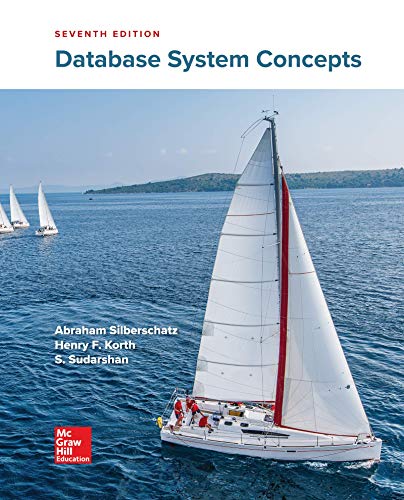
You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment.
In this problem, you need to implement operation bit encoding for Assembly instructions. Given a line of text, your
Input Format
The input to the program will consist of some number of lines. Each line contains some text, either a valid Assembly instruction type (with no extra whitespace or other characters, e.g. READ on a line by itself) or some other text.
Constraints
There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a performance-centric problem.
Output Format
The output should consist of several lines, each of which contains a 4-bit opcode/optype string (e.g. 1000, corresponding to READ). If an instruction has undefined bits, such as NAND, those undefined bits should be represented wuth asterisks: *.
Input 0
ADD
Output 0
0000
Explanation 0
The input consists of a single line, containing the instruction "ADD", which corresponds to the opcode/optype 0000.
Input 1
SUB
SUBI
Sample Output 1
00100011
Explanation 1
Both lines in this input are valid instruction types, with no additional whitespace or other characters, so they should be encoded according to the Assembly instruction table.
Sample Input 2
NAND
NOR
WRITE
JMP
OUT
Sample Output 2
010*
011*
1010
1011
1100
Explanation 2
All of the lines contain valid instructions that should be encoded. Since the second optype bit of both NAND and NOR is undefined in Assembly, it is left as a wildcard * here.
Sample Input 3
ADDI
a
READ
WRITE WRITE
BEQ
jjjj
INP
Sample Output 3
0001
1000
1001
1110
Explanation 3
This test case contains 3 invalid lines: 1. "a" is not a valid instruction type, so its line should be skipped. 2. "WRITE WRITE" is not a valid instruction type; even though WRITE is a valid instruction, the entire line should be considered for this problem. 3. "jjjj" is not a valid instruction type. Since 3 lines out of the 7 input lines are invalid, the output only contains 4 lines.
![Instruction Set
Arithmetic
ADD
ADDI
SUB
SUBI
Logical
NAND
NOR
Memory
READ
WRITE
Branch
JMP
BEQ
INPUT/OUTPUT
INP
OUT
EXAMPLE
ADD RO, R1, R2 (i.e. RO = R1 + R2)
ADDI RO, R1, 8 (i.e. RO= R1 + 8)
SUB RO, R1, R2 (i.e. RO =R1 - R2)
SUBI RO, R1, 8 (i.e. RO= R1-8)
EXAMPLE
NAND RO, R1, R2 (i.e. RO= R1 NAND R2)
NOR RO, R1, R2 (i.e. R0 = R1 NAND R2)
EXAMPLE
READ RO, R1 (i.e. RO= MEM[R1])
WRITE RO, R1 (i.e. MEM[RO] = R1)
EXAMPLE
JMP RO (i.e. PCOut = RO)
BEQ RO, R1 (i.e. PCOut = R0 if R1==0)
EXAMPLE
INP RO (i.e. RO = MEM[KEYBOARD])
OUT RO, R1 (i.e. SCREEN[RO] = R1)
In[15] In[14] In[13] In[12]
OPCODE
OPTYPE
0
THE
0
1
0
1
0
1
In[15] In[14] In[13] In[12] In[11] In[10] In[9] In[8] In[7] In[6]
OPCODE
OPTYPE
DEST REGISTER
SRC1 REGISTER
0
In[15] In[14]
OPCODE
1
1
0
0
1 0
In[15] In[14] In[13] In[12]
OPCODE
OPTYPE
1
1
0
1
1
1
0
In[15] In[14] In[13] In[12]
OPCODE
OPTYPE
0
0
1
Any Reg: 000 to 111
1
In[13] |In[12] |In[11] In[10] In[9]
OPTYPE
LEFT SIDE
DEST REGISTER
DEST PTR REGISTER
In[11] In[10] In[9]
TARGET ADDRESS
In[11] In[10] In[9] In[8] In[7] In[6]
DEST REGISTER
SRC1 REGISTER
1
0
0
0
Any Reg: 000 to 111 Any Reg: 000 to 111
Any Reg: 000 to 111
In[11] In[10] In[9]
TARGET ADDRESS
Any Reg: 000 to 111
Any Reg: 000 to 111
In[8] In[7] In[6]
RIGHT SIDE
SRC PTR REGISTER
SRC REGISTER
In[8] In[7] In[6]
SRC REGISTER
Any Reg: 000 to 111
In[8] In[7] In[6]
SRC REGISTER
Any Reg: 000 to 111
In[5] In[4] In[3] In[2] In[1] In[0]
SRC2 REGISTER
Any Reg: 000-111
Six Bit Immediate Value (0-63)
Any Reg: 000-111
Six Bit Immediate Value (0-63)
In[5] In[4] In[3] In[2] In[1] In[0]
SRC2 REGISTER
UNUSED
Any Reg: 000 to
111
In[5] In[4] In[3] In[2] In[1] In[0]
In[5] In[4] In[3] In[2] In[1] In[0]
In[5] In[4] In[3] In[2] In[1] In[0]](https://content.bartleby.com/qna-images/question/7912ce54-d52f-486c-b093-cdbfa1d021eb/ec28c450-705f-4b6a-b3e3-08762434dc57/6p49g28_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Write an interactive program that inputs the coefficient of a quadratic equation, computes, and displays the roots of the quadratic equation. All input, computation, and output should be done using double-precision floating-point instructions and registers. The program should handle the case of complex roots and displays the results properly. note: the solution should be in MIPS assembly language.arrow_forwardIm having trouble with this problem in writing a masm code for assembly language.arrow_forwardWHats the pseduo code in c++/python for number 2?arrow_forward
- If moderately active persons cut their calorie intake by 500 calories a day, they can typically lose about 4 pounds a month. Write a program that has the users enter their starting weight and then creates and displays a table showing what their expected weight will be at the end of each month for the next 6 months if they stay on this diet. In 80x86 assembly language.arrow_forwardhow to write a python program - 1. Read the initial grid specifications from the input text file and create a 2-dimensional list structure to represent the grid.2. Implement a function to display the current state of the grid on the console.3. Implement the command processing logic to move Pacman on the grid according to the user's input.4. Update the grid state based on the user's input and display the updated grid.5. Repeat step 3 and 4 until the user chooses to quit the game.arrow_forwardWrite an assembly language program in MIPS that repeatedly asks the user for a scale F or a C (for"Fahrenheit" or "Celsius") on one line followed by an integertemperature on the next line. It then converts the given temperature tothe other scale. Use the formulas:F = (9/5)C + 32C = (5/9)(F - 32) Exit the loop when the user types "Q/q". Assume that all input is correct.For example: Sample Output: Enter Scale : FEnter Temperature: 32Celsius Temperature: 0CEnter Scale : CEnter Temperature: 100Fahrenheit Temperature: 212FEnter Scale : Qdonearrow_forward
- If the port A, B, C address of 8255 IC are 38H, 3AH, 3CH and its are configured as port A is an output port, both ports B and C are input ports, and all three ports are set up for mode 0 operation. Construct a program that will input the data at ports B and C, find the difference (port C) - (port B), and output this difference to port A.arrow_forwardWrite a code in sim8085 for the following problem: The pressure of two boilers is monitored and controlled by a microcomputer works based on microprocessor programming. A set of 6 readings of first boiler, recorded by six pressure sensors, which are stored in the memory location starting from 2050H. A corresponding set of 6 reading from the second boiler is stored at the memory location starting from 2060H. Each reading from the first set is expected to be higher than the corresponding position in the second set of readings. Write an 8085 sequence to check whether the first set of reading is higher than the second one or not. If all the readings of first set is higher than the second set, store 00 in the ‘D’ register. If any one of the readings is lower than the corresponding reading of second set, stop the process and store FF in the register ‘D’. Data (H): First set: 78, 89, 6A, 80, 90, 85 Second Set:71, 78, 65, 89, 56, 75arrow_forwardI ran the code and this is the error I got? Please fix the code and make sure the code compiles propertly. I really need to get full points on this.arrow_forward
- Do it on sim8085arrow_forwardWrite a MIPS assembly program that can draw a rectangle pattern onto the console output based on a given set of parameter values. To fully define the rectangle pattern, the following four parameters will be used: 1. Height: the number of lines used to draw the rectangle 2. Width: the number of characters in each line. 3. Border: the character used to draw the rectangle's periphery 4. Fill: the character used to draw the rectangle's inner points For example, a rectangle of Height=5, Width=D4, Border=*' and Fill ='@' would print as follows: **** * @ @ * * @ @ * * @ @ * ****arrow_forwardUsing MIPS in assembly language, you are to create a MIPS program that demonstrates that the associative law fails in addition for floating point numbers (single or double precision). You only need to demonstrate it for single precision. Remember the associative law is a + (b + c) = (a + b) + c. *Include a screenshot of the code running, Also have a lot of comments in your program as this is an assembly language program. Make the code neat and readable.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
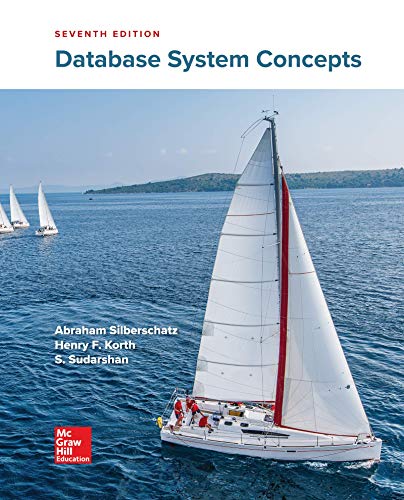
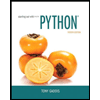
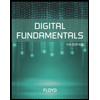
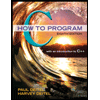
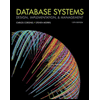
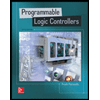