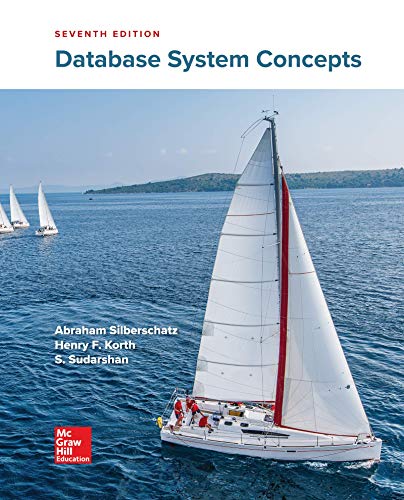
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Write a simple C++ program to simulate the insertion program.
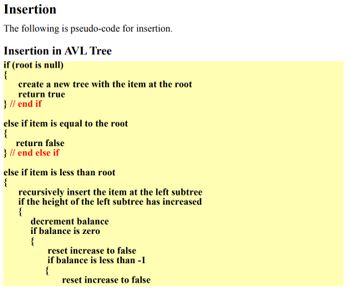
Transcribed Image Text:### Insertion
The following is pseudo-code for insertion.
#### Insertion in AVL Tree
```plaintext
if (root is null)
{
create a new tree with the item at the root
return true
} // end if
else if item is equal to the root
{
return false
} // end else if
else if item is less than root
{
recursively insert the item at the left subtree
if the height of the left subtree has increased
{
decrement balance
if balance is zero
{
reset increase to false
}
if balance is less than -1
{
reset increase to false
}
}
}
```
This pseudocode is part of a larger algorithm used to insert elements into an AVL tree, which is a type of self-balancing binary search tree. The primary operations performed in this pseudocode include:
1. **Checking if the root is null:**
- If it is, a new tree is created with the item as the root, and the function returns `true`.
2. **Comparing the item to the root:**
- If the item is equal to the root, the function returns `false`, indicating the item already exists in the tree.
3. **Recursive insertion for items less than the root:**
- If the item is less than the root, it is recursively inserted into the left subtree.
- If the height of the left subtree increases as a result of the insertion, the balance factor is adjusted accordingly.
- If necessary, adjustments are made to maintain the AVL tree property, namely, that the balance factor remains between -1 and 1. If the balance factor goes out of this range, further balancing operations (not shown in the provided pseudocode) would be necessary.
These steps ensure that the AVL tree remains balanced after each insertion, providing efficient performance for search, insertion, and deletion operations.
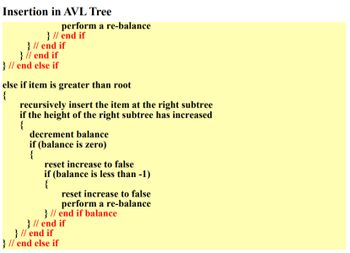
Transcribed Image Text:### Insertion in AVL Tree
#### Algorithm Steps
1. **Determine Position for Insertion:**
- **If the item is greater than the root:**
- Recursively insert the item into the right subtree.
- If the height of the right subtree has increased:
- Decrement the balance.
- If the balance is zero:
- Reset `increase` to `false`.
- If the balance is less than -1:
- Reset `increase` to `false`.
- Perform a re-balance.
```plaintext
if item is greater than root
{
recursively insert the item at the right subtree
if the height of the right subtree has increased
{
decrement balance
if (balance is zero)
{
reset increase to false
if (balance is less than -1)
{
reset increase to false
perform a re-balance
} // end if balance
} // end if
} // end if
} // end else if
```
In an AVL tree, insertion follows defined steps to ensure the tree remains balanced after every insertion. When inserting:
- If the new item is greater than the root, it will be inserted into the right subtree.
- After the item is inserted, the height of the right subtree may increase.
- If this causes the height of a subtree to change, the balance factor is adjusted. Depending on the balance factor, the tree may need re-balancing to ensure efficient look-up times in the tree.
Understanding and following these steps is crucial for maintaining the balanced nature of an AVL tree, which is an essential aspect of many search operations in computer science.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
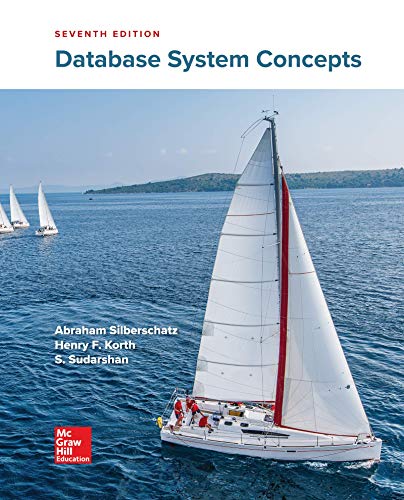
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
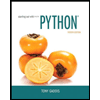
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
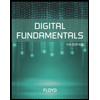
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
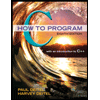
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
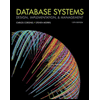
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
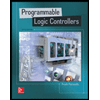
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education