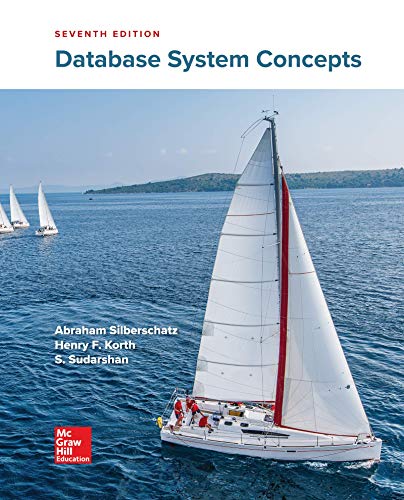
Include the code to any h files used
Write two c++ programs to implement a distributed version of a multithreaded Huffman decompressor using the one in project 1
The server
The user will execute this program using the following syntax:
./exec_filename port_no < input_filename
where exec_filename is the name of your executable file, port_no is the port number to create the socket, and input_filename is the name of the file with the alphabet's information. The port number will be available to the server program as a command-line argument.
The server program receives from STDIN the alphabet's information (using input redirection). The input file has multiple lines, where each line contains information (character and frequency) about a symbol from the alphabet. The input file format is as follows:
A char representing the symbol.
An integer representing the frequency of the symbol.
Example Input File:
E 3
G 3
F 1
H 2
Given the previous input file, the expected output for the server program is:
Symbol: G, Frequency: 3, Code: 0
Symbol: F, Frequency: 1, Code: 100
Symbol: H, Frequency: 2, Code: 101
Symbol: E, Frequency: 3, Code: 11
The client program:
The user will execute this program using the following syntax:
./exec_filename hostname port_no < compressed_filename
where exec_filename is the name of your executable file, hostname is the address where the server program is located, port_no is the port number used by the server program, and compressed_filename is the name of the compressed file. The hostname and the port number will be available to the client as command-line arguments.
The client program receives from STDIN (using input redirection) m lines (where m is the number of symbols in the alphabet). Each line of the compressed file has the following format:
A string representing the binary code of the symbol.
A list of n integers (where n is the frequency of the symbol) representing the positions where the symbol appears in the message.
Example Compressed File:
11 1 3 5
0 0 2 4
101 6 8
100 7
Given the previous compressed, the expected output is:
Original message: GEGEGEHFH
Notes:
You can safely assume that the input files will always be in the proper format.
You must use the output statement format based on the example above.
For the client program, you must use POSIX Threads and stream sockets.
You must use multiple processes (fork) and stream sockets for the server program.
project 1 code:
https://replit.com/@jacksmith-2023/PA1
input and output
usage: ./server 8080 < input1.txt
Input for the Server:
E 3
G 3
F 1
H 2
usage: ./client local host 8080 < compressed1.txt
Input for the Client:
11 1 3 5
0 0 2 4
101 6 8
100 7
Expected Output
Symbol: G, Frequency: 3, Code: 0
Symbol: F, Frequency: 1, Code: 100
Symbol: H, Frequency: 2, Code: 101
Symbol: E, Frequency: 3, Code: 11
Original message: GEGEGEHFH

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- Unix Question: Only some of the EMACS code is complete. Observe the comments to complete the EMACS code. The program enables you to document payroll information: Surname Forename Sector How many hours worked The code writes the information into a file named “measurecard” The user is asked to attach another “s” (for sure) or “l” to leave Please see the EMACS code below: # ================================================================== # Script Name: Measurecard # By: Anonymous # Date: April 26, 2022 # # Command Line: measurecard # ==================================================================measurefile=measurefilebendtrial="s"attachanother = “s”while [ $bendtrial = s ]do###### empty the mesh### tput cup 1 4; echo "Workers' Worktime" tput cup 2 4; echo "==============================" tput cup 4 4; echo "Type l to leave or s to attach: " tput cup 5 4; echo "Surname : "###### tput cup Forename: ### tput cup "Sector Number: "### tput cup "Hours…arrow_forwardThe ObjectOutputStream class is used to serialize an Object. The following Serialize Demo program instantiates an Employee object and serializes it to a file. Complete the code. Write codes where asked with appropriate try catch block class SDemo { } main method { } //Create an Employee object //set a name for this employee //set employee number to 1010 //create an object of file output stream //create an object of the object output stream //write Employee info to this object of output stream //close the out stream //close the file //print statementarrow_forwardPython S3 Get File In the Python file, write a program to get all the files from a public S3 bucket named coderbytechallengesandbox. In there there might be multiple files, but your program should find the file with the prefix and cb - then output the full name of the file. You should use the boto3 module to solve this challenge. You do not need any access keys to access the bucket because it is public. This post might help you with how to access the bucket. Example Output ob name.txt Browse Resources Search for any help or documentation you might need for this problem. For exampler array indexing, Ruby hash tables, etc.arrow_forward
- Write a function named repeat_words that takes two string parameters: 1. in_file: the name of an input file that exists before repeat_words is called 2. out_file: the name of an output file that repeat_words creates Assume that the input file is in the current working directory and write the output file to that directory. For each line of the input file, the function repeat_words should write to the output file all of the words that appear more than once on that line. Each word should be lower cased and stripped of leading and trailing punctuation. Each repeated word on a line should be written to the corresponding line of the output file only once, regardless of the number of times the word is repeated. For example, if the following is the content of the file catInTheHat.txt: Too wet to go out and too cold to play ball. So we sat in the house. We did nothing at all. So all we could do was to Sit! Sit! Sit! Sit! The following function call: inF = 'catInTheHat.txt' outF =…arrow_forwardin c# i need to Write the program named DirectoryInformation that allows a user to continually enter directory names until the user types end. If the directory name exists, display a list of the files in it; otherwise, display the following message, Directory foo does not exist, to indicate the directory does not exist (foo would be the name of the directory to be checked) . Create as many test directories and files as necessary to test your program. An example of the program is shown below: Enter a directory >> lorem lorem contains the following files lorem/ipsum.txt Enter another directory or type end to quit >> foo Directory foo does not existarrow_forwardThe Payroll Department keeps a list of employee information for each pay period in a text file. The format of each line of the file is the following: <last name> <hours worked> <hourly wage> Write a program that inputs a filename from the user and prints to the terminal a report of the wages paid to the employees for the given period. The report should be in tabular format with the appropriate header. Each line should contain: An employee’s name The hours worked The wages paid for that period. An example of the program input and output is shown below:arrow_forward
- Unix Commands Using the Unix commands, you have learned in class, create the following file structure: • Create a directory called "unix_hw" in your home directory. • Within "unix_hw", create a file called "helloworld.c" and a directory called "output". • Compile your helloworld.c file into output folder. This should create an output executable file in output folder. • Run the helloworld program from output folder. • Within "output", create a file called "notes.txt". • Move "notes.txt" to "unix_hw" (using relative path) • Remove "notes.txt" from output folder. (using absolute path) • Remove "output" (using absolute path) Perform above steps on your Unix account, then copy each command your ran into a text file and take a screenshot of your terminal window such that all steps are visible.arrow_forwardThe Payroll Department keeps a list of employee information for each pay period in a text file. The format of each line of the file is the following: <last name> <hours worked> <hourly wage> Write a program that inputs a filename from the user and prints to the terminal a report of the wages paid to the employees for the given period. The report should be in tabular format with the appropriate header. Each line should contain: An employee’s name The hours worked The wages paid for that period. An example of the program input and output is shown below: Enter the file name: data.txt Name Hours Total Pay Lambert 34 357.00 Osborne 22 137.50 Giacometti 5 503.50arrow_forwardInclude the code to any h files usedWrite two c++ programs to implement a distributed version of a multithreaded Huffman decompressor using the one in project 1 The server program The user will execute this program using the following syntax: ./exec_filename port_no < input_filename where exec_filename is the name of your executable file, port_no is the port number to create the socket, and input_filename is the name of the file with the alphabet's information. The port number will be available to the server program as a command-line argument. The server program receives from STDIN the alphabet's information (using input redirection). The input file has multiple lines, where each line contains information (character and frequency) about a symbol from the alphabet. The input file format is as follows: A char representing the symbol. An integer representing the frequency of the symbol. Example Input File: E 3 G 3 F 1 H 2 Given the previous input file, the expected output for the…arrow_forward
- This is using basic C and Linux System Callsarrow_forwardThe Directory class has a list of names in it. You should use dynamic memory to create anarray upon instantiation. You should create a copy constructor for the directory class and anassignment operator (i.e. operator=()). You should create a function called fillDirectory whichaccepts a size for the directory and the names for it both of which should be given by the user.In main, create directory1 and fill it with information. Use the assignment operator=() to setdirectory2 equal to directory1. This is in c++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
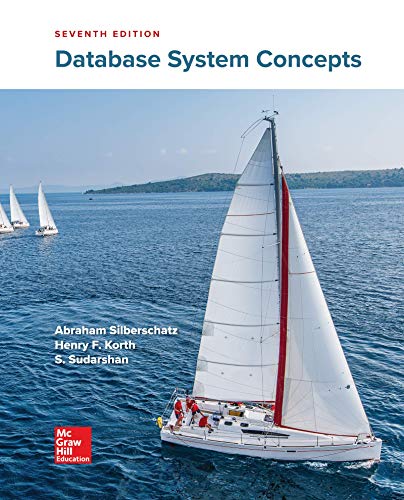
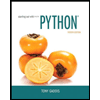
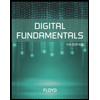
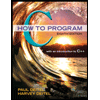
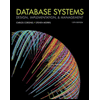
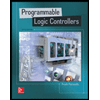