you create a programmer-defined class and then use it in a C++ program. The program should create two Rectangle objects and find their area and perimeter. Instructions Ensure the class file named Rectangle.cpp is open in your editor. In the Rectangle class, create two private attributes named length and width. Bothlength and width should be data type double. Write public set methods to set the values for length and width. Write public get methods to retrieve the values for length and width. Write a public calculateArea()method and a public calculatePerimeter() method to calculate and return the area of the rectangle and the perimeter of the rectangle. Open the file named MyRectangleClassProgram.cpp. In the MyRectangleClassProgram, create two Rectangle objects named rectangle1 and rectangle2 using the default constructor as you saw in MyEmployeeClassProgram.cpp. Set the length of rectangle1 to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.0. Print the value of rectangle1’s perimeter and area, and then print the value of rectangle2’s perimeter and area.
you create a programmer-defined class and then use it in a C++ program. The program should create two Rectangle objects and find their area and perimeter. Instructions Ensure the class file named Rectangle.cpp is open in your editor. In the Rectangle class, create two private attributes named length and width. Bothlength and width should be data type double. Write public set methods to set the values for length and width. Write public get methods to retrieve the values for length and width. Write a public calculateArea()method and a public calculatePerimeter() method to calculate and return the area of the rectangle and the perimeter of the rectangle. Open the file named MyRectangleClassProgram.cpp. In the MyRectangleClassProgram, create two Rectangle objects named rectangle1 and rectangle2 using the default constructor as you saw in MyEmployeeClassProgram.cpp. Set the length of rectangle1 to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.0. Print the value of rectangle1’s perimeter and area, and then print the value of rectangle2’s perimeter and area.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
In this lab, you create a programmer-defined class and then use it in a C++
Instructions
- Ensure the class file named Rectangle.cpp is open in your editor.
- In the Rectangle class, create two private attributes named length and width. Bothlength and width should be data type double.
- Write public set methods to set the values for length and width.
- Write public get methods to retrieve the values for length and width.
- Write a public calculateArea()method and a public calculatePerimeter() method to calculate and return the area of the rectangle and the perimeter of the rectangle.
- Open the file named MyRectangleClassProgram.cpp.
- In the MyRectangleClassProgram, create two Rectangle objects named rectangle1 and rectangle2 using the default constructor as you saw in MyEmployeeClassProgram.cpp.
- Set the length of rectangle1 to 10.0 and the width to 5.0. Set the length of rectangle2 to 7.0 and the width to 3.0.
- Print the value of rectangle1’s perimeter and area, and then print the value of rectangle2’s perimeter and area.
- Execute the program by clicking the Run button at the bottom of the screen
GIVEN CODE
MyRectangleClassProgram.cpp
// This program uses the programmer-defined Rectangle class.
#include "Rectangle.cpp"
#include <iostream>
using namespace std;
int main()
{
Rectangle rectangle1;
Rectangle rectangle2;
rectangle1.setLength(10.0);
rectangle1.setWidth(5.0);
rectangle2.setLength(7.0);
rectangle2.setWidth(3.0);
cout << "Perimeter of rectangle1 is " << rectangle1.calculatePerimeter() << endl;
cout << "Area of rectangle1 is " << rectangle1.calculateArea() << endl;
cout << "Perimeter of rectangle2 is " << rectangle2.calculatePerimeter() << endl;
cout << "Area of rectangle2 is " << rectangle2.calculateArea() << endl;
return 0;
}
Rectangle.cpp
// Rectangle.cpp
using namespace std;
class Rectangle
{
public:
// Declare public methods here
private:
// Create length and width here
};
void Rectangle::setLength(double len)
{
}
void Rectangle::setWidth(double wid)
{
// write setWidth here
}
double Rectangle::getLength()
{
// write getLength here
}
double Rectangle::getWidth()
{
// write getWidth here
}
double Rectangle::calculateArea()
{
// write calculateArea here
}
double Rectangle::calculatePerimeter()
{
// write calculatePerimeter here
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
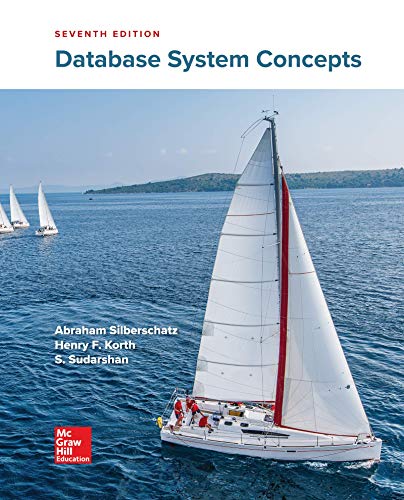
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
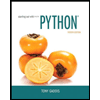
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
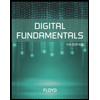
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
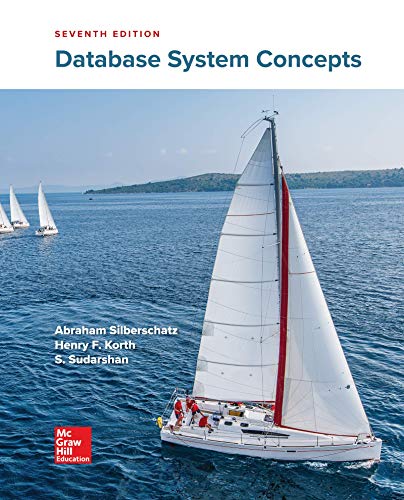
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
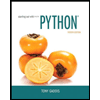
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
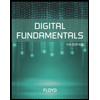
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
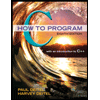
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
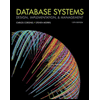
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
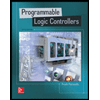
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education