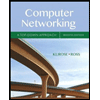
import java.util.Scanner;
public class LabProgram {
// Recursive method to draw the triangle
public static void drawTriangle(int baseLength, int currentLength) {
if (currentLength <= 0) {
return; // Base case: stop when currentLength is 0 or negative
}
// Calculate the number of spaces needed for formatting
int spaces = (baseLength - currentLength) / 2;
if (currentLength == baseLength) {
// If it's the first line, don't output spaces before the first '*'
System.out.println(" ".repeat(spaces) + "*".repeat(currentLength));
} else {
// Output spaces and asterisks
System.out.println(" ".repeat(spaces) + "*".repeat(currentLength));
}
// Recursively call drawTriangle with the reduced currentLength
drawTriangle(baseLength, currentLength - 2);
}
public static void drawTriangle(int baseLength) {
drawTriangle(baseLength, baseLength);
}
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int baseLength = scnr.nextInt();
drawTriangle(baseLength);
}
}
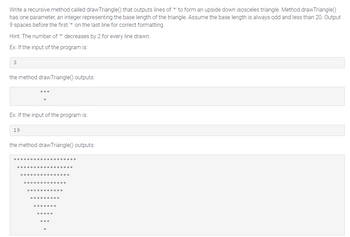
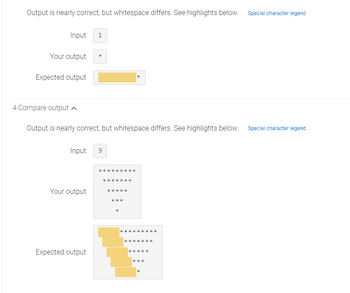

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Determine whether a string is a palindromeA palindrome is a string of characters that reads the same from right to left as it does from left to right, regardless of punctuation and spaces.The specifications for this assignment are: •Write and test a non-recursive solution in Java that determines whether a string is a palindrome •Your program should consist of at least two methods: (1) the main method (2) the method which performs the task of determining whether the specified string is a palindrome. You should name this method isPalindrome. You should name the class that contains your “main” method and the isPalindrome method FindPalindrome. •You must use a Stack and a Queue in your solution: Write your own Stack and Queue based on the Vector in the Java API and use those in your solution. You should name those classes StackVector and QueueVector respectively. You already have access to the relevant exception classes and interfaces for the above ADTs. •All of your belong to a Java…arrow_forwardCode 16-1 /** This class has a recursive method. */ public class EndlessRecursion { public static void message() { System.out.println("This is a recursive method."); message(); } } Code 16.2 /** This class has a recursive method message, which displays a message n times. */ public class Recursive { public static void messge (int n) { if (n>0) { System.out.println (" This is a recursive method."); message(n-1); } } } Task #1 Tracing Recursive Methods 1. Copy the file Recursion.java (see Code Listing 16.1) from the Student Files or as directed by your instructor. 2. Run the program to confirm that the generated answer is correct. Modify the factorial method in the following ways: a. Add these lines above the first if statement: int temp; System.out.println("Method call -- " + "calculating " + "Factorial of: " + n); Copyright © 2019 Pearson Education, Inc., Hoboken NJ b. Remove this line in the recursive section at the end of the method: return…arrow_forwardFix all errors to make the code compile and complete. //MainValidatorA3 public class MainA3 { public static void main(String[] args) { System.out.println("Welcome to the Validation Tester application"); // Int Test System.out.println("Int Test"); ValidatorNumeric intValidator = new ValidatorNumeric("Enter an integer between -100 and 100: ", -100, 100); int num = intValidator.getIntWithinRange(); System.out.println("You entered: " + num + "\n"); // Double Test System.out.println("Double Test"); ValidatorNumeric doubleValidator = new ValidatorNumeric("Enter a double value: "); double dbl = doubleValidator.getDoubleWithinRange(); System.out.println("You entered: " + dbl + "\n"); // Required String Test System.out.println("Required String Test:"); ValidatorString stringValidator = new ValidatorString("Enter a required string: "); String requiredString =…arrow_forward
- import java.util.Scanner; public class LabProgram { // Recursive method to draw the triangle public static void drawTriangle(int baseLength, int currentLength) { if (currentLength <= 0) { return; // Base case: stop when currentLength is 0 or negative } // Calculate the number of spaces needed for formatting int spaces = (baseLength - currentLength) / 2; if (currentLength == baseLength) { // If it's the first line, don't output spaces before the first '*' System.out.println("*".repeat(currentLength) + " "); } else { // Output spaces and asterisks System.out.println(" ".repeat(spaces) + "*".repeat(currentLength) + " "); } // Recursively call drawTriangle with the reduced currentLength drawTriangle(baseLength, currentLength - 2); } public static void drawTriangle(int baseLength) { drawTriangle(baseLength, baseLength); } public static…arrow_forwardpublic class main { public static void main(String [] args) { Dog dog1=new Dog(“Spark”,2),dog2=new Dog(“Sammy”,3); swap(dog1, dog2); System.out.println(“dog1 is ”+ dog1); System.out.println(“dog2 is ”+ dog2); } public static void swap(Dog a, Dog b) { String nameA = a.getName(); String nameB = b.getName(); a.setName(nameB); b.setName(nameA); } What is the output of the main()?arrow_forwardpublic class Main { public static void main(String[] args) { System.out.println("Your factorial is: " + factorial(9)); }} public static int factorial(int number) { if (number == 0) { return 1; } return number * factorial(number -1); } i need help fixing this code involving recursionarrow_forward
- write a code in java (using recursion)arrow_forwardusing System; class HelloWorld { static void Main() { int rows=21; int columns=76; for(int i=0;i<rows;i++) //for loop for printing the pattern for 21 rows { for(int j=1;j<=columns;j++) //for loop for printing the pattern in 76 columns { if((i+j)%7!=1 ) //if the addition of i and j does not give remainder of 1 when divided by 7 then Console.Write("-"); //print dash - on console else //otherwise peint a space on console Console.Write(" "); } Console.WriteLine(); //print a newline for printing on new row... } } }arrow_forwardConsider the following recursive method: Public static int Fib(int a1, int a2, int n){ if(n == 1) return a1; else if (n == 2) return a2;else return Fib(a1, a2, n-1) + Fib(a1, a2, n-2);} Please draw the recursion trace for Fib(2,3,5)arrow_forward
- write a code in java (using recursion)arrow_forwardI want solution with steparrow_forwardJava source code writing - a recursive algorithm. Please use non-recursive and recursive ways to determine if a string s is a palindrome, that is, it is equal to its reverse. Examples of palindromes include 'racecar' and 'gohangasalamiimalasagnahog'. Turn in your java source code file with three methods, including one main() method.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
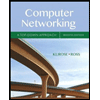
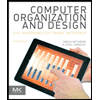
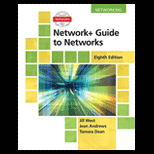
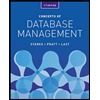
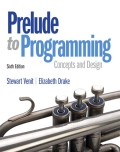
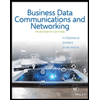