Write the method named withoutString(). * * Given two strings, base and remove, return a * version of the base string where all instances * of the remove string have been removed (not case * sensitive). You may assume that the remove * string is length 1 or more. Remove only non-overlapping * instances, so with "xxx" removing "xx" leaves "x". *
Use any loops in Java Language
Write the method named withoutString().
*
* Given two strings, base and remove, return a
* version of the base string where all instances
* of the remove string have been removed (not case
* sensitive). You may assume that the remove
* string is length 1 or more. Remove only non-overlapping
* instances, so with "xxx" removing "xx" leaves "x".
*
* Note: Despite the term "remove" you should actually not
* try to remove the characters from a string. You'll continue
* to use the "building a string" pattern from lecture.
*
* Examples:
* withoutString("Hello there", "llo") returns "He there"
* withoutString("Hello there", "e") returns "Hllo thr"
* withoutString("Hello there", "x") returns "Hello there"
*
* @param base the base String to modify.
* @param remove the substring to remove.
* @return the base String with all copies of remove removed.
*/
// TODO - Write the withoutString method here
/**
* Write the method named sameEnds().
*
* Given a string, return the longest substring
* that appears at both the beginning and end of
* the string without overlapping. For example,
* sameEnds("abXab") should return "ab".
*
* Examples:
* sameEnds("abXYab") returns "ab"
* sameEnds("xx") returns "x"
* sameEnds("xxx") returns "x"
*
* @param str the String to search.
* @return the longest substring that appears at beginning and end.
*/
// TODO - Write the sameEnds method here.
/**
* Write the method named maxBlock().
*
* Given a string, return the length of the
* largest "block" in the string. A block is a run
* of adjacent characters that are the same.
*
* Examples:
* maxBlock("hoopla") returns 2
* maxBlock("abbCCCddBBBxx") returns 3
* maxBlock("") returns 0
*
* @param str the String to examine.
* @return the size of the longest run of adjacent characters.
*/
// TODO - Write the maxBlock method here.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

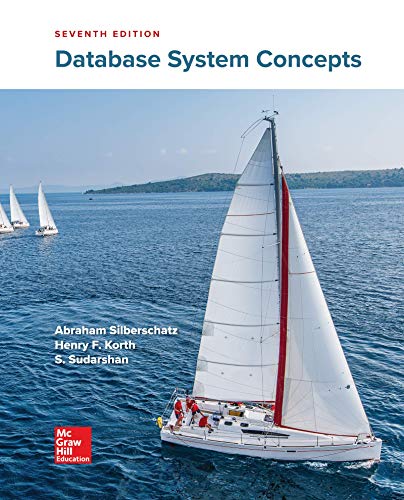
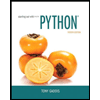
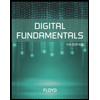
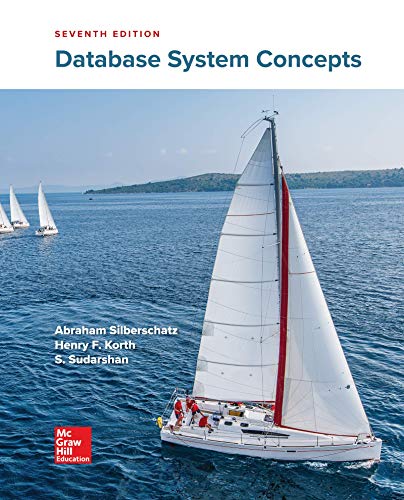
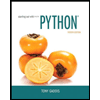
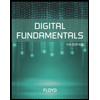
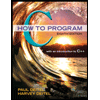
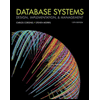
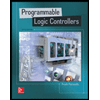