One lap around a standard high-school running track is exactly 0.25 miles. Define a function named LapsToMiles that takes a double as a parameter, representing the number of laps, and returns a double that represents the number of miles. Then, write a main program that takes a number of laps as an input, calls function LapsToMiles() to calculate the number of miles, and outputs the number of miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: printf("%0.21f\n", yourValue); Ex: If the input is: 7.6 the output is: 1.90 Ex: If the input is: 2.2 the output is: 0.55
One lap around a standard high-school running track is exactly 0.25 miles. Define a function named LapsToMiles that takes a double as a parameter, representing the number of laps, and returns a double that represents the number of miles. Then, write a main program that takes a number of laps as an input, calls function LapsToMiles() to calculate the number of miles, and outputs the number of miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: printf("%0.21f\n", yourValue); Ex: If the input is: 7.6 the output is: 1.90 Ex: If the input is: 2.2 the output is: 0.55
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:One lap around a standard high-school running track is exactly 0.25 miles. Define a function named LapsToMiles that takes a double as a
parameter, representing the number of laps, and returns a double that represents the number of miles. Then, write a main program that
takes a number of laps as an input, calls function LapsToMiles() to calculate the number of miles, and outputs the number of miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
printf("%0.21f\n", yourValue);
Ex: If the input is:
7.6
the output is:
1.90
Ex: If the input is:
2.2
the output is:
0.55
The program must define and call a function:
double LapsToMiles (double userLaps)
412800.2778464.qx3zqy7
LAB
ACTIVITY
1 #include <stdio.h>
2
3 /* Define your function here */
4
6.20.1: LAB: Track laps to miles
8
5 int main(void) {
6
7
/* Type your code here. Your code must call the function. */
9
10
11
main.c
return 0;
0/10
Load default template...
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
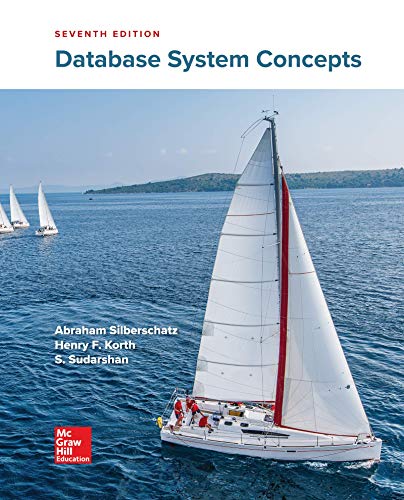
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
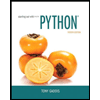
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
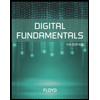
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
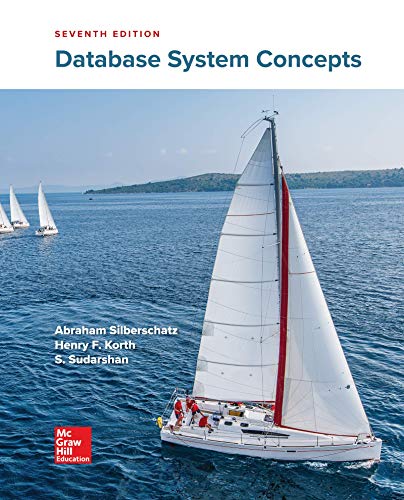
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
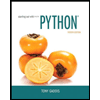
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
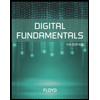
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
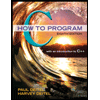
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
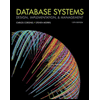
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
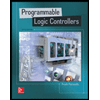
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education