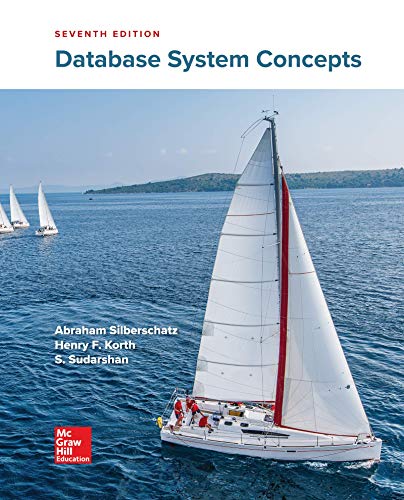
You are given the heads of two sorted linked lists list1 and list2.
Merge the two lists in a one sorted list. The list should be made by splicing together the nodes of the first two lists.
Return the head of the merged linked list.
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode() : val(0), next(nullptr) {}
* ListNode(int x) : val(x), next(nullptr) {}
* ListNode(int x, ListNode *next) : val(x), next(next) {}
* };
*/
class Solution {
public:
ListNode* mergeTwoLists(ListNode* list1, ListNode* list2) {
//PLACE YOUR CODE HERE
}
};
Example:
Input: list1 = [1,2,4], list2 = [1,3,4] Output: [1,1,2,3,4,4]

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- ASSUMING C LANGUAGE True or False: You can have the data portion of a Linked List be a Struct containing a Linked List itselfarrow_forwardQuestion 2: Linked List Implementation You are going to create and implement a new Linked List class. The Java Class name is "StringLinkedList". The Linked List class only stores 'string' data type. You should not change the name of your Java Class. Your program has to implement the following methods for the StringLinked List Class: 1. push(String e) - adds a string to the beginning of the list (discussed in class) 2. printList() prints the linked list starting from the head (discussed in class) 3. deleteAfter(String e) - deletes the string present after the given string input 'e'. 4. updateToLower() - changes the stored string values in the list to lowercase. 5. concatStr(int p1, int p2) - Retrieves the two strings at given node positions p1 and p2. The retrieved strings are joined as a single string. This new string is then pushed to the list using push() method.arrow_forwardplease go in to the detail and explain the purpose and function to each line & function of code listed below. #include <stdio.h> //Represent a node of the singly linked list struct node{ int data; struct node *next; }; //Represent the head and tail of the singly linked list struct node *head, *tail = NULL; //addNode() will add a new node to the list void addNode(int data) { //Create a new node struct node *newNode = (struct node*)malloc(sizeof(struct node)); newNode->data = data; newNode->next = NULL; //Checks if the list is empty if(head == NULL) { //If list is empty, both head and tail will point to new node head = newNode; tail = newNode; } else { //newNode will be added after tail such that tail's next will point to newNode tail->next = newNode; //newNode will become new tail of the list tail = newNode; } }…arrow_forward
- C++The List class represents a linked list of dynamically allocated elements. The list has only one member variable head which is a pointer that leads to the first element. See the following code for the copy constructor to List. List (const List & list) { for (int i = 0; i <list.size (); i ++) { push_back (list [i]); } } What problems does the copy constructor have? Select one or more options: 1. List (const List & list) {} does not define a copy constructor. 2. The list parameter should not be constant. 3. The new elements will be added to the wrong list. 4. Copy becomes shallow rather than deep. 5. The copy will create dangling pointers. 6. Copying will create memory leaks. 7. The condition must be: i <size () 8. head is never initialized.arrow_forwardIn c++, how do I display the last node in a linked list?arrow_forwardC++arrow_forward
- struct insert_at_back_of_sll { // Function takes a constant Book as a parameter, inserts that book at the // back of a singly linked list, and returns nothing. void operator()(const Book& book) { /// TO-DO (3) /// // Write the lines of code to insert "book" at the back of "my_sll". Since // the SLL has no size() function and no tail pointer, you must walk the // list looking for the last node. // // HINT: Do not attempt to insert after "my_sll.end()". // ///// END-T0-DO (3) ||||// } std::forward_list& my_sll; };arrow_forwardEvery time you write a non-const member function for a linked list, you should always think about if that function is preserving your class invariants. Group of answer choices A. True B. Falsearrow_forwardT/F: All Linked Lists must have head node.arrow_forward
- 1)Write a Java program which stores three values by using singly linked list.- Node class1. stuID, stuName, stuScore, //data fields2. constructor3. update and accessor methods- Singly linked list class which must has following methods:1. head, tail, size// data fields2. constructor3. update and accessor methodsa. getSize() //Returns the number of elements in the list.b. isEmpty( ) //Returns true if the list is empty, and false otherwise.c. getFirstStuID( ), getStuName( ), getFirstStuScore( )d. addFirst(stuID, stuName, stuScore)e. addLast(stuID, stuName, stuScore)f. removeFirst ( ) //Removes and returns the first element of the list.g. displayList( ) //Displays all elements of the list by traversing the linked list.- Test class – initialize a singly linked list instance. Test all methods of singly linked list class. 2. Write a Java program which stores three values by using doubly linked list.- Node class4. stuID, stuName, stuScore, //data fields5. constructor6. update and…arrow_forwardExplain the differences between a statically allocated array, a dynamically allocated array, and a linked list.arrow_forwardstruct node{int num;node *next, *before;};start 18 27 36 45 54 63 The above-linked list is made of nodes of the type struct ex. Your task is now to Write a complete function code to a. Find the sum of all the values of the node in the linked list. b. Print the values in the linked list in reverse order. Use a temporary pointer temp for a and b. i dont need a full code just the list partarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
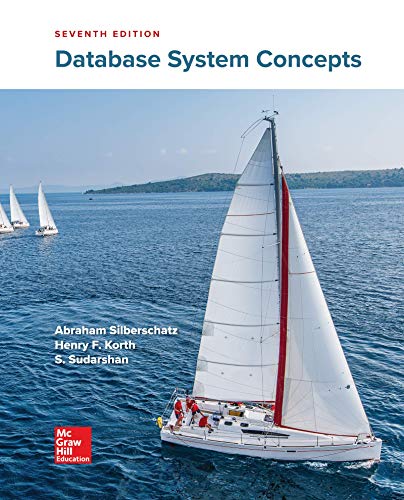
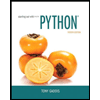
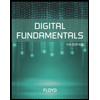
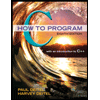
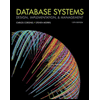
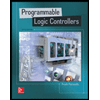