Write a program that uses a structure to store the information about the Items in a retail store. It has Items with name, manufacturer (Final) and price. 1. Provide default & Parameterized constructors. 2. Provide getters & setters for data members. 3. Provide A toString() method to print values. We can add several items to Store. Now create another class store which has name, city and array of Items. 1. Provide default & Parameterized constructors. 2. Provide getters & setters for data members. 3. Provide necessary validation checks ( null pointer exception, index out of bounds,etc). where possible. 4. Provide a method AddItem (Item i) which is used to add items into the store, but number of items should be less than the size of array (check for exception). 5. A print function which shows all items in the store. 6. Create Test Application and test the functionality of above classes. Use exception also Programming language : Java
Write a program that uses a structure to store the information about the Items in a retail store. It has Items with name, manufacturer (Final) and price.
1. Provide default & Parameterized constructors.
2. Provide getters & setters for data members.
3. Provide A toString() method to print values.
We can add several items to Store. Now create another class store which has name, city and array of Items.
1. Provide default & Parameterized constructors.
2. Provide getters & setters for data members.
3. Provide necessary validation checks ( null pointer exception, index out of bounds,etc). where possible.
4. Provide a method AddItem (Item i) which is used to add items into the store, but number of items should be less than the size of array (check for exception).
5. A print function which shows all items in the store.
6. Create Test Application and test the functionality of above classes.
Use exception also

Step by step
Solved in 2 steps with 1 images

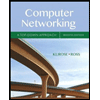
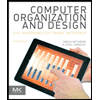
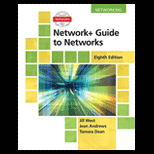
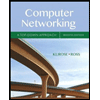
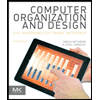
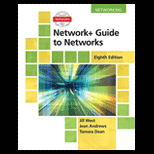
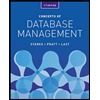
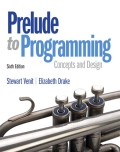
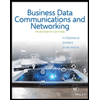