Write the java code corresponding to the following UML diagram: Teacher Indications: name: String age: int salary: float Teacher(n:String, a:int, float s) // get + set methods print():void Person(name:String, age:int) // get + set methods for each attributes print():void(abstract) Tab[]: Person size Tab:int n:int Person (Abstract) University id: int Person is an abstract class containing the abstract method Print.() In Teacher and Student the method print() will print the information of either Teacher or Student. University (size Tab) void addPerson(p:Person) printAll():void Student Write the class university corresponding to the following UML diagram. It contains three attributes. Tab is an array of Person. sizeTab is the size of Tab. The attribute n represents the number of elements added to the tab. When the tab is empty n is equal to 0. The constructor University (size Tab) will initialize the different attributes and allocate the memory to the array Tab. addPerson(p:Person) will add the new person to Tab. Student(name:String, age:int,id:int) // get + set methods print():void The method printAll() will print all the information of the Persons inside Tab. Write the main method. Create three Teacher and three Student and add them to University Object and print them all.
Indications:
Person is an abstract class containing the abstract method Print().
In Teacher and Student the method print() will print the information of either Teacher or Student.
Write the class university corresponding to the following UML diagram. It contains three attributes.
Tab is an array of Person. sizeTab is the size of Tab. The attribute n represents the number of elements added to the tab. When the tab is empty n is equal to 0.
The constructor University(sizeTab) will initialize the different attributes and allocate the memory to the array Tab.
addPerson(p:Person) will add the new person to Tab.
The method printAll() will print all the information of the Persons inside Tab.
Write the main method. Create three Teacher and three Student and add them to University Object and print them all.
![Write the java code corresponding to the following UML diagram:
Teacher
Indications:
salary: float
Teacher(n:String, a:int, float s)
// get + set methods
print():void
name: String
age: int
Person(name:String, age:int)
// get + set methods for each attributes
print():void(abstract)
Tab[]: Person
size Tab:int
n:int
University
Person (Abstract)
printAll():void
Person is an abstract class containing the abstract method Print.()
In Teacher and Student the method print() will print the information of either Teacher or
Student.
University (size Tab)
void addPerson(p:Person)
id: int
Write the class university corresponding the llowing UML diagram. It contains three
attributes.
Tab is an array of Person. sizeTab is the size of Tab. The attribute n represents the number
of elements added to the tab. When the tab is empty n is equal to 0.
The constructor University (size Tab) will initialize the different attributes and allocate the
memory to the array Tab.
add Person (p:Person) will add the new person to Tab.
Student
Student(name:String, age:int,id:int)
// get + set methods
print():void
The method printAll() will print all the information of the Persons inside Tab.
Write the main method. Create three Teacher and three Student and add them to University
Object and print them all.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcbbe4657-9f5f-4004-9833-35081028474b%2Fd7651552-98ea-4983-87e3-bd37c877b5d6%2F4mpm3kg_processed.jpeg&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

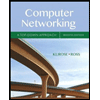
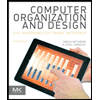
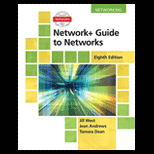
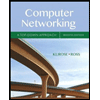
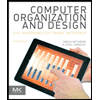
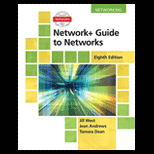
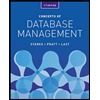
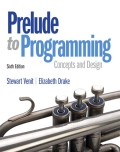
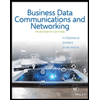