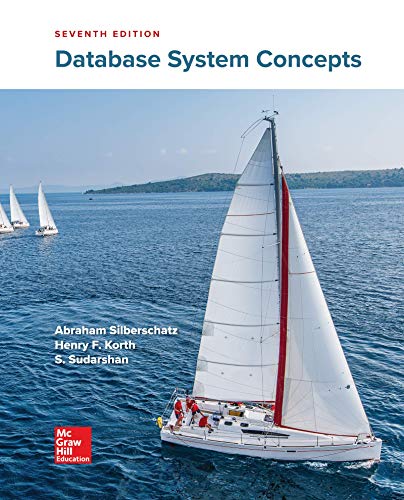
You will write a library of static methods that performs various geometric transforms on polygons. Mathematically, a polygon is defined by its sequence of vertices (x0, y0), (x1, y1), (x2, y2), …. In Java, we will represent a polygon by storing the x- and y-coordinates of the vertices in two parallel arrays x[] and y[]. For example:
// a polygon with these four vertices: // (0, 0), (1, 0), (1, 2), (0, 1) double x[] = { 0, 1, 1, 0 }; double y[] = { 0, 0, 2, 1 }; // Draw the polygon StdDraw.polygon(x, y); |
Transform2D.java
Write a two-dimensional transformation library Transform2D.java by implementing the following API:
public class Transform2D { // Returns a new array object that is an exact copy of the given array. // The given array is not mutated. public static double[] copy(double[] array) // Scales the polygon by the factor alpha. public static void scale(double[] x, double[] y, double alpha) // Translates the polygon by (dx, dy). public static void translate(double[] x, double[] y, double dx, double dy) // Rotates the polygon theta degrees counterclockwise, about the origin. public static void rotate(double[] x, double[] y, double theta) // Tests each of the API methods by directly calling them. public static void main(String[] args) } |
Requirements
- The API expects the angles to be in degrees, but Java's trigonometric functions take the arguments in radians. Use Math.toRadians() to convert from degrees to radians.
- The transformation methods scale(), translate(), and rotate() mutate the arrays, while copy() returns a new array.
- The main method must test each method of the Transform2D library. In other words, you must call each Transform2D method from main. You should experiment with various data so you are confident that your methods are implemented correctly.
- You can assume the following about the inputs: the arrays passed to scale(), translate(), and rotate() are not null, are the same length, and do not contain the values NaN, Double.POSITIVE_INFINITY, or Double.NEGATIVE_INFINITY.
- The array passed to copy() is not null.
- The values for the parameters alpha, theta, dx, and dy are not NaN, Double.POSITIVE_INFINITY, or Double.NEGATIVE_INFINITY.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- The java program ArrayTest.java is intended to do the following: • Fill the array a with integer values input from the file ints.txt. • (Shift-right) Shift the elements of a to the right one position and put the last element of array a in the first position in a (wrap around). Example: if a originally contained (1, 2, 3, 4} then the resulting array a would be (4, 1, 2, 3} What command would be given on the command line to redirect the input to the program ArrayTest to come from the text file ints.txt? Enter your answer herearrow_forwardWrite a program RURottenTomatoes.java that creates a 2 dimensional integer array of movie ratings from the command line arguments and displays the index of the movie that has the highest sum of ratings. Ratings range from 1 to 5 (inclusive). The rows of the 2D array correspond to movie reviewers and the columns of the 2D array correspond to movies. The reviewer at index 2 gave movie at index 0 a rating of 4. Take a look at the following example for an explanation on the command line arguments sequenc. The first argument corresponds to the number of reviewers and the second argument corresponds to the number of movies (the dimensions of the 2D integer array). Following are the movie ratings in a row-major order. This means that the first row is filled first, then the second row, etc. In the example above there are 3 reviewers and 2 movies, and the program displays 0 (zero) because the movie at index zero has the highest sum of ratings (movie 0 has sum of ratings equals to 12 while…arrow_forwardWrite a library of static methods that performs various geometric transforms on polygons. Mathematically, a polygon is defined by its sequence of vertices (xo, y o), (x 1, y 1), (x 2, y 2), ... In Java, we will represent a polygon by storing the x- and y-coordinates of the vertices in two parallel arrays x[] and y[]. Three useful geometric transforms are scale, translate and rotate. o Scale the coordinates of each vertex (x, y ) by a factor a. o X;= a Xi o y;=ay o Translate each vertex (x , y ) by a given offset (dx, dy). o X; = X; + dx o yj=y+ dy o Rotate each vertex (x , y) by 0 degrees counterclockwise, around the origin. O X; = X; Cos 0 – y sin e o y;= y cos 0 + x; sin e Write a two-dimensional transformation library by implementing the following API:arrow_forward
- Using Java, Define a method named sortArray that takes an array of integers and the number of elements in the array as parameters. Method sortArray() modifies the array parameter by sorting the elements in descending order (highest to lowest). Then write a main program that reads a list of integers from input, stores the integers in an array, calls sortArray(), and outputs the sorted array. The first input integer indicates how many numbers are in the list. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 10 4 39 12 2 the output is: 39,12,10,4,2, For coding simplicity, follow every output value by a comma, including the last one. Your program must define and call the following method:public static void sortArray(int[] myArr, int arrSize)arrow_forwardWrite a Java program that expands a given binomial (x + y)^n, where integer n is user input. To do the work of the binomial expression, first create a method that accepts n as a parameter and then returns an array holding the coefficients needed for the binomial expansion using the Pascal’s Triangle method. Create a 2nd method which takes the array holding the coefficients as a parameter and prints the appropriate binomial expansion. For example, if n = 5 is entered by the user, the method calculating the coefficients should return {1,5,10,10,5,1} and the method that prints the expansion should print the following: (x + y)^5 = x^5 + 5x^4y + 10x^3y^2 + 10x^2y^3 + 5xy^4 + y^5 Your main method should use an appropriate loop for repeated inputs and automatically call the methods to calculate the coefficients and print the binomial expansion. There isn’t a need for a menu although you should ask the user if they want to quit or continue after their binomial expansion is printed each time.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
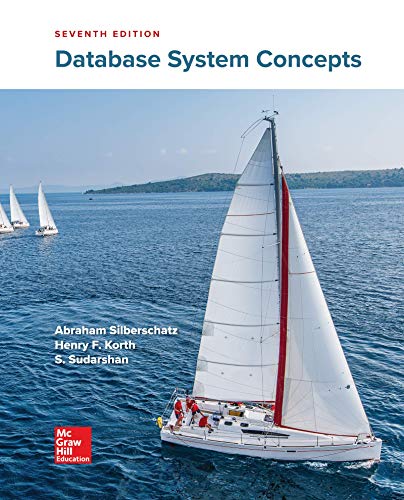
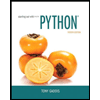
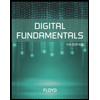
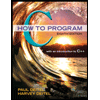
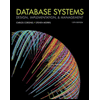
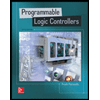