You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add (must be completed) to add nodes to this linked list(I created one linked list in TestLinkedList). I’ve already created the class for you and have some completed method in there, your job is to complete the empty methods.For every method you have to complete, you are provided with a header, Do not modify those headers( method name, return type or parameters). You have to complete the body of the method. public void add(T afterThis, T info) The parameter info will be the information the new node should contain, and the new node will be added after the node that contains the parameter afterThis as an information public void removeFirst() Removes the first node in the linked list. Hint: be aware of empty lists. public void removeLast() Removes the last node in the linked list. Hint: be aware of empty lists. public int size() returns the size of the linked list. public boolean isEmpty() returns true if the linked list is empty, otherwise returns false. public boolean contains(T item) returns true if the list contains a node with the same information as the parameter of the method. Test your method with TestLinkedList, complete one method at the time and uncomment the method calls in the main method. package chapter02; public class LinkedList { protected LLNode list; public LinkedList() { list = null; } public void addFirst(T info) { LLNode node = new LLNode(info); node.setLink(list); list = node; } public void addLast(T info) { LLNode curr = list; LLNode newNode = new LLNode(info); if(curr == null) { list = newNode; } else { while(curr.getLink() != null) { curr = curr.getLink(); } curr.setLink(newNode); } } public void add(T afterThis, T info) { //TODO Complete this method as required in the homework instructions } public void removeFirst() { //TODO Complete this method as required in the homework instructions } public void removeLast() { //TODO Complete this method as required in the homework instructions } public int size() { //TODO Complete this method as required in the homework instructions } public boolean isEmpty() { //TODO Complete this method as required in the homework instructions } public boolean contains(T item) { //TODO Complete this method as required in the homework instructions } public void display() { LLNode currNode = list; while(currNode != null) { System.out.println(currNode.getInfo()); currNode = currNode.getLink(); } } }
You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add (must be completed) to add nodes to this linked list(I created one linked list in TestLinkedList). I’ve already created the class for you and have some completed method in there, your job is to complete the empty methods.For every method you have to complete, you are provided with a header, Do not modify those headers( method name, return type or parameters). You have to complete the body of the method. public void add(T afterThis, T info) The parameter info will be the information the new node should contain, and the new node will be added after the node that contains the parameter afterThis as an information public void removeFirst() Removes the first node in the linked list. Hint: be aware of empty lists. public void removeLast() Removes the last node in the linked list. Hint: be aware of empty lists. public int size() returns the size of the linked list. public boolean isEmpty() returns true if the linked list is empty, otherwise returns false. public boolean contains(T item) returns true if the list contains a node with the same information as the parameter of the method. Test your method with TestLinkedList, complete one method at the time and uncomment the method calls in the main method. package chapter02; public class LinkedList { protected LLNode list; public LinkedList() { list = null; } public void addFirst(T info) { LLNode node = new LLNode(info); node.setLink(list); list = node; } public void addLast(T info) { LLNode curr = list; LLNode newNode = new LLNode(info); if(curr == null) { list = newNode; } else { while(curr.getLink() != null) { curr = curr.getLink(); } curr.setLink(newNode); } } public void add(T afterThis, T info) { //TODO Complete this method as required in the homework instructions } public void removeFirst() { //TODO Complete this method as required in the homework instructions } public void removeLast() { //TODO Complete this method as required in the homework instructions } public int size() { //TODO Complete this method as required in the homework instructions } public boolean isEmpty() { //TODO Complete this method as required in the homework instructions } public boolean contains(T item) { //TODO Complete this method as required in the homework instructions } public void display() { LLNode currNode = list; while(currNode != null) { System.out.println(currNode.getInfo()); currNode = currNode.getLink(); } } }
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 18RQ
Related questions
Question
You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add (must be completed) to add nodes to this linked list(I created one linked list in TestLinkedList).
I’ve already created the class for you and have some completed method in there, your job is to complete the empty methods.For every method you have to complete, you are provided with a header, Do not modify those headers(
method name, return type or parameters). You have to complete the body of the method.
public void add(T afterThis, T info)
The parameter info will be the information the new node should contain, and the new node will be added after the node that contains the parameter afterThis as an information
public void removeFirst()
Removes the first node in the linked list. Hint: be aware of empty lists.
public void removeLast()
Removes the last node in the linked list. Hint: be aware of empty lists.
public int size()
returns the size of the linked list.
public boolean isEmpty()
returns true if the linked list is empty, otherwise returns false.
public boolean contains(T item)
returns true if the list contains a node with the same information as the parameter of the method.
Test your method with TestLinkedList, complete one method at the time and uncomment the method calls in the main method.
package chapter02;
public class LinkedList
{
protected LLNode list;
public LinkedList()
{
list = null;
}
public void addFirst(T info)
{
LLNode node = new LLNode(info);
node.setLink(list);
list = node;
}
public void addLast(T info)
{
LLNode curr = list;
LLNode newNode = new LLNode(info);
if(curr == null)
{
list = newNode;
}
else
{
while(curr.getLink() != null)
{
curr = curr.getLink();
}
curr.setLink(newNode);
}
}
public void add(T afterThis, T info)
{
//TODO Complete this method as required in the homework instructions
}
public void removeFirst()
{
//TODO Complete this method as required in the homework instructions
}
public void removeLast()
{
//TODO Complete this method as required in the homework instructions
}
public int size()
{
//TODO Complete this method as required in the homework instructions
}
public boolean isEmpty()
{
//TODO Complete this method as required in the homework instructions
}
public boolean contains(T item)
{
//TODO Complete this method as required in the homework instructions
}
public void display()
{
LLNode currNode = list;
while(currNode != null)
{
System.out.println(currNode.getInfo());
currNode = currNode.getLink();
}
}
}
![package chapter02;
public class TestLinkedList
(
public static void main(String[] args)
(
LinkedList<String> list = new LinkedList<String>();
System.out.println("Empty list? " + list.isEmpty());
System.out.println();
list.addLast("Smith, John");
list.addLast("Lee, Kelvin");
list.addLast("Davids, Tim");
list.addLast("Willis, Linda");
list.addLast("Wilton, Lisa");
list.add("Lee, Kelvin", "Hunter, Roy");
list.display();
System.out.println();
System.out.println(list.contains ("Willis, Linda"));
System.out.println();
list.removeFirst();
System.out.println("After remove first: ");
list.display();
System.out.println();
list.removeLast();
System.out.println("After remove last: ");
list.display();
System.out.println();
"
System.out.println("Size: + list.size());](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F00e22777-512f-4c60-a1c3-c275cee7ac4e%2F96d7d74a-aede-445d-a0f5-fef3f60b92b9%2F0j0gdvu_processed.jpeg&w=3840&q=75)
Transcribed Image Text:package chapter02;
public class TestLinkedList
(
public static void main(String[] args)
(
LinkedList<String> list = new LinkedList<String>();
System.out.println("Empty list? " + list.isEmpty());
System.out.println();
list.addLast("Smith, John");
list.addLast("Lee, Kelvin");
list.addLast("Davids, Tim");
list.addLast("Willis, Linda");
list.addLast("Wilton, Lisa");
list.add("Lee, Kelvin", "Hunter, Roy");
list.display();
System.out.println();
System.out.println(list.contains ("Willis, Linda"));
System.out.println();
list.removeFirst();
System.out.println("After remove first: ");
list.display();
System.out.println();
list.removeLast();
System.out.println("After remove last: ");
list.display();
System.out.println();
"
System.out.println("Size: + list.size());
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
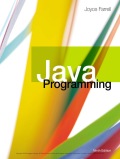
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
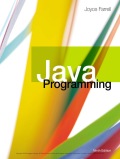
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT