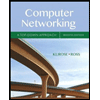
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the
As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2
Note: The above algorithm outputs the 0's and 1's in reverse order.
Ex: If the input is 6, the output is:
011
(6 in binary is 110; the algorithm outputs the bits in reverse).
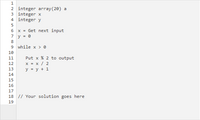
Transcribed Image Text:```plaintext
1 integer array(20) a
2 integer x
3 integer y
4
5 x = Get next input
6 y = 0
7
8 while x > 0
9
10 Put x % 2 to output
11 x = x / 2
12 y = y + 1
13
14
15
16 // Your solution goes here
```
### Explanation
This code snippet appears to be the beginning of a program that processes input integers. Here is a breakdown of each part:
1. **Declarations:**
- `integer array(20) a`: Declares an array `a` with space for 20 integers.
- `integer x`: Declares an integer variable `x`.
- `integer y`: Declares an integer variable `y`.
2. **Initialization:**
- `x = Get next input`: Assigns the next input value to `x`.
- `y = 0`: Initializes `y` to 0.
3. **While Loop (`while x > 0`)**:
- This loop continues as long as `x` is greater than 0.
- Within the loop:
- `Put x % 2 to output`: Outputs the remainder of `x` divided by 2 (i.e., outputs the least significant bit of `x`).
- `x = x / 2`: Updates `x` by performing integer division by 2, effectively right-shifting its binary representation.
- `y = y + 1`: Increments `y` by 1.
4. **Comment Placeholder:**
- `// Your solution goes here`: A placeholder comment indicating where additional solution logic should be implemented.
### Analysis
This appears to be a snippet often used to convert an integer into its binary representation, outputting each bit. The loop divides the number by 2 each time, outputting the least significant bit until the number is reduced to 0.
No graphs or diagrams are included in this image; it is purely a text representation of an algorithm.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Java - Convert to Reverse Binaryarrow_forwardWrite a program using integers userNum and x as input, and output userNum divided by x four times. Ex: If the input is: 2000 2 the output is: 1000 500 250 125 Note: In Coral, integer division discards fractions. Ex: 6 / 4 is 1 (the 0.5 is discarded). 333666.1636490.qx3zqy7arrow_forwardWrite a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 Your program must define and call the following two functions. The IntegerToReverseBinary() function should return a string of 1's and 0's representing the integer in binary (in reverse). The ReverseString() function should return a string representing the input string in reverse. string IntegerToReverseBinary(int integerValue)string ReverseString(string userString) #include <iostream>using namespace std; /* Define your functions here */ int main() {/* Type your code here. Your code must call the functions. */ return 0;} Please help me with this problem using…arrow_forward
- Let f(x)= 1 if x is even; f(x)= 0 if x is odd. Write a program in ý that computes f.arrow_forwardGiven a positive integer 'n', find and return the minimum number of steps that 'n' has to take to get reduced to 1. You can perform any one of the following 3 steps:1.) Subtract 1 from it. (n = n - 1) ,2.) If its divisible by 2, divide by 2.( if n % 2 == 0, then n = n / 2 ) ,3.) If its divisible by 3, divide by 3. (if n % 3 == 0, then n = n / 3 ). Write brute-force recursive solution for this.Input format :The first and the only line of input contains an integer value, 'n'.Output format :Print the minimum number of steps.Constraints :1 <= n <= 200 Time Limit: 1 secSample Input 1 :4Sample Output 1 :2 Explanation of Sample Output 1 :For n = 4Step 1 : n = 4 / 2 = 2Step 2 : n = 2 / 2 = 1 Sample Input 2 :7Sample Output 2 :3Explanation of Sample Output 2 :For n = 7Step 1 : n = 7 - 1 = 6Step 2 : n = 6 / 3 = 2 Step 3 : n = 2 / 2 = 1 SolutionDp///.arrow_forwardLet P(x, y) is "x + 2y = xy," where x and y are integers. Given: (1) ForEvery x ThereExists y P(x, y). (2) ThereExists y ForEvery x P(x, y). Select one of the following choices: a. (1) and (2) are True. ○ b. (1) and (2) are False. c. (1) is False and (2) is True. ○ d. (1) is True and (2) is False.arrow_forward
- Justin receives 52 weekly paychecks per year. Each week, he contributes a specific percentage of his gross weekly pay to his retirement plan at work. His employer also contributes to his retirement plan, but at a different rate. Justin wants a program that calculates and displays the total annual contribution made to his retirement plan by him and his employer. Desk-check your solution’s algorithm using $640 as the gross weekly pay, 6% as Justin’s contribution rate, and 4% as the employer’s contribution rate. Then desk-check it using your own set of data.arrow_forwardGiven a positive integer 'n', find and return the minimum number of steps that 'n' has to take to get reduced to 1. You can perform any one of the following 3 steps:1.) Subtract 1 from it. (n = n - 1) ,2.) If its divisible by 2, divide by 2.( if n % 2 == 0, then n = n / 2 ) ,3.) If its divisible by 3, divide by 3. (if n % 3 == 0, then n = n / 3 ). Write brute-force recursive solution for this.Input format :The first and the only line of input contains an integer value, 'n'.Output format :Print the minimum number of steps.Constraints :1 <= n <= 200 Time Limit: 1 secSample Input 1 :4Sample Output 1 :2 Explanation of Sample Output 1 :For n = 4Step 1 : n = 4 / 2 = 2Step 2 : n = 2 / 2 = 1 Sample Input 2 :7Sample Output 2 :3Explanation of Sample Output 2 :For n = 7Step 1 : n = 7 - 1 = 6Step 2 : n = 6 / 3 = 2 Step 3 : n = 2 / 2 = 1 SolutionDp///.arrow_forwardWrite a program that takes in a line of text as input, and outputs that line of text in reverse. The program repeats, ending when the user enters "Done", "done", or "d" for the line of text. Ex: If the input is: Hello there Hey done then the output is: ereht olleH yeH 461710 3116371arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
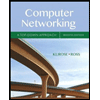
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
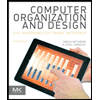
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
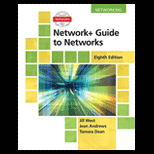
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
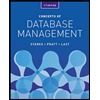
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
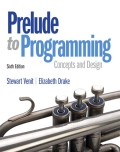
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
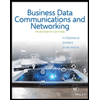
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY