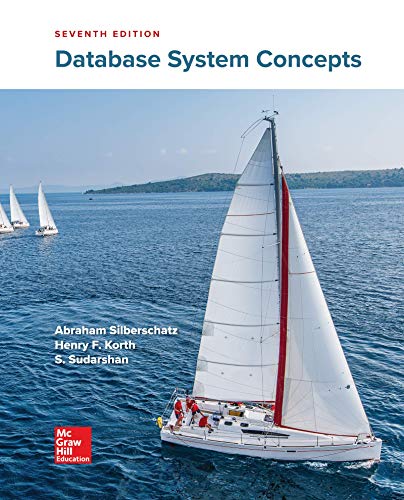
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
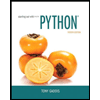
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
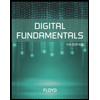
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
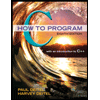
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
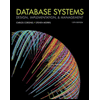
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
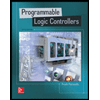
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education