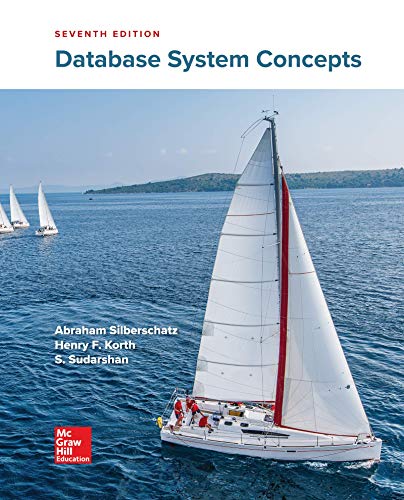
Concept explainers
I need help with this Java problem to output as it's explained in this image below:
import java.util.Scanner;
public class LabProgram {
// Read size and create an array of size ints.
// Read size ints, storing them in the array.
// Return the array.
public static int[] readNums() {
Scanner scnr = new Scanner(System.in);
int size = scnr.nextInt(); // Read array size
int[] nums = new int[size]; // Create array
for (int j = 0; j < size; ++j) { // Read the numsbers
nums[j] = scnr.nextInt();
}
return nums; // Return the array
}
// Output the numbers in nums, separated by spaces.
// No space or newline will be output before the first number or after the last.
public static void printNums(int[] nums) {
for (int i = 0; i < nums.length; i++) {
System.out.print(nums[i]);
if (i < nums.length) {
System.out.print(" ");
}
}
}
public static void merge(int[] numbers, int i, int j, int k) {
int mergedSize = k - i + 1;
int mergedNumbers[] = new int[mergedSize];
int mergePos;
int leftPos;
int rightPos;
mergePos = 0;
leftPos = i;
rightPos = j + 1;
while (leftPos <= j && rightPos <= k) {
if (numbers[leftPos] < numbers[rightPos]) {
mergedNumbers[mergePos] = numbers[leftPos];
++leftPos;
}
else {
mergedNumbers[mergePos] = numbers[rightPos];
++rightPos;
}
++mergePos;
}
while (leftPos <= j) {
mergedNumbers[mergePos] = numbers[leftPos];
++leftPos;
++mergePos;
}
while (rightPos <= k) {
mergedNumbers[mergePos] = numbers[rightPos];
++rightPos;
++mergePos;
}
for (mergePos = 0; mergePos < mergedSize; ++mergePos) {
numbers[i + mergePos] = mergedNumbers[mergePos];
}
}
public static void mergeSort(int numbers[], int i, int k) {
int j;
if (i < k) {
j = (i + k) / 2;
/* Trace output added to code in book */
System.out.printf("%d %d | %d %d\n", i, j, j+1, k);
mergeSort(numbers, i, j);
mergeSort(numbers, j + 1, k);
merge(numbers, i, j, k);
}
}
public static void main(String[] args) {
int[] numbers = readNums();
System.out.print("unsorted: ");
printNums(numbers);
System.out.println("\n");
mergeSort(numbers, 0, numbers.length - 1);
System.out.print("\nsorted: ");
printNums(numbers);
}
}
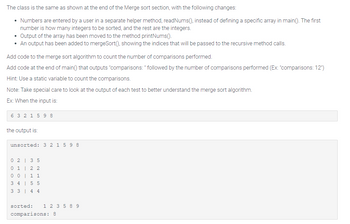

Step by stepSolved in 3 steps with 1 images

- Using Java.arrow_forwardStringFun.java import java.util.Scanner; // Needed for the Scanner class 2 3 /** Add a class comment and @tags 4 5 */ 6 7 public class StringFun { /** * @param args not used 8 9 10 11 12 public static void main(String[] args) { Scanner in = new Scanner(System.in); System.out.print("Please enter your first name: "); 13 14 15 16 17 18 System.out.print("Please enter your last name: "); 19 20 21 //Output the welcome message with name 22 23 24 //Output the length of the name 25 26 27 //Output the username 28 29 30 //Output the initials 31 32 33 //Find and output the first name with switched characters 34 //All Done! } } 35 36 37arrow_forwardneed help finishing/fixing this java code so it prints out all the movie names that start with the first 2 characters the user inputs the code is as follows: Movie.java: import java.io.File;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Movie{public String name;public int year;public String genre;public static ArrayList<Movie> loadDatabase() throws FileNotFoundException {ArrayList<Movie> result=new ArrayList<>();File f=new File("db.txt");Scanner inputFile=new Scanner(f);while(inputFile.hasNext()){String name= inputFile.nextLine();int year=inputFile.nextInt();inputFile.nextLine();String genre= inputFile.nextLine();Movie m=new Movie(name, year, genre);//System.out.println(m);result.add(m);}return result;}public Movie(String name, int year, String genre){this.name=name;this.year=year;this.genre=genre;}public boolean equals(int year, String genre){return this.year==year&&this.genre.equals(genre);}public String…arrow_forward
- import java.util.Scanner;public class main{public static void main(String[] args){Scanner sc = new Scanner(System.in):int year, day, weekday;String month;String outday = "";System.out.printf("Enter the month%n");month = sc.nextLine();System.out.printf("Enter the day%n");month = sc.nextInt();weekay = find_day(month, day);outday = out_weekday(weekday);System.out.printf("The day is: %s%n", outday);}} Change the program from the previous example to take command line arguments instead of using the Scanner. For example, running the program like this:> java OutDays March 14will output the weekday string (which is Thursday) on the console for March 14, 2019). public static void main(String[] args){ int year, day, weekday; String month; String outday = ""; // #### your code here for accessing the command line arguments weekday = find_day(month, day); outday = out_weekday(weekday); System.out.printf("The day is: %s%n", outday);}arrow_forwardConvert the following java code to C++ //LabProgram.javaimport java.util.Scanner;public class LabProgram {public static void main(String[] args) {//defining a Scanner to read input from the userScanner input = new Scanner(System.in);//reading the value for Nint N = input.nextInt();//creating a 1xN matrixint[] m1 = new int[N];//creating an NxN matrixint[][] m2 = new int[N][N];//creating a 1xN matrix to store the resultint[] result = new int[N];//looping and reading N integers into m1for (int i = 0; i < N; i++) {m1[i] = input.nextInt();}//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//reading an integer and storing it into m2 at position i,jm2[i][j] = input.nextInt();}}//multiply m1 and m2, store result in result//looping from 0 to N-1for (int i = 0; i < N; i++) {//looping from 0 to N-1for (int j = 0; j < N; j++) {//multiplying value at index j in m1 with value at j,i in m2, adding to current value at index i// on…arrow_forwardimport java.util.Scanner ; //This program enables the user enters 10 int between -100 and 100 //then a menu is presented to the user to choose an operation to be //performed on the entered numbers. //operations: add all numbers and display their total //display the average of the numbers //find and display all negative numbers //find and display odd numbers //display all numbers in reverse order //display all 5 multiples public class OPonArrays { public static void main (String [] main) { int SIZE = 10; int [] nums= new int [SIZE]; Scanner KB = new Scanner(System.in); // populate the array for(int i = 0; i < 10; i++) { System.out.println("please enter a number between -100 and 100"); nums[i] = KB.nextInt(); } //display all elments for(int i = 0; i < 10; i++) { System .out.println(nums[i]); } fix the errorarrow_forward
- Write the following Java program Allocate an array a of ten integers. Put the number 17 as the initial element of the array. Put the number 29 as the last element of the array. Fill the remaining elements with –1. Add 1 to each element of the array. Print all elements of the array, one per line. Print all elements of the array in a single line, separated by commas.arrow_forward- Write a java program that contains a two-dimensional array of 2 rows and 3 columns. Find the sum of each row in the array, trace the code using a tracing table. - Note: No two students can try the same inputs. - You should submit the code and the tracing table in a handwritten pdf file. - Also, you should submit the code and a screenshot of the output using a word file. Tracing Table may look like the following table: Line# i j Array[0][0] Array[0][1] Array[0][2] Array[1][0] Array[1][1] Array[1][2] rowSum Output …….arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
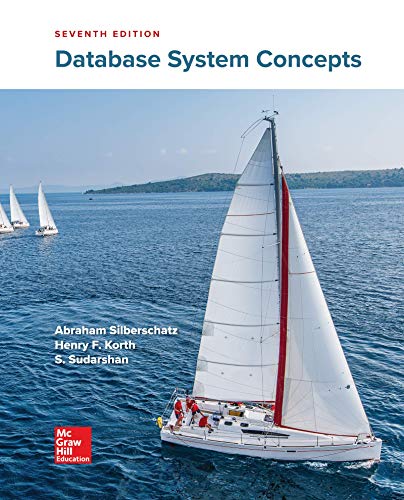
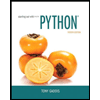
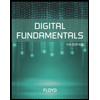
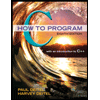
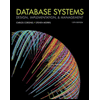
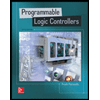