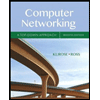
write a program whose input is a string wich contains a character and a phrase,and whos output indicates the number of times the character appears in the phrase.
ex: if the input is: n Monday
the output is:1
ex: if the input is: z Today is Monday
the output is: 0
ex: if the input is: n It's a sunny day
the output is: 2
Case Matters.
ex: if the input is: n Nobody
then the output is:0
n is different than N

Step-1: Start
Step-2: Declare a variable str and take input from user
Step-3: Declare a variable character and initialize with 0th index of str
Step-4: Declare a variable phrase and initialize with the substring of str from 1 to length of str
Step-5: Declare variable count and initialize with 0
Step-6: Start a loop from i=0 to length of phrase
Step-6.1: if i'th position of phrase is equal to character, increase count by 1
Step-7: Print count
Step-8: Stop
Step by stepSolved in 4 steps with 5 images

- Extend to cast the double to an integer, and output that integer. Enter integer: 99 Enter double: 3.77 Enter character: z Enter string: Howdy 99 3.77 z Howdy Howdy z 3.77 99 3.77 cast to an integer is 3arrow_forwardAlyssa is very particular about what she gets for dinner. The more hungry she is, the more she can not figure out what to eat! Write a short program that asks a few questions and then makes the correct suggestion based on the following rules: Alyssa considers any temperature below 50 to be cold and anything 50 and above to be warm. If it is cold and raining, Alyssa wants to have soup. If none of these rules apply, Alyssa will always have meatballs. Alyssa has to eat before class starts! When it is 15 minutes or less until class, she gets a sandwich. This is the most important rule. Your program should prompt the user with the following. Enter temperature (0 to 100): Enter weather (sunny, raining): Enter minutes until class starts (0 to 60): Below is sample input to test your program. 40 raining 30 Your program should output the following. soup Code Skeleton: import java.util.Scanner; public class Main { public static void main(String[] args) { Scanner scan = new…arrow_forwardWrite a program to calculate the net pay of an employee. Input the basic pay and calculate the net pay as follows: House rent is 45% of basic pay. Medical allowance is 2% of basic pay if basic pay is greater than Rs. 5000/-. It is 5% of basic pay if the basic pay is less than Rs. 5000/-. Conveyance allowance is Rs. 96/- if basic pay is less than Rs. 5000/-. It is Rs. 193/- if the basic pay is more than Rs. 5000/- Net pay is calculated by adding basic pay, medical allowance, conveyance allowance and house rant. using ooparrow_forward
- Write a program that takes in a line of text as input, and outputs that line of text in reverse. The program repeats, ending when the user enters "Done", "done", or "d" for the line of text. Ex: If the input is: Hello there Hey done then the output is: ereht olleH yeH 382800 2462264gayarrow_forwardQUESTION 7. Write a program that reads multiple integers from the user and finds the largest value. The program should ignore negative values and print a message indicating so. The sentinel is -1. If no valid input is entered, the program should print a message indicating so, like the second sample below. Use a flag variable that indicates whether valid input has been entered so far or not. Enter integer: 4 Enter integer: -5 >>> Invalid input! Enter integer: 20 Enter integer: 12 Enter integer: 7 Enter integer: -1 The largest value is: 20 Enter integer: -5 >>> Invalid input! Enter integer: -20 >>> Invalid input! Enter integer: -1 No valid input was entered!arrow_forwardRead string integer value pairs from input until "Done" is read. For each string read: • If the following integer read is less than 40, output the string followed by ": low on stock". • Otherwise, output the string followed by ": well stocked". End each output with a newline. Ex: If the input is Oven 43 Curtain 7 Pillow 1 Done, then the output is: Oven: well stocked Curtain: low on stock Pillow: low on stock 1 #include 2 #include 3 using namespace std; 4 5 int main() { 6 7 8 9 10 } * Your code goes here */ return 0;arrow_forward
- ogramming Languages home-335 LAB Mad Lib-loops Mad Libs are activities that have a person provide various words, which are then used to complete a short story in unexpected (and hopefully funny) ways Write a program that takes a string and an integer as input and outputs a sentence using the input values as shown in the example below The program repeats until the input string is quit and disregards the integer input that follows Ex If the input is apples 5 shoes 2 quit o the output is Eating 5 apples a day keeps you happy and healthy. Eating 2 shoes a day keeps you happy and healthy. LAB ACTIVITY B 9 3351 LAB Mad Lib-loops 2 #includearrow_forwardC Programming Lab For students with roll number ending with 5. Write a program to find the sum of the ASCII values of all uppercase alphabets and take the modulus of the sum with 26 and display the obtained value in the output.arrow_forwardStatistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the average and max. A negative integer ends the input and is not included in the statistics. Ex: When the input is 15 20 0 5 -1, the output is: 10 20 You can assume that at least one non-negative integer is input. Code need to be writing in coral languagearrow_forwardAlert dont submit AI generated answer.arrow_forwardplese code in python The midfix of 3 is the middle 3 characters of a string. Given a string input, output the middle three characters of that string. Assume the word length is always odd and at least three characters. Ex: If the input is: xxxtoyxxx the output is: Midfix: toyarrow_forwardProblem 2 Write a program that lets the user enter an integer number and display its equivalent value in text form. The range of input values should be from -99999 to 99999. Example 1 Enter integer in range [-99999, 99999] -> 100 Expected Output Text equivalence: one hundred Example 2 Enter integer in range [-99999, 99999] -> 5 Expected Output Text equivalence: five Example 3 Enter integer in range [-99999, 99999] -> 1812 Expected Output Text equivalence: one thousand eight hundred twelve Example 4 Enter integer in range [-99999, 99999] -> 44040 Expected Output Text equivalence: forty-four thousand forty Example 5 Enter integer in range [-99999, 99999] -> -10 Expected Output Text equivalence: negative ten In [40]:arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
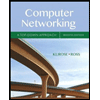
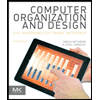
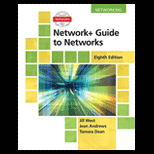
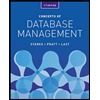
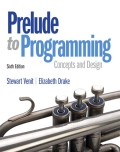
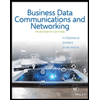