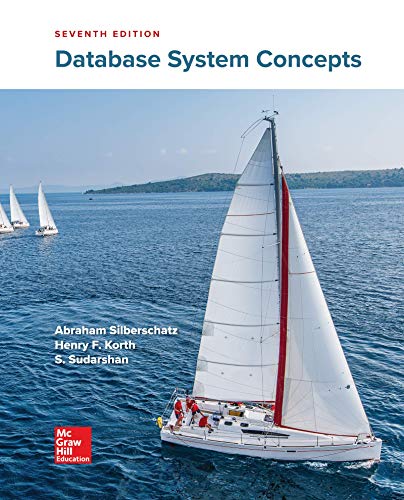
Write a
program should allow the user to repeat this calculation as often as the user wishes. Define a function to compute the number of miles per gallon. Your program should use a globally defined constant for the number of gallons per liter. Note: A liter is 0.264179 gallons. An example run of the program is shown below:
Hints:
1. What will your function do? Document that in the comment.
2. What are the parameter(s) to the function? What type? What type is the returned value?
3. What kind of a loop should you use? What’s the minimum number of times the user will go through the
loop?
How to write a function
1) Determine the type and number of parameters
2) Determine the type of the return value
3) Declare the function (usually at the top of the program)
4) Write the function (usually at the bottom of the program)
![[mingli@polaris:~/TA]$ ./lab4_function
Please enter the number of liters and the number of miles:
10 50
Miles per gallon is : 18.9266
Continue (Y/N) ?
Y
Please enter the number of liters and the number of miles:
5.8 43
Miles per gallon is : 28.0635
Continue (Y/N) ?
Y
Please enter the number of liters and the number of miles:
3.2 36.7
Miles per gallon is : 43.4128
Continue (Y/N) ?
N
[mingli@polaris:~/TA]$ O](https://content.bartleby.com/qna-images/question/5bd3697d-7244-4dc8-845a-c06954fa71b0/f93faddb-f64b-4c0f-b97e-e385a5a9714c/85z8faj_thumbnail.png)

Modified Program:
#include <iostream>
using namespace std;
int main(void)
{
const double GALLONS_PER_LITER = 0.264179;
int liters = 0;
double distance = 0.0;
double mpg = 0.0;
do
{
cout << "Please input how many liters of gasoline is in your vehicle: ";
cin >> liters;
cout << "Please input the distance in miles you traveled in your vehicle: ";
cin >> distance;
mpg = distance / (liters * GALLONS_PER_LITER);
cout << "Your vehicle's MPG is: " << mpg << endl;
}while(liters >-1);
return 0;
}
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- One lap around a standard high-school running track is exactly 0.25 miles. Define a function named LapsToMiles that takes a double as a parameter, representing the number of laps, and returns a double that represents the number of miles. Then, write a main program that takes a number of laps as an input, calls function LapsToMiles() to calculate the number of miles, and outputs the number of miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: printf("%0.21f\n", yourValue); Ex: If the input is: 7.6 the output is: 1.90 Ex: If the input is: 2.2 the output is: 0.55 The program must define and call a function: double LapsToMiles (double userLaps) 412800.2778464.qx3zqy7 LAB ACTIVITY 1 #include 2 3 /* Define your function here */ 4 6.20.1: LAB: Track laps to miles 8 5 int main(void) { 6 7 /* Type your code here. Your code must call the function. */ 9 10 11 main.c return 0; 0/10 Load default template...arrow_forwardOne lap around a standard high-school running track is exactly 0.25 miles. Define a function named laps_to_miles that takes a number of laps as a parameter, and returns the number of miles. Then, write a main program that takes a number of laps as an input, calls function laps_to_miles() to calculate the number of miles, and outputs the number of miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print (f'{your_value:.2f}') Ex: If the input is: 7.6 the output is: 1.90 Ex: If the input is: 2.2 the output is: 0.55 The program must define and call the following function: def laps_to_miles (user_laps) 461710.3116374.qx3zqy7 LAB ACTIVITY 7.8.1: LAB: Track laps to miles 1 # Define your function here 2 3 if __name__ 4 main.py == '__main__': #Type your code here. Your code must call the function. 0/10 Load default template...arrow_forwardDo not use global variables for this assignment Choose descriptive variable names in all programs. Currency format. There should be no space between the $ sign and the first digit. In python, Write a program that uses a custom function named as you wish and the main function. In main, prompt the user for a first name and an integer less than 10 and then execute the custom function with these two inputs as arguments. The custom function should output one line displaying the name as many times as specified by the integer, separating each repetition of the name with a space.arrow_forward
- The prompt asks: Write a function that calculates the amount of money a person would earn over a period of years if his or her salary is one penny the first day, two pennies the second day, and continues to double each day. The program should ask the user for the number of years and call the function which will return the total money earned in dollars and cents, not pennies. Assume there are 365 days in a year. function totalEarned(years) { } var yearsWorked = parseInt(prompt('How many years will you work for pennies a day? ')); alert('Over a total of ' + yearsWorked + ', you will have earned $' + totalEarned(yearsWorked));arrow_forwardExercise 4 A number, a, is a power of b if it is divisible by b and a/b is a power of b. Write a function called is_power that takes parameters a and b and returns True if a is a power of b. Note: you will have to think about the base case.arrow_forwardOne lap around a standard high-school running track is exactly 0.25 miles. Define a function named laps_to_miles that takes a number of laps as a parameter, and returns the number of miles. Then, write a main program that takes a number of laps as an input, calls function laps_to_miles() to calculate the number of miles, and outputs the number of miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print (f' {your_value:.2f}') Ex: If the input is: 7.6 the output is: 1.90 Ex: If the input is: 2.2 the output is: 0.55 The program must define and call the following function: def laps_to_miles (user_laps) 461710.3116374.qx3zqy7 LAB ACTIVITY 1 # Define your function here 21 3 00 Jo UAWN P 4 if ___name__ 5 6 7.8.1: LAB: Track laps to miles 7 8 main.py '__main__': # Type your code here. Your code must call the function. 8/10 Load default template...arrow_forward
- in c++ please A liter is 0.264179 gallons. Write a program that will read in the number of liters of gasoline consumed by the user’s car and the number of miles traveled by the car and will then output the number of miles per gallon the car delivered. Your program should allow the user to repeat this calculation as often as the user wishes. Define a function to compute the number of miles per gallon. Your program should use a globally defined constant for the number of liters per gallon.arrow_forwardDefine a function called exact_change that takes the total change amount in cents and calculates the change using the fewest coins. The coin types are pennies, nickels, dimes, and quarters. Then write a main program that reads the total change amount as an integer input, calls exact_change(), and outputs the change, one coin type per line. Use singular and plural coin names as appropriate, like 1 penny vs. 2 pennies. Output "no change" if the input is 0 or less. Ex: If the input is: 0 (or less), the output is: no change Ex: If the input is: 45 the output is: 2 dimes 1 quarter Your program must define and call the following function. The function exact_change() should return num_pennies, num_nickels, num_dimes, and num_quarters. def exact_change (user_total)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
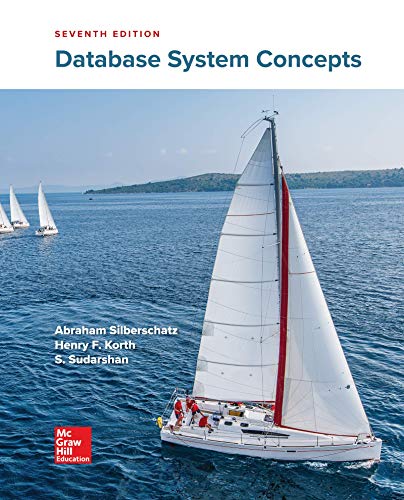
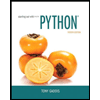
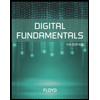
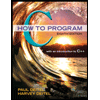
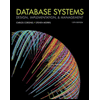
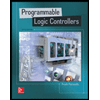