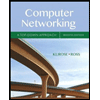
Can someone please help me with the following coding given based on the instructions (//*** in bold)? I am not sure on how to go about it here. Just as a side note, this is coding that is going to be used for a working Yahtzee program. I am also using the IntelliJ IDEA application for this code. Thanks for the help!
//***
//*** INSTRUCTIONS FOR CODE FOR YOU TO WRITE
//***
//*** 1) Replace these six if-else structures with the code in Steps 2 & 3:
//***
//*** if (aces == SCORE_NO_VALUE)
//*** System.out.println(FIRST_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + ACES_LABEL);
//*** else
//*** System.out.println(FIRST_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + ACES_LABEL + EQUALS_LABEL + aces);
//***
//*** if (twos == SCORE_NO_VALUE)
//*** System.out.println(SECOND_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + TWOS_LABEL);
//*** else
//*** System.out.println(SECOND_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + TWOS_LABEL + EQUALS_LABEL + twos);
//***
//*** if (threes == SCORE_NO_VALUE)
//*** System.out.println(THIRD_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + THREES_LABEL);
//*** else
//*** System.out.println(THIRD_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + THREES_LABEL + EQUALS_LABEL + threes);
//***
//*** if (fours == SCORE_NO_VALUE)
//*** System.out.println(FOURTH_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + FOURS_LABEL);
//*** else
//*** System.out.println(FOURTH_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + FOURS_LABEL + EQUALS_LABEL + fours);
//***
//*** if (fives == SCORE_NO_VALUE)
//*** System.out.println(FIFTH_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + FIVES_LABEL);
//*** else
//*** System.out.println(FIFTH_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + FIVES_LABEL + EQUALS_LABEL + fives);
//***
//*** if (sixes == SCORE_NO_VALUE)
//*** System.out.println(SIXTH_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + SIXES_LABEL);
//*** else
//*** System.out.println(SIXTH_OPTION_LABEL + OPTION_SUFFIX_ONE_DIGIT + SIXES_LABEL + EQUALS_LABEL + sixes);
//***
//*** 2) In place of the code in Step 1, write a for-loop that iterates "number of items in upper section category" times.
//***
//*** Hint: There is a constant already defined for "number of items in upper section category" that you must use.
//***
//*** 3) Code block for the for-loop in Step 2:
//*** A) Using the for-loop's control variable as an index into array variable "scores", write an if-statement that checks the value of each upper category score element in the array variable "scores" for "equality to" SCORE_NO_VALUE.
//*** B) If the condition in Step 3A evaluates to true, then the category has not been used, therefore do the following:
//*** a) Code block if Step 3A evaluates to true:
//*** 1) Write a println statement that displays the menu option using the for-loop's control variable in displaying both the menu number and the associated value in array constant "labels".
//*** b) Code block when Step 3A evaluates to false (else-statement):
//*** 1) Write a println statement that displays the menu option using the for-loop's control variable in displaying: the menu number, the associated value in array constant "labels", and the associated score value stored in array variable "scores".
//***
//*** NOTE: 1) Array variable "scores" replaces all of the individual score variables (e.g. aces, twos, etc).
//*** 2) Array constant "labels" replaces all of the individual label constants (e.g. ACES_LABEL, TWOS_LABEL, etc).
//*** 3) You are replacing the six if-else structures that use the individual score variables with a for-loop that contains a nested if-else structure that references into array variable "scores" and array constant "labels".
//***
//*** This can be as few as five lines of code not including any curly braces.
//***

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- This is a debugging question I am stuck on - The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. I have edited some of the code but I am unsure how to fix the rest - // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'}; int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter";…arrow_forwardplease do fast i will give you thumbs uparrow_forwardCoding in python Please!!I need help please help me in the easiest way to do this coding question. Please do not post it's answer from chegg just use your own skills to write code in easyiest way possible. I will really appriciate your help. Coding language PYTHON : The purpose of this assignment is to help you get comfortable creating and using simple classes and objects. In this assignment, we wish to model a 2-D video game character’s movements. In particular, suppose we are creating a tiny game, where the character can occupy one of 25 squares, like so: The first thing we need to keep track of is the character’s name, so we can differentiate this character from other characters or enemies. The other thing we need to keep track of is the character’s position, i.e., which square they are in. In order to do this, we’ll use an X-Y coordinate system with the origin in the top left corner (the 0,0 square in the picture). So, in the picture, the character occupies square 3, 2;…arrow_forward
- 2. I have provided the code I have written. All I need you to do is to rewrite the code. I need you to rewrite the comments, rename all the variables, and shift the code. It should look different once you have edited it. I would like to see another format for this code. It is in C-Programming. I think it shouldn’t take so long. Take your time, please! I really appreciate any help you can provide! CODE STARTS HERE: #include<stdio.h>#include<string.h>#include<stdlib.h> int main(int argc, char* argv[]){ printf("Entered String is: %s\n", argv[1]); const char *original_str = argv[1]; int i = 0, j = 0; int err_flag = 0; char num_arry[10] = {'0','1','2','3','4','5','6','7','8','9'}; /*if string conatins a digit in it return error, simple linear search has been used*/ for(i=0;i<10;i++){ for(j=0;j<strlen(original_str);j++){ if(original_str[j] == num_arry[i]){ printf("error\n"); err_flag = 1;…arrow_forwardThe class I'm taking is assembly programming. I have attached the instructions. i have also attached my work. Can you please tell me how to fix it so I can successfully run and compile program in visual studio . Thank you so much for your help!arrow_forwardI am learning C++ and would like to make cleaner code. I am learning on Udemy.com how having the main at the bottom of the program helps when making blocks of code. What I would like to do is take some code I already have, and have the user enter two prime colors to make a mixed color, then ask the user if they would like to mix another color with two of the same prime and one other prime color to get: yellow-green, yellow-orange, orange-red, red-purple, blue-green, and blue-purple. Is my logic flawed? What is the correct logic? How would I set up this code to have different functions outside the main with the main at the bottom? I know it is supposed to read the information first somehow. I just can't process how to write it. #include <iostream>#include <string> using namespace std; int main() { string arr[] = {"red", "blue", "yellow"}; int color1, color2; string mixedColor; char choice; do { cout << "Enter one prime color followed by a second…arrow_forward
- Please help me with these question. I am having trouble understanding what to do. Please use HTML, CSS, and JavaScript Thank youarrow_forwardThe class I'm taking is assembly programming. I have attached the instructions. i have also attached my work. Can you please tell me how to fix it so I can successfully run and compile program in visual studio . i keep getting syntax errors on line 11 and 12. Thank you so much for your help!arrow_forwardC++ Programming I would like to make this code like the result(photo submitted). And please let me know how I can open it on notepad. ----------------------------------------------------------------- Code: #include<iostream>#include<fstream>using namespace std;int main();int taxi_num=5; int tx1_call=0,tx1_pass=0,tx2_call=0,tx2_pass=0, // Creating global variablestx3_call=0,tx3_pass=0,tx4_call=0,tx4_pass=0,tx5_call=0,tx5_pass=0; int total_passangers=0; // Total passanger count char NeedTaxi(){if(taxi_num<1) taxi_num=5;char ans; cout<<"\n\nDo you need Taxi (Y/N) : ";cin>>ans;if(ans!='Y' && ans!='N') {cout<<"\n\nError entry !\n\n"; main();}if(ans=='N') return ans;cout<<"Taxi #"<<taxi_num<<" will pick you up in a few minutes."; if(taxi_num==5) {tx5_call++; tx5_pass+=3;} // Add 3 passangers everytime taxi 5 calledif(taxi_num==4) {tx4_call++; tx4_pass+=5;} // Add 5 passangers everytime taxi 4 calledif(taxi_num==3) {tx3_call++;…arrow_forward
- I need help making this program in C programming. Please look at the pictures provided at that helps. Thank You!arrow_forwardUsing p5* JavaScript on "openprocessing" websitearrow_forwardPS: Please answer number 1 and 2 as they are all linked altogether with the subparts by following the instructions perfectly and not confusing. Write a Java Program with an intentional error in it. 1. Your program must include Loops and Arrays with a breakpoint 2. Once you have created your program, debug the application in eclipse to fix the error. a. Ensure that you take at least 3 screenshots of the debugging process and provide a brief explanation of the step. b. Ensure you use breakpoints and submit a snapshot labelling it. c. Submit all steps.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
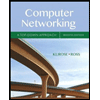
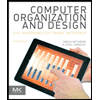
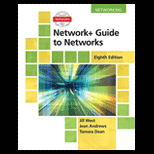
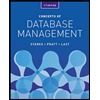
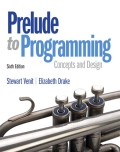
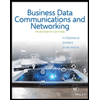